Using functions in PHP OOP: Q&A
There are two types of functions in PHP OOP: class methods and static methods. Class methods belong to a specific class and are called by instances of that class; static methods do not belong to any class and are called through the class name. Class methods are declared using public function and static methods are declared using public static function. Class methods are called through object instances ($object->myMethod()), and static methods are called directly through the class name (MyClass::myStaticMethod()).
Functions in PHP Object-Oriented Programming (OOP): Questions and Answers
Question: Functions in PHP OOP What are the types?
Answer: There are two types of functions in PHP OOP:
- Class methods: Functions belonging to a specific class, Can only be called by instances of this class.
- Static methods: Functions that do not belong to any specific class and can be called directly through the class name.
Q: How to declare a class method?
Answer: You can declare a class method using the following syntax:
class MyClass { public function myMethod() { ... } }
Q: How to declare a static method?
Answer: You can declare static methods using the following syntax:
class MyClass { public static function myStaticMethod() { ... } }
Q: How to call a class method?
Answer: You can use the following syntax to call class methods:
$object = new MyClass(); $object->myMethod();
Q: How to call a static method?
Answer: You can use the following syntax to call static methods:
MyClass::myStaticMethod();
Practical case: Create a class that calculates area
class Rectangle { private $width; private $height; public function setWidth($width) { $this->width = $width; } public function setHeight($height) { $this->height = $height; } public function getArea() { return $this->width * $this->height; } public static function calculateArea($width, $height) { return $width * $height; } } // 创建矩形对象 $rectangle = new Rectangle(); $rectangle->setWidth(10); $rectangle->setHeight(5); // 调用类方法计算面积 $area = $rectangle->getArea(); // 调用静态方法计算面积 $staticArea = Rectangle::calculateArea(10, 5); echo "类方法计算的面积:{$area}\n"; echo "静态方法计算的面积:{$staticArea}\n";
The above is the detailed content of Using functions in PHP OOP: Q&A. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


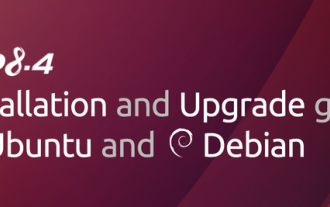
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
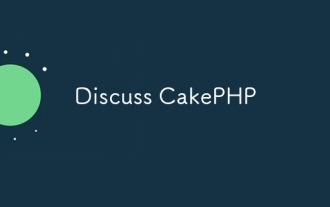
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
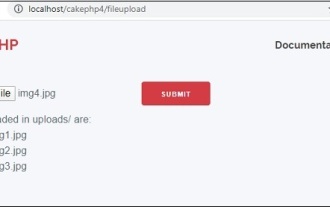
To work on file upload we are going to use the form helper. Here, is an example for file upload.
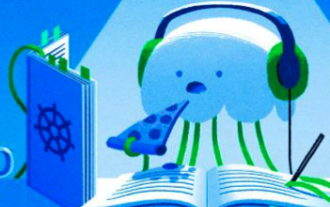
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
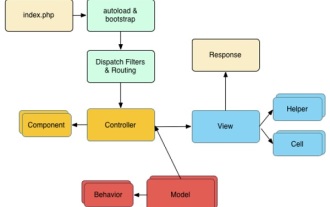
CakePHP is an open source MVC framework. It makes developing, deploying and maintaining applications much easier. CakePHP has a number of libraries to reduce the overload of most common tasks.
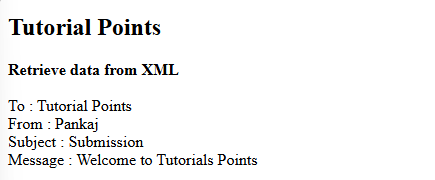
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
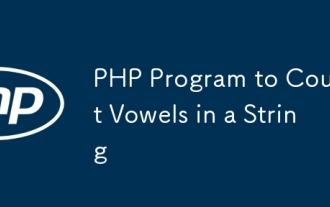
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
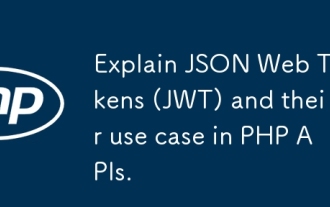
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
