How to implement closure function in Golang?
In Go, a closure function refers to an anonymous function that can access external variables. Implementation method: When creating a closure function, a reference to an external variable is formed. Practical applications include summing cumulative lists of numbers. Advantages: Improve code maintainability and reusability, encapsulate state, and create powerful callback functions.
Detailed explanation of closure function in Go language
What is closure function?
In Go, a closure function refers to an anonymous function that can access variables outside its definition scope. When a closure function captures external variables, it forms a reference, so even if the closure function is returned or passed to other functions, these external variables are still available.
How to implement closure function?
In Go, closure functions can be created in the following ways:
func main() { // 定义一个外部变量 x := 10 // 创建一个闭包函数 fn := func() { fmt.Println(x) // 访问外部变量 } // 执行闭包函数 fn() // 输出:10 }
In this example, fn
the closure function can access external variablesx
, even if the fn
function is executed outside the main
function.
Practical Case
Suppose we have a function that needs to calculate the sum of a list of numbers. We can use closure functions to accumulate sum values:
func main() { nums := []int{1, 2, 3, 4, 5} // 创建一个闭包函数来计算和值 sum := func() int { total := 0 for _, num := range nums { total += num } return total } // 调用闭包函数并打印和值 fmt.Println(sum()) // 输出:15 }
Advantages
Closure functions have the following advantages:
- Allow functions to access outside their definition scope variables to improve code maintainability and reusability.
- Helps encapsulate state so that it is not easily modified by external code.
- Ability to create powerful callback functions that can be called when events occur.
The above is the detailed content of How to implement closure function in Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
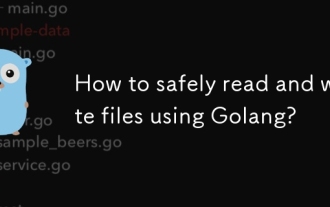
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
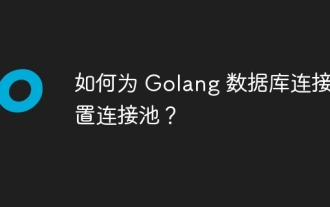
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
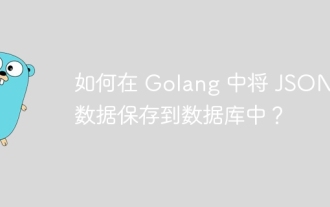
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
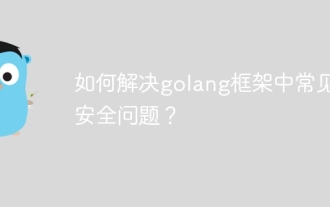
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
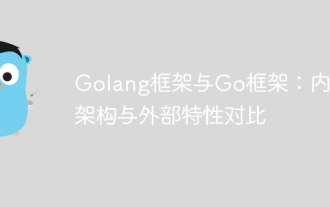
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
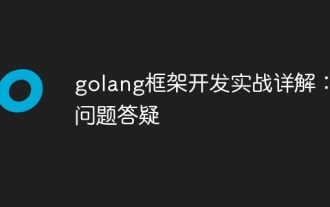
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
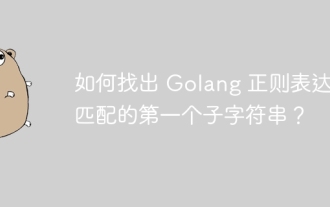
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
