The essence of programming absorbed in Go language
Go 语言融合了并发性、类型系统、内置函数和标准库的精粹,使其成为现代编程语言。并发模型基于 CSP,允许 goroutine 并行执行,通过管道和通道通信。类型系统强调结构体和接口,提供清晰的数据组织。内置函数操作字符串和数据类型,简洁且易读。标准库包含用于各种任务的包,例如网络和文件处理。
Go 语言中的编程精粹
Go 语言是一种现代编程语言,以其简洁性、并发性和高性能而闻名。它吸收了来自其他编程语言的众多精粹,使其成为从事各种项目的有力选择。
并发编程
Go 语言的并发模型基于CSP(通信顺序进程)范式。它允许并发地执行多个 goroutine,这是 Go 中轻量级的线程。通过使用管道和通道,goroutine 可以安全有效地通信。
实战案例: 并发 Web 服务器
package main import ( "fmt" "log" "net/http" "sync" ) func main() { // 创建一个等待组来跟踪并发请求 wg := &sync.WaitGroup{} // 启动一个 HTTP 服务器 http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { // 增加等待组的计数,表示有一个并发请求 wg.Add(1) // 处理请求 fmt.Fprintf(w, "Hello, World!") // 减少等待组的计数,表示请求已完成 wg.Done() }) http.ListenAndServe(":8080", nil) // 等待所有并发请求完成 wg.Wait() }
类型系统
Go 语言具有简单的类型系统,强调结构体和接口。结构体是用于分组相关数据的值类型,而接口则定义契约,指定类型必须实现的一组方法。
实战案例: 自定义数据结构和接口
package main import "fmt" // 学生结构体 type Student struct { Name string Age int } // 获取学生姓名的方法 func (s Student) GetName() string { return s.Name } func main() { // 创建一个学生结构体 student := Student{ Name: "Alice", Age: 20, } // 使用学生结构体实现 GetName 方法 fmt.Println("Student name:", student.GetName()) }
内置函数
Go 语言提供了一组丰富的内置函数,可用于操纵字符串、数字和其他数据类型。这些函数简洁且易于使用,提供了强大且可读性强的代码。
实战案例: 使用内置函数处理字符串
package main import "strings" import "fmt" func main() { // 字符串比较 fmt.Println(strings.EqualFold("str1", "STR1")) // true // 从字符串中获取前缀 fmt.Println(strings.HasPrefix("abcdef", "ab")) // true // 将字符串转换为小写 fmt.Println(strings.ToLower("EXAMPLE")) // example }
标准库
Go 语言随附了标准库,其中包含大量用于各种任务的包。标准库中的包涵盖了网络、文件处理、加密和许多其他领域。
实战案例: 使用标准库进行文件操作
package main import ( "fmt" "io/ioutil" ) func main() { // 读取文件 contents, err := ioutil.ReadFile("file.txt") if err != nil { fmt.Println("Error reading file:", err) } else { fmt.Println(string(contents)) } }
The above is the detailed content of The essence of programming absorbed in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
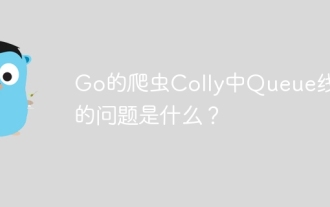
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
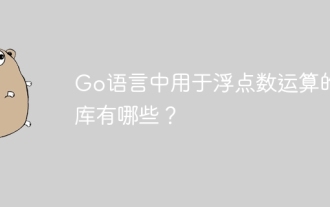
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
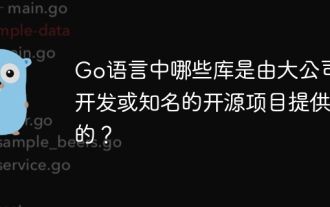
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
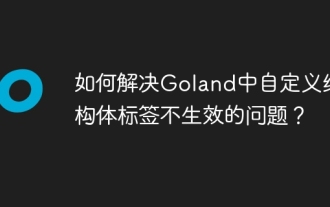
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
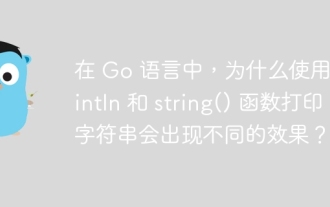
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
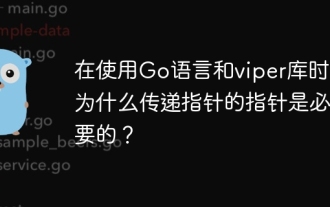
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
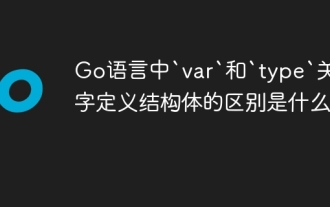
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
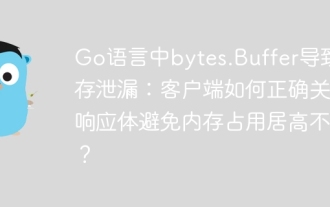
Analysis of memory leaks caused by bytes.makeSlice in Go language In Go language development, if the bytes.Buffer is used to splice strings, if the processing is not done properly...
