


Go language concurrent data structure: performance optimization of queues and stacks
In the Go language, the performance of queues and stacks can be achieved through the following optimizations: using sync.Mutex and sync.Cond to implement concurrent queues to ensure the safety of read and write operations. Use sync.Mutex and atomic packages to implement concurrent stacks to ensure atomicity of top pointer updates. In practical cases, efficient concurrent processing is achieved through concurrent queue and stack processing tasks.
Go language concurrent data structure: performance optimization of queues and stacks
In Go, queues and stacks are commonly used data structure. However, in high-concurrency scenarios, the default implementation may not meet performance requirements. This article will introduce how to use the built-in concurrency primitives of the Go language to optimize the performance of queues and stacks.
Optimizing Queue
Go provides sync.Mutex
and sync.Cond
primitives to implement concurrent queues. Here is a concurrent queue implemented using sync.Mutex
and sync.Cond
:
type ConcurrentQueue struct { m sync.Mutex items []interface{} conds sync.Cond } func (q *ConcurrentQueue) Enqueue(v interface{}) { q.m.Lock() defer q.m.Unlock() q.items = append(q.items, v) q.conds.Signal() } func (q *ConcurrentQueue) Dequeue() interface{} { q.m.Lock() defer q.m.Unlock() var v interface{} if len(q.items) > 0 { v = q.items[0] q.items = q.items[1:] } return v }
By using sync.Mutex
and sync.Cond
, we can safely read and write queues in concurrent scenarios. Use the Signal
signal to wake up the waiting coroutine, thereby improving efficiency.
Optimized Stack
There is no built-in concurrent stack implementation in Go. Here is a concurrency stack implemented using the sync.Mutex
and atomic
packages:
type ConcurrentStack struct { m sync.Mutex top *node } type node struct { data interface{} next *node } func (s *ConcurrentStack) Push(v interface{}) { s.m.Lock() defer s.m.Unlock() n := &node{data: v} n.next = s.top s.top = n } func (s *ConcurrentStack) Pop() interface{} { s.m.Lock() defer s.m.Unlock() if s.top == nil { return nil } v := s.top.data s.top = s.top.next return v }
Using variables in the atomic
package can ensure a concurrent environment The top
pointer updates below are atomic.
Practical case
The following is an example of using concurrent queues and stacks to handle concurrent tasks:
func main() { q := ConcurrentQueue{} s := ConcurrentStack{} for i := 0; i < 1000; i++ { // 向队列中并发添加任务 go func(i int) { q.Enqueue(i) }(i) } for i := 0; i < 1000; i++ { // 从队列中并发获取任务并推入栈中 go func() { if v := q.Dequeue(); v != nil { s.Push(v) } }() } for i := 0; i < 1000; i++ { // 从栈中弹出任务并处理 go func() { if v := s.Pop(); v != nil { // 处理任务 v } }() } }
This example adds 1000 tasks concurrently to queue, and concurrently obtain tasks from the queue and push them onto the stack. Tasks are then concurrently popped from the stack and processed. By using concurrent data structures, this example can efficiently handle large concurrency tasks.
The above is the detailed content of Go language concurrent data structure: performance optimization of queues and stacks. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
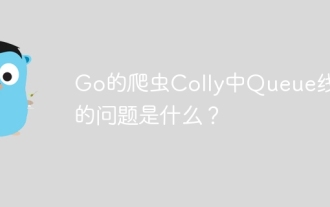
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
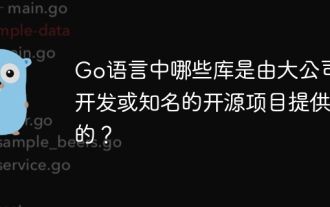
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
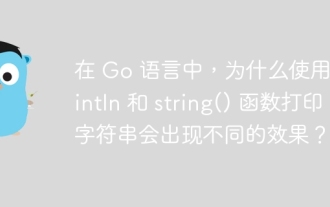
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
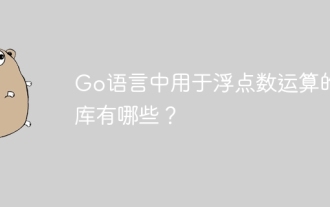
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
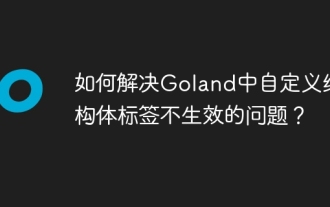
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
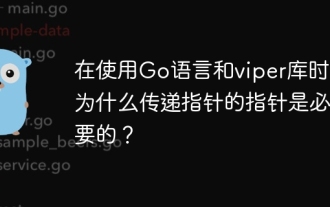
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
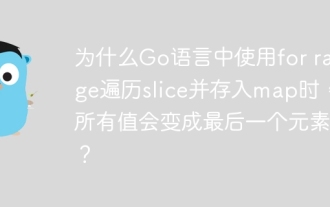
Why does map iteration in Go cause all values to become the last element? In Go language, when faced with some interview questions, you often encounter maps...
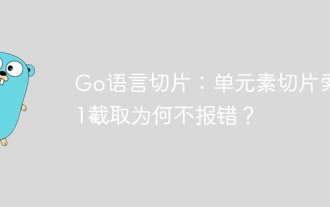
Go language slice index: Why does a single-element slice intercept from index 1 without an error? In Go language, slices are a flexible data structure that can refer to the bottom...
