


Interpretation of the differences between go language and Java language
The main differences between Go language and Java language are reflected in three aspects: syntax, concurrency model and runtime. Grammatically, Go uses a concise syntax, while Java uses a more verbose syntax. In terms of concurrency model, Go is famous for its goroutine concurrency model, while Java manages concurrency through threads and synchronization primitives. At runtime, Go is compiled into a static binary file, while Java is compiled into intermediate bytecode, which requires JVM execution. The final choice needs to be based on specific project needs. Go is suitable for projects that require low latency and high concurrency, and Java is suitable for projects that require cross-platform portability and a strong library ecosystem.
Interpretation of the differences between Go language and Java language
Go and Java are both popular programming languages, but there are differences in syntax, concurrency model and runtime. Some significant differences. This article will focus on these differences to help you make an informed choice.
Syntax
Go: Go uses a concise syntax with no semicolons or braces. Its developers emphasize code readability and minimize unnecessary syntactic sugar.
Java: Java uses a more verbose syntax, requiring semicolons and explicit braces. This provides greater type safety, but may also result in longer lines of code.
Concurrency Model
Go: Go is famous for its goroutine concurrency model. Goroutines are lightweight user-level threads that can communicate via chan. This provides efficient concurrent execution without the need for locks or other synchronization mechanisms.
Java: Java concurrency is managed through threads and synchronization primitives. Although concurrent programming in Java is relatively mature, the use of locks and atomic operations will increase the complexity of implementing complex concurrent tasks.
Runtime
Go: Go compiles to static binaries that can run on different platforms. Its runtime environment provides garbage collection, concurrency facilities, resource management and other functions.
Java: Java is compiled into intermediate bytecode, which requires a Java Virtual Machine (JVM) to execute. The JVM is responsible for parsing bytecode and managing memory, which provides cross-platform portability but may also increase runtime overhead.
Practical Case
To better understand the difference, let us consider a simple example: parallel calculation of the Fibonacci sequence.
Go:
package main import ( "fmt" "sync" ) var wg sync.WaitGroup func main() { n := 100 res := make([]int, n+1) wg.Add(n) for i := 1; i <= n; i++ { go func(i int) { res[i] = fib(i) wg.Done() }(i) } wg.Wait() fmt.Println(res) } func fib(n int) int { if n <= 1 { return 1 } return fib(n-1) + fib(n-2) }
Java:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class Fibonacci { public static void main(String[] args) { int n = 100; int[] res = new int[n+1]; ExecutorService executorService = Executors.newFixedThreadPool(n); for (int i = 1; i <= n; i++) { executorService.submit(() -> { res[i] = fib(i); }); } executorService.shutdown(); while (!executorService.isTerminated()) { try { Thread.sleep(100); } catch (InterruptedException e) { e.printStackTrace(); } } for (int i : res) { System.out.println(i); } } public static int fib(int n) { if (n <= 1) { return 1; } return fib(n-1) + fib(n-2); } }
Conclusion
Go and Java are powerful Programming languages, each has its own advantages and disadvantages. With its concise syntax, efficient concurrency model and static compilation features, Go is very suitable for projects that require low latency and high concurrency. Java is better suited for projects that require cross-platform portability and a strong library ecosystem. Carefully choosing the right language based on your specific needs is crucial.
The above is the detailed content of Interpretation of the differences between go language and Java language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


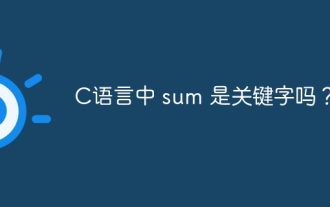
The sum keyword does not exist in C language, it is a normal identifier and can be used as a variable or function name. But to avoid misunderstandings, it is recommended to avoid using it for identifiers of mathematical-related codes. More descriptive names such as array_sum or calculate_sum can be used to improve code readability.
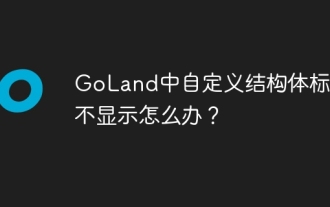
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
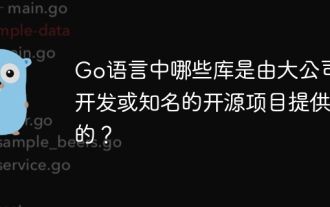
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
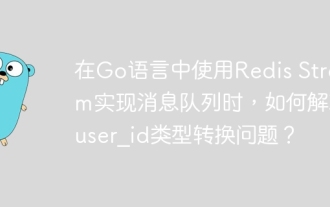
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
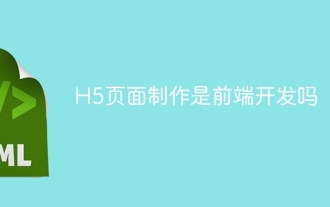
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
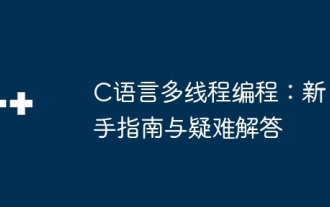
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
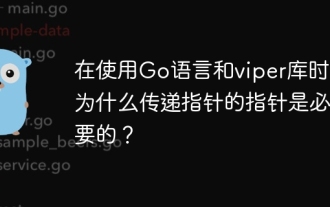
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
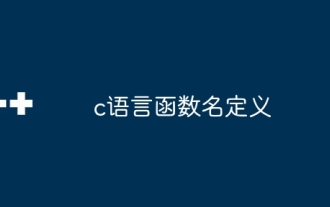
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
