How to define variable scope in Golang function?
In Go, function scope limits variable visibility to the function where the variable is declared: Declare a variable within a function: var name type = value Scope is limited to the declared code block, other functions or nested blocks These variables cannot be accessed
Function scope variable definition in Go
In Go, function scope determines the visibility of variables. Variables declared inside a function can only be accessed within that function.
Variable declaration
The way to declare variables in a function is as follows:
var name string = "Alice"
Among them:
var
Keywords Indicates declaring a new variable.name
is the name of the variable.string
is the type of the variable.= "Alice"
Initialize the value of the variable.
Scope
In Go, the scope of a variable is limited to the code block in which it is declared. This means that these variables cannot be accessed within other functions or nested blocks.
For example:
func main() { age := 20 fmt.Println(age) // 输出:20 } func other() { // age 未定义 fmt.Println(age) // 错误 }
Practical case: Calculating the area of a triangle
In order to demonstrate the function scope, we write a function to calculate the area of a triangle:
func area(base, height float64) float64 { // 定义局部变量面积 var area float64 // 计算三角形面积 area = 0.5 * base * height return area } func main() { // 在主函数中调用 area 函数并打印面积 fmt.Println(area(5.0, 10.0)) // 输出:25.0 }
In In the above example:
- Function
area
declares a local variablearea
. - Variable
area
is valid in functionarea
, but not in main functionmain
. - Main function
main
Usefmt.Println
to print the return value of thearea
function.
The above is the detailed content of How to define variable scope in Golang function?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


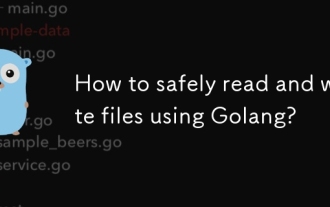
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
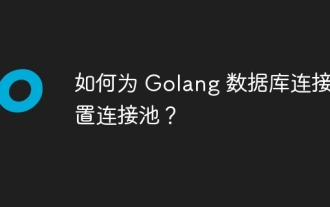
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
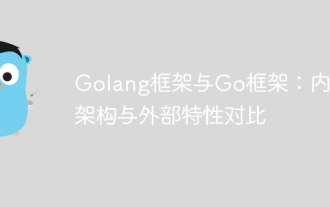
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
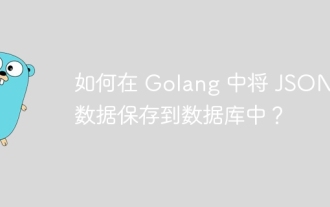
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
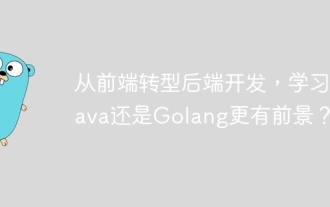
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
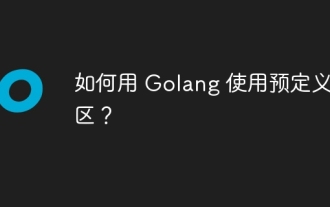
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
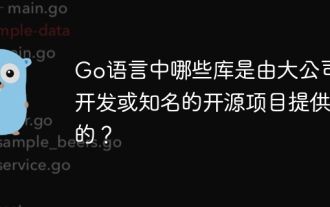
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
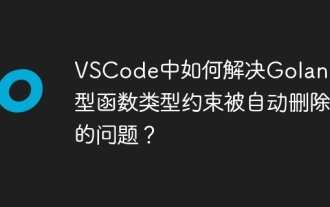
Automatic deletion of Golang generic function type constraints in VSCode Users may encounter a strange problem when writing Golang code using VSCode. when...
