How to pass object as function parameter in PHP?
In PHP, objects can be passed to functions by reference or value. Passing a reference allows the function to modify the original object, and passing a value creates a copy of the original object. In the actual case, the employee management system uses object reference passing to allow functions to modify the salary of the original employee object.
How to pass objects as function parameters in PHP?
In PHP, objects can be passed to functions by reference or value. Either way, the function will obtain a reference or copy of the object.
Passing Object Reference
Passing an object by reference allows a function to modify the original object. To do this, pass the object using the &
notation:
class Person { public $name; public function __construct($name) { $this->name = $name; } } function changeName(&$person) { $person->name = "John Doe"; } $person = new Person("Jane Doe"); changeName($person); echo $person->name; // 输出 "John Doe"
Passing the object by value
Passing the object by value creates a copy of the original object. This allows functions to modify the copy without affecting the original object:
class Person { public $name; public function __construct($name) { $this->name = $name; } } function changeName($person) { $person->name = "John Doe"; } $person = new Person("Jane Doe"); changeName($person); echo $person->name; // 输出 "Jane Doe"
Practical Case
Employee Management System
Suppose we have an employee management system , one of the functions needs to access employee information for modification.
class Employee { public $name; public $salary; } function updateSalary(Employee $employee, $newSalary) { $employee->salary = $newSalary; } $employee = new Employee(); $employee->name = "Jane Doe"; $employee->salary = 1000; updateSalary($employee, 1200); echo $employee->salary; // 输出 "1200"
In this case, passing the $employee
object using an object reference enables the updateSalary()
function to modify the salary of the original object.
The above is the detailed content of How to pass object as function parameter in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
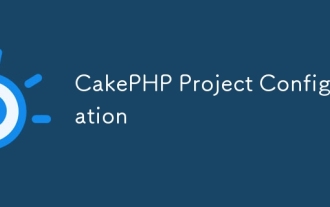
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
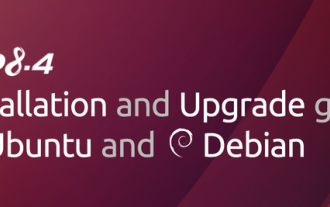
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
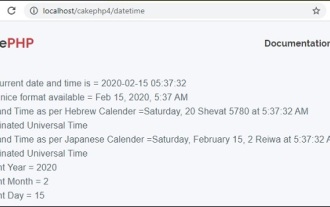
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
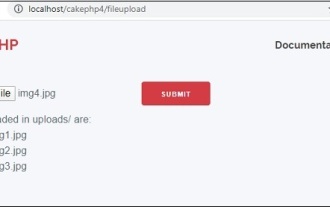
To work on file upload we are going to use the form helper. Here, is an example for file upload.
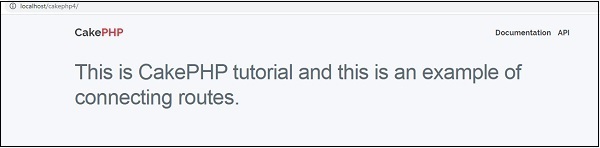
In this chapter, we are going to learn the following topics related to routing ?
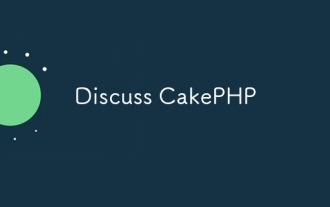
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
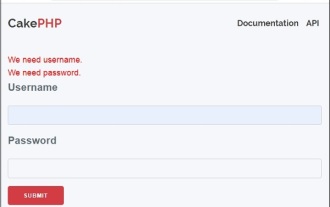
Validator can be created by adding the following two lines in the controller.
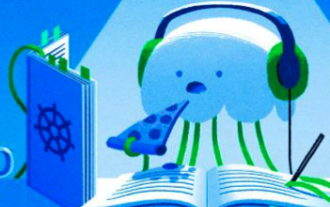
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
