Demystifying the interceptor mechanism in Golang
Interceptor is a design pattern that allows custom behavior to be inserted before and after method execution. In Go, it can be implemented through net/http middleware. It has the advantages of scalability, reusability, testability, etc., and can be used in scenarios such as authentication, authorization, caching, logging, and custom error handling.
Demystifying the interceptor mechanism in Golang
Introduction
Interceptor is a design pattern. Allows custom behavior to be inserted before or after method execution. In Go, interceptors can be implemented by writing net/http
middleware.
Specific implementation
The following is an example of writing an interceptor using net/http
:
package main import ( "fmt" "log" "net/http" ) func main() { // 创建一个新的中间件函数 logMiddleware := func(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { log.Println("Request received:", r.URL.Path) // 调用下一个处理程序 next.ServeHTTP(w, r) }) } // 创建一个新的 HTTP 处理程序 mux := http.NewServeMux() mux.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "Hello, World!") }) // 将中间件应用到处理程序 http.Handle("/", logMiddleware(mux)) // 启动 HTTP 服务器 log.Println("Listening on port 8080") http.ListenAndServe(":8080", nil) }
Principle
This interceptor uses net/http.HandlerFunc
to create a new HTTP handler. This handler is executed before and after the request reaches the original handler. In our example, logMiddleware
logs the received request before the handler receives the request and calls the next handler.
Practical cases
Interceptors can be used in various scenarios, including:
- Authentication and authorization
- Cache
- Logging and Monitoring
- Custom Error Handling
Advantages
- Scalability: Interception The tool allows you to easily add new functionality to your application without modifying existing code.
- Reusability: Interceptors can be reused across multiple endpoints or handlers.
- Testability: Interceptors are modular components that are easy to test and maintain.
The above is the detailed content of Demystifying the interceptor mechanism in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


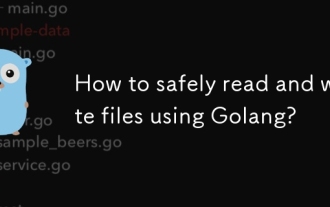
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
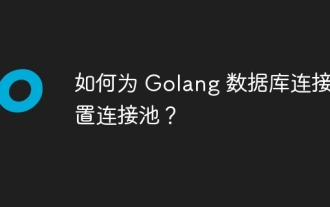
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
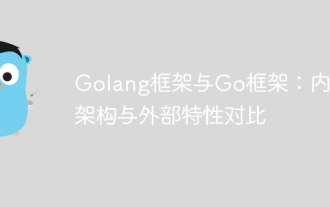
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
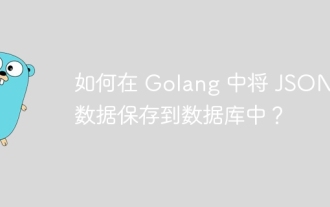
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
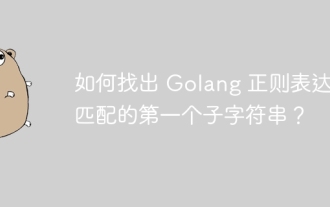
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
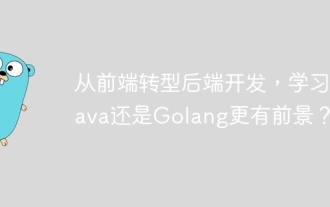
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
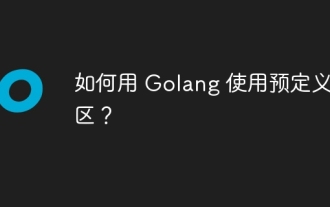
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
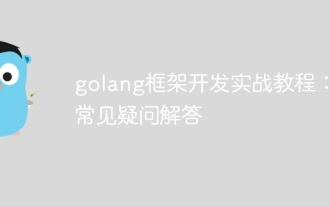
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
