Getting Started with Go Language: Analysis of Essential Features
Go language is a modern programming language known for its speed, efficiency and ease of use. Its essential features include: Variables and Constants: Variables can be reassigned, while constants cannot be modified once declared. Data types: Provides rich built-in data types, including numerical values, strings, sets, etc. Control flow: supports if-else, for loops, and switch-case statements. Function: supports function declaration and calling. Concurrency and Goroutine: Provides Goroutine (lightweight thread) to support concurrent programming.
Getting Started with Go Language: Analysis of Essential Features
Go language, also known as Golang, is a modern language developed by Google. programming language. It is known for its speed, efficiency and ease of use. This guide will introduce the essential features of the Go language and explain them through practical cases.
1. Variables and constants
// 变量声明 var name string = "John Doe" // 常量声明 const age = 30
Variables can be reassigned, but constants cannot be modified once declared.
2. Data types
The Go language provides a wealth of built-in data types:
- Numeric types:
int
,float64
,bool
- String type:
string
- Collection type:
slice
,map
,struct
3. Control flow
if-else Statement:
if age >= 18 { fmt.Println("成年人") } else { fmt.Println("未成年人") }
Copy after loginfor Loop:
for i := 0; i < 10; i++ { fmt.Println(i) }
Copy after loginswitch-case Statement:
switch age { case 18: fmt.Println("刚成年") case 30: fmt.Println("三十而立") default: fmt.Println("其他年龄") }
Copy after login
4. Function
Function declaration:
func greet(name string) { fmt.Println("Hello", name) }
Copy after loginFunction call:
greet("John Doe")
Copy after login
5. Concurrency and Goroutine
Go language supports concurrent programming, goroutine
It is a lightweight thread in Go.
Create Goroutine:
go greet("John Doe")
Copy after loginWaiting for Goroutine:
var wg sync.WaitGroup wg.Add(1) go func() { defer wg.Done() greet("John Doe") }() wg.Wait()
Copy after login
Actual case: Calculating prime numbers
package main import "fmt" // 判断是否为质数 func isPrime(num int) bool { for i := 2; i <= num/2; i++ { if num%i == 0 { return false } } return true } func main() { fmt.Println("计算 100 以内的质数:") for i := 1; i <= 100; i++ { if isPrime(i) { fmt.Printf("%d ", i) } } fmt.Println() }
Through this guide, you have learned about the basic features of the Go language, including variables, data types, control flow, functions, and concurrency. Mastering these features will lay a solid foundation for your in-depth learning of the Go language.
The above is the detailed content of Getting Started with Go Language: Analysis of Essential Features. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

Kimi: In just one sentence, in just ten seconds, a PPT will be ready. PPT is so annoying! To hold a meeting, you need to have a PPT; to write a weekly report, you need to have a PPT; to make an investment, you need to show a PPT; even when you accuse someone of cheating, you have to send a PPT. College is more like studying a PPT major. You watch PPT in class and do PPT after class. Perhaps, when Dennis Austin invented PPT 37 years ago, he did not expect that one day PPT would become so widespread. Talking about our hard experience of making PPT brings tears to our eyes. "It took three months to make a PPT of more than 20 pages, and I revised it dozens of times. I felt like vomiting when I saw the PPT." "At my peak, I did five PPTs a day, and even my breathing was PPT." If you have an impromptu meeting, you should do it
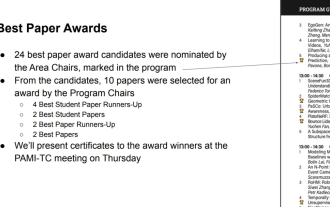
In the early morning of June 20th, Beijing time, CVPR2024, the top international computer vision conference held in Seattle, officially announced the best paper and other awards. This year, a total of 10 papers won awards, including 2 best papers and 2 best student papers. In addition, there were 2 best paper nominations and 4 best student paper nominations. The top conference in the field of computer vision (CV) is CVPR, which attracts a large number of research institutions and universities every year. According to statistics, a total of 11,532 papers were submitted this year, and 2,719 were accepted, with an acceptance rate of 23.6%. According to Georgia Institute of Technology’s statistical analysis of CVPR2024 data, from the perspective of research topics, the largest number of papers is image and video synthesis and generation (Imageandvideosyn
