Go memory leak tracking: Go pprof practical guide
pprof tool can be used to analyze the memory usage of Go applications and detect memory leaks. It provides memory profile generation, memory leak identification and real-time analysis capabilities. Generate a memory snapshot by using pprof.Parse and identify the data structures with the most memory allocations using the pprof -allocspace command. At the same time, pprof supports real-time analysis and provides endpoints to remotely access memory usage information.
Go pprof: Memory Leak Tracking Guide
Memory leaks are common problems during the development process, and in serious cases may cause the application to Crashes or performance degradation. Go provides a tool called pprof for analyzing and detecting memory leaks.
pprof Tools
pprof is a set of command line tools that can be used to generate memory profiles of applications and analyze and visualize memory usage. pprof provides multiple configurations for customizing memory profiling for different situations.
Installation
To install pprof, run the following command:
go install github.com/google/pprof/cmd/pprof
Usage
To generate For memory profiling, you can use the pprof.Parse
function, which accepts a running application as input and generates a memory snapshot file:
import _ "net/http/pprof" func main() { // ...程序代码... // 生成内存快照 f, err := os.Create("mem.pprof") if err != nil { log.Fatal(err) } _ = pprof.WriteHeapProfile(f) // ...更多程序代码... }
Analyze memory leaks
The generated memory snapshot file can be analyzed using the pprof -allocspace
command. This command identifies the memory allocated to different data structures and sorts them by allocation size.
For example, to see which data structures take up the most memory, you can use the following command:
pprof -allocspace -top mem.pprof
Real-time analysis
pprof also supports real-time analysis , which allows you to track your application's memory usage and receive notifications when leaks occur. To enable real-time analysis, import the net/http/pprof
package into your application:
import _ "net/http/pprof"
This will start an HTTP server that provides various endpoints to analyze memory usage . It can be accessed by accessing the endpoint at http://localhost:6060/debug/pprof/
.
Practical Case
Suppose we have a cache
structure in a Go application that uses mapping to store key-value pairs:
type Cache struct { data map[string]interface{} }
We may find a memory leak in the cache
structure because the map key retains a reference to the value even if we no longer need the value.
To solve this problem, we can use so-called "weak references", which allow the reference to a value to be automatically released when the value is not used by the garbage collector.
import "sync/atomic" // 使用原子 int 来跟踪值的引用次数 type WeakRef struct { refCount int32 } type Cache struct { data map[string]*WeakRef } func (c *Cache) Get(key string) interface{} { ref := c.data[key] if ref == nil { return nil } // 增添对弱引用值的引用次数 atomic.AddInt32(&ref.refCount, 1) return ref.v } func (c *Cache) Set(key string, value interface{}) { ref := new(WeakRef) // 将值包装在弱引用中 c.data[key] = ref ref.v = value // 标记对弱引用值的引用 atomic.StoreInt32(&ref.refCount, 1) }
In the above code, we use an atomic int to track the number of references to a weak reference value. When the value is no longer needed, the reference count is reduced to 0 and the weak reference is garbage collected. This may resolve a memory leak in the cache
structure.
The above is the detailed content of Go memory leak tracking: Go pprof practical guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


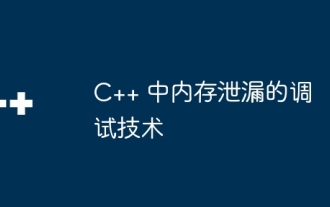
A memory leak in C++ means that the program allocates memory but forgets to release it, causing the memory to not be reused. Debugging techniques include using debuggers (such as Valgrind, GDB), inserting assertions, and using memory leak detector libraries (such as Boost.LeakDetector, MemorySanitizer). It demonstrates the use of Valgrind to detect memory leaks through practical cases, and proposes best practices to avoid memory leaks, including: always releasing allocated memory, using smart pointers, using memory management libraries, and performing regular memory checks.
