Golang + browser: building cross-platform web applications
The Go language can be combined with a browser to build cross-platform web applications. With the help of the Browser.Dial() and Browser.Close() functions, connection and communication with the browser can be achieved. Through WebSockets, Go applications can also communicate bi-directionally with the browser, sending and receiving messages. Practical examples include building a real-time chat application using Go and the browser.
Golang Browser: Building Cross-Platform Web Applications
Introduction
The Go language relies on its Concurrency, high performance, and simplicity make it an ideal choice for building web applications. By integrating with the browser, Go applications can be easily cross-platform, highly interactive, and responsive. This article will introduce how to use the Go language to interact with the browser and provide practical cases to demonstrate its powerful capabilities.
Browser.Dial() and Browser.Close()Browser.Dial()
function is used to make HTTP requests, it returns a BrowserConn
object, which can be used to send and receive HTTP messages. Browser.Close()
The function closes the connection with the browser.
import ( "context" "fmt" "log" "github.com/GoogleCloudPlatform/functions-framework-go/functions" ) func init() { functions.HTTP("HelloWorld", HelloWorld) } // HelloWorld 是一个 HTTP Cloud Function,它向浏览器发送一个包含 "Hello, World!" 的 HTML 响应。 func HelloWorld(w http.ResponseWriter, r *http.Request) { browser, err := Browser.Dial(context.Background()) if err != nil { log.Printf("faile to dial browser: %v", err) return } defer browser.Close() resp, err := browser.Get(context.Background(), "https://example.com") if err != nil { log.Printf("failed to get from: %v", err) return } fmt.Fprintln(w, "<h1>Hello, World!</h1>") }
Two-way communication via WebSocket
Go applications can also achieve two-way communication with the browser via WebSocket.
import ( "context" "fmt" "github.com/Goddard4387/browser" ) func main() { browser, err := Browser.Dial(context.Background()) if err != nil { log.Fatalf("failed to dial browser: %v", err) } defer browser.Close() conn, err := browser.ConnectWS(context.Background(), "ws://example.com/ws") if err != nil { log.Fatalf("failed to connect WS: %v", err) } defer conn.Close() // 发送消息 if err = conn.Write(context.Background(), []byte("Hello from Go")); err != nil { log.Printf("failed to write to WS: %v", err) return } // 接收消息 for { msg, err := conn.Read(context.Background()) if err == ErrClosed { fmt.Println("connection closed") break } if err != nil { log.Printf("failed to read from WS: %v", err) return } fmt.Println("received message:", string(msg)) } }
Practical Case
A practical case built using Go language and browser is a real-time chat application. The application can broadcast messages via WebSocket to all browsers connected to the server.
Conclusion
The combination of the Go language and the browser provides powerful tools for building cross-platform, highly interactive and responsive web applications. By using functions such as Browser.Dial()
and Browser.Close()
, as well as WebSocket functionality, Go applications can easily communicate with the browser and create complex interactive applications .
The above is the detailed content of Golang + browser: building cross-platform web applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


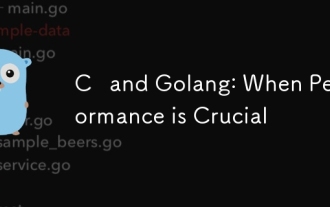
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
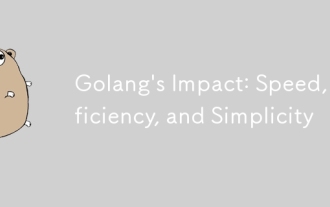
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
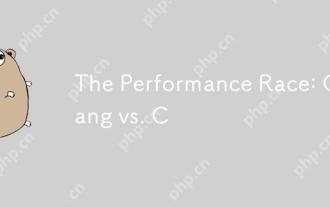
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
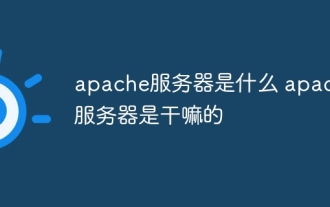
Apache server is a powerful web server software that acts as a bridge between browsers and website servers. 1. It handles HTTP requests and returns web page content based on requests; 2. Modular design allows extended functions, such as support for SSL encryption and dynamic web pages; 3. Configuration files (such as virtual host configurations) need to be carefully set to avoid security vulnerabilities, and optimize performance parameters, such as thread count and timeout time, in order to build high-performance and secure web applications.
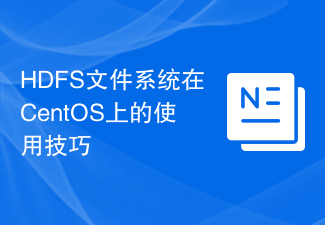
The Installation, Configuration and Optimization Guide for HDFS File System under CentOS System This article will guide you how to install, configure and optimize Hadoop Distributed File System (HDFS) on CentOS System. HDFS installation and configuration Java environment installation: First, make sure that the appropriate Java environment is installed. Edit /etc/profile file, add the following, and replace /usr/lib/java-1.8.0/jdk1.8.0_144 with your actual Java installation path: exportJAVA_HOME=/usr/lib/java-1.8.0/jdk1.8.0_144exportPATH=$J
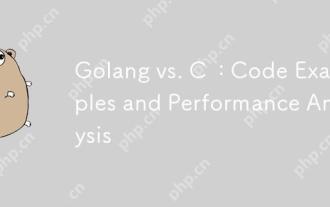
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
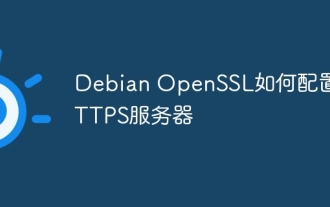
Configuring an HTTPS server on a Debian system involves several steps, including installing the necessary software, generating an SSL certificate, and configuring a web server (such as Apache or Nginx) to use an SSL certificate. Here is a basic guide, assuming you are using an ApacheWeb server. 1. Install the necessary software First, make sure your system is up to date and install Apache and OpenSSL: sudoaptupdatesudoaptupgradesudoaptinsta
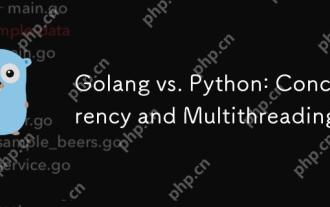
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
