What types of return values do PHP functions have?
PHP functions support returning various data types, including basic types (Boolean values, integers, floating point numbers, strings), composite types (arrays, objects), resource types (file handles, database handles), null values (NULL ) and void (introduced in PHP 8).
Return value type of PHP function
PHP function can return various data types, including:
- Scalar type: Boolean, integer, floating point number, string
- Composite type: Array, object
- Resources Type: File handle, MySQL connection handle
- Empty (NULL) type: No clear value
Actual case:
Function that returns a Boolean value:
<?php function is_prime(int $number): bool { // 对于 1 和 2,返回真 if ($number <= 2) { return true; } // 遍历 2 到 number 的平方根 for ($i = 2; $i <= sqrt($number); $i++) { if ($number % $i == 0) { return false; } } return true; }
Function that returns an array:
<?php function get_employee_data(int $employee_id): array { // 从数据库中查询员工数据 $result = $mysqli->query("SELECT * FROM employees WHERE id = $employee_id"); // 将结果封装到数组中 $employee_data = $result->fetch_assoc(); return $employee_data; }
Function that returns an object :
<?php class Employee { public $id; public $name; public $department; } function create_employee(string $name, string $department): Employee { $employee = new Employee(); $employee->name = $name; $employee->department = $department; return $employee; }
Function that returns null value:
<?php function get_file_contents(string $filename): ?string { if (file_exists($filename)) { return file_get_contents($filename); } return null; }
Note:
- ##PHP 7 and Later versions eliminated all return types except Boolean.
- In PHP 8, a new void return type was introduced to indicate that the function does not return any value.
The above is the detailed content of What types of return values do PHP functions have?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










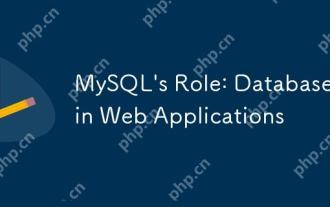
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
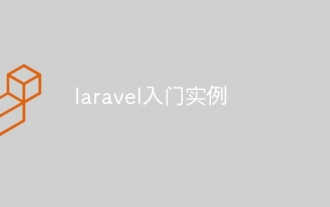
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.

MySQL and phpMyAdmin are powerful database management tools. 1) MySQL is used to create databases and tables, and to execute DML and SQL queries. 2) phpMyAdmin provides an intuitive interface for database management, table structure management, data operations and user permission management.
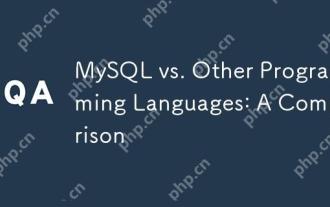
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
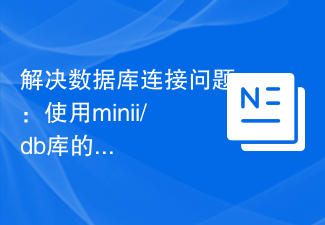
I encountered a tricky problem when developing a small application: the need to quickly integrate a lightweight database operation library. After trying multiple libraries, I found that they either have too much functionality or are not very compatible. Eventually, I found minii/db, a simplified version based on Yii2 that solved my problem perfectly.
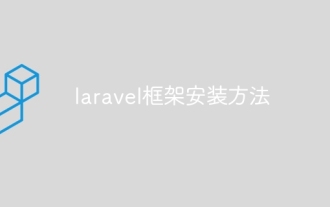
Article summary: This article provides detailed step-by-step instructions to guide readers on how to easily install the Laravel framework. Laravel is a powerful PHP framework that speeds up the development process of web applications. This tutorial covers the installation process from system requirements to configuring databases and setting up routing. By following these steps, readers can quickly and efficiently lay a solid foundation for their Laravel project.
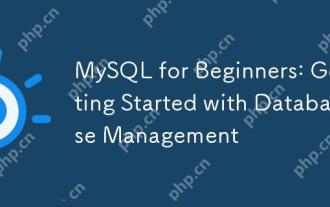
The basic operations of MySQL include creating databases, tables, and using SQL to perform CRUD operations on data. 1. Create a database: CREATEDATABASEmy_first_db; 2. Create a table: CREATETABLEbooks(idINTAUTO_INCREMENTPRIMARYKEY, titleVARCHAR(100)NOTNULL, authorVARCHAR(100)NOTNULL, published_yearINT); 3. Insert data: INSERTINTObooks(title, author, published_year)VA
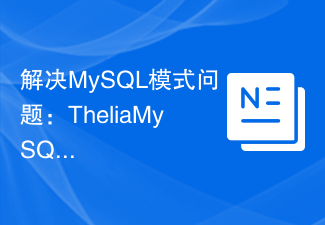
When developing an e-commerce website using Thelia, I encountered a tricky problem: MySQL mode is not set properly, causing some features to not function properly. After some exploration, I found a module called TheliaMySQLModesChecker, which is able to automatically fix the MySQL pattern required by Thelia, completely solving my troubles.