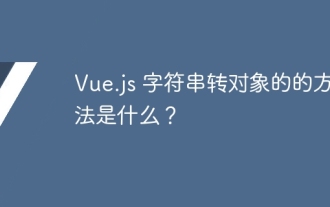
Using JSON.parse() string to object is the safest and most efficient: make sure that strings comply with JSON specifications and avoid common errors. Use try...catch to handle exceptions to improve code robustness. Avoid using the eval() method, which has security risks. For huge JSON strings, chunked parsing or asynchronous parsing can be considered for optimizing performance.
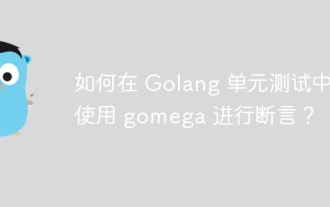
How to use Gomega for assertions in Golang unit testing In Golang unit testing, Gomega is a popular and powerful assertion library that provides rich assertion methods so that developers can easily verify test results. Install Gomegagoget-ugithub.com/onsi/gomega Using Gomega for assertions Here are some common examples of using Gomega for assertions: 1. Equality assertion import "github.com/onsi/gomega" funcTest_MyFunction(t*testing.T){

Recently, "Black Myth: Wukong" has attracted huge attention around the world. The number of people online at the same time on each platform has reached a new high. This game has achieved great commercial success on multiple platforms. The Xbox version of "Black Myth: Wukong" has been postponed. Although "Black Myth: Wukong" has been released on PC and PS5 platforms, there has been no definite news about its Xbox version. It is understood that the official has confirmed that "Black Myth: Wukong" will be launched on the Xbox platform. However, the specific launch date has not yet been announced. It was recently reported that the Xbox version's delay was due to technical issues. According to a relevant blogger, he learned from communications with developers and "Xbox insiders" during Gamescom that the Xbox version of "Black Myth: Wukong" exists.
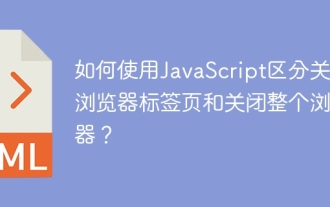
How to distinguish between closing tabs and closing entire browser using JavaScript on your browser? During the daily use of the browser, users may...
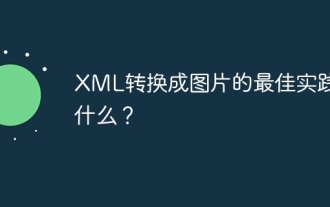
Converting XML into images can be achieved through the following steps: parse XML data and extract visual element information. Select the appropriate graphics library (such as Pillow in Python, JFreeChart in Java) to render the picture. Understand the XML structure and determine how the data is processed. Choose the right tools and methods based on the XML structure and image complexity. Consider using multithreaded or asynchronous programming to optimize performance while maintaining code readability and maintainability.
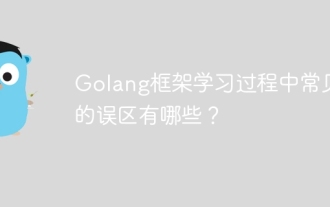
There are five misunderstandings in Go framework learning: over-reliance on the framework and limited flexibility. If you don’t follow the framework conventions, the code will be difficult to maintain. Using outdated libraries can cause security and compatibility issues. Excessive use of packages obfuscates code structure. Ignoring error handling leads to unexpected behavior and crashes.
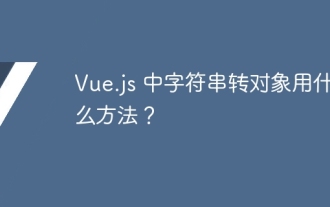
When converting strings to objects in Vue.js, JSON.parse() is preferred for standard JSON strings. For non-standard JSON strings, the string can be processed by using regular expressions and reduce methods according to the format or decoded URL-encoded. Select the appropriate method according to the string format and pay attention to security and encoding issues to avoid bugs.