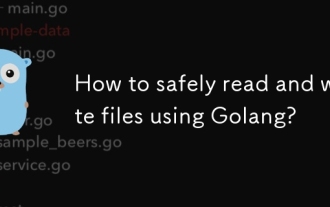
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
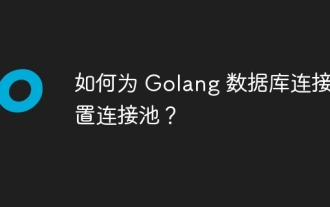
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
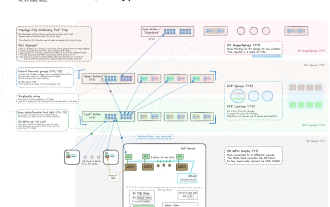
We know that LLM is trained on large-scale computer clusters using massive data. This site has introduced many methods and technologies used to assist and improve the LLM training process. Today, what we want to share is an article that goes deep into the underlying technology and introduces how to turn a bunch of "bare metals" without even an operating system into a computer cluster for training LLM. This article comes from Imbue, an AI startup that strives to achieve general intelligence by understanding how machines think. Of course, turning a bunch of "bare metal" without an operating system into a computer cluster for training LLM is not an easy process, full of exploration and trial and error, but Imbue finally successfully trained an LLM with 70 billion parameters. and in the process accumulate
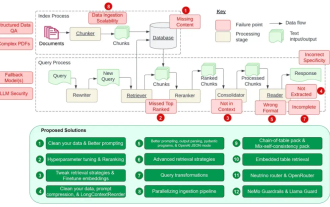
Retrieval-augmented generation (RAG) is a technique that uses retrieval to boost language models. Specifically, before a language model generates an answer, it retrieves relevant information from an extensive document database and then uses this information to guide the generation process. This technology can greatly improve the accuracy and relevance of content, effectively alleviate the problem of hallucinations, increase the speed of knowledge update, and enhance the traceability of content generation. RAG is undoubtedly one of the most exciting areas of artificial intelligence research. For more details about RAG, please refer to the column article on this site "What are the new developments in RAG, which specializes in making up for the shortcomings of large models?" This review explains it clearly." But RAG is not perfect, and users often encounter some "pain points" when using it. Recently, NVIDIA’s advanced generative AI solution
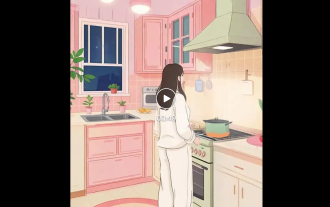
Editor of the Machine Power Report: Yang Wen The wave of artificial intelligence represented by large models and AIGC has been quietly changing the way we live and work, but most people still don’t know how to use it. Therefore, we have launched the "AI in Use" column to introduce in detail how to use AI through intuitive, interesting and concise artificial intelligence use cases and stimulate everyone's thinking. We also welcome readers to submit innovative, hands-on use cases. Video link: https://mp.weixin.qq.com/s/2hX_i7li3RqdE4u016yGhQ Recently, the life vlog of a girl living alone became popular on Xiaohongshu. An illustration-style animation, coupled with a few healing words, can be easily picked up in just a few days.
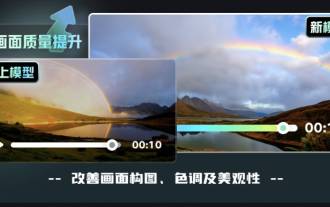
On July 24, Kuaishou video generation large model Keling AI announced that the basic model has been upgraded again and is fully open for internal testing. Kuaishou said that in order to allow more users to use Keling AI and better meet the different levels of usage needs of creators, from now on, on the basis of fully open internal testing, it will also officially launch a membership system for different categories of members. Provide corresponding exclusive functional services. At the same time, the basic model of Keling AI has also been upgraded again to further enhance the user experience. The basic model effect has been upgraded to further improve the user experience. Since its release more than a month ago, Keling AI has been upgraded and iterated many times. With the launch of this membership system, the basic model effect of Keling AI has once again undergone transformation. The first is that the picture quality has been significantly improved. The visual quality generated through the upgraded basic model
