Solving JavaScript:void conundrums: A comprehensive guide
The void operator is used in JavaScript to evaluate the value of an expression as undefined. Its usage includes: suppressing function return values, preventing unexpected behavior, checking whether the expression is "truthy", creating implicit conversions, and has higher priority. Low, not recommended for general use.
JavaScript: void puzzle: A comprehensive guide
What is void?
void
is an operator in JavaScript that evaluates an expression to undefined
. Unlike other operators, void
does not affect the execution of the expression.
Usage
void
The operator can only be used with one operand. The syntax is as follows:
void expression;
where expression
is the expression to be evaluated as undefined
.
Practical cases
The following are some practical cases of void
operators:
- Suppression function Return value of the call:
void myFunction(); // myFunction 返回一个值,但不会使用它
- Prevent unexpected behavior:
let x = void (y ?? 0); // 如果 y 为 null 或 undefined,则 x 将为 undefined,否则为 y
- Check expression Whether it is "truthy":
if (!void expression) { // 如果 expression 为 truthy,则不会执行此代码块 }
- Create implicit conversion:
const number = new Number(42); // 创建一个 Number 对象 const primitive = void number; // 获取原始值 42
Notes
void
operator does not throw an exception even if the operand is an invalid expression. Thevoid
operator has lower precedence than most other operators.void
Mainly used in special situations, not recommended in general.
Other examples
const result = void (prompt("Please enter your name:") || "Unknown"); // 获取用户输入,或使用 "Unknown" 作为默认值
let value; if (void value) { // 当 value 为 undefined 或 null 时,执行此代码块 }
The above is the detailed content of Solving JavaScript:void conundrums: A comprehensive guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


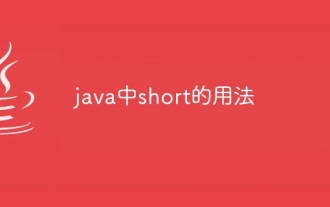
short is a primitive data type in Java that represents a 16-bit signed integer in the range -32,768 to 32,767. It is often used to represent small integers, such as counters or IDs, and supports basic arithmetic operations and type conversions. But since short is a signed type, you need to be careful when using division to avoid overflow or underflow.
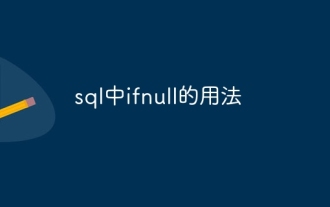
The IFNULL function checks whether an expression is NULL and returns the specified default value if so, otherwise it returns the value of the expression. It prevents null values from causing errors, allows manipulation of null values, and improves the readability of queries. Usage includes: replacing null values with default values, excluding null values from calculations, and nested usage to handle multiple null value situations.
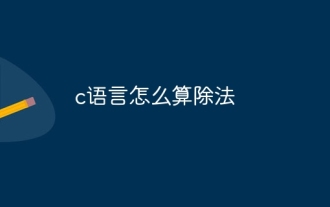
In C language, the behavior of the division operator / depends on the data type of the operands: Integer division: When the operand is an integer, integer division is performed and the result is rounded down. Floating point division: When the operand is a floating point number, floating point division is performed and the result is a floating point number. Type conversion: When one operand is an integer and the other is not, the integer is implicitly converted to a floating point number, and then floating point division is performed. Divisor by 0: A mathematical error occurs when the divisor is 0. Modulo operation: Use the % operator for modulo operation instead of modulo division.
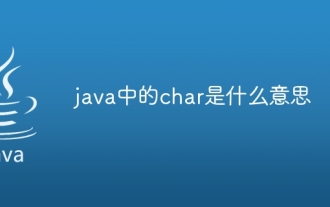
The char type in Java is used to store a single Unicode character, accounting for 2 bytes, ranging from U+0000 to U+FFFF. It is mainly used to store text characters. It can be initialized through single quotes or Unicode escape sequences, and can participate in comparison, Equality, inequality and join operations can be implicitly converted to int type or explicitly converted to Character objects.
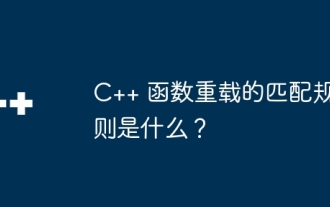
The C++ function overload matching rules are as follows: match the number and type of parameters in the call. The order of parameters must be consistent. The constness and reference modifiers must match. Default parameters can be used.
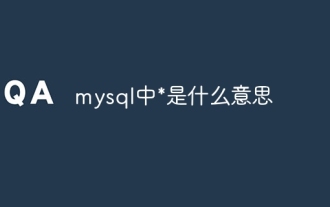
The asterisk (*) in MySQL means "all" and has different uses: Select all columns Select all rows JOIN wildcards for table LIKE clause Quantifier implicit type conversion for REGEXP clause
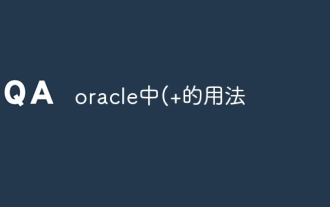
The plus (+) operator in Oracle can be used to: connect strings, numbers, dates, and time intervals; handle NULL values and convert NULL to non-NULL values; convert data types to string types.
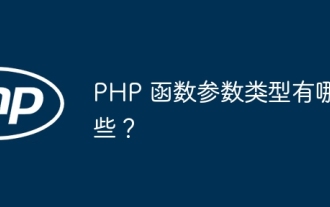
PHP function parameter types include scalar types (integers, floating point numbers, strings, Boolean values, null values), composite types (arrays, objects) and special types (callback functions, variable parameters). Functions can automatically convert parameters of different types, but they can also force specific types through type declarations to prevent accidental conversions and ensure parameter correctness.
