Common errors and debugging techniques in C++ functions
Common C function errors: Return value error: Forgot or returned an unexpected value. Parameter error: Passed incorrect or missing parameters. Scope and lifetime error: accessing freed memory. Function pointer error: An error occurred while creating or using a function pointer. Function overloading error: Overload declaration is incorrect.
Common errors and debugging skills of C functions
C functions also have some common errors while having powerful functions. This article explores some common errors and provides practical debugging tips to help resolve them.
1. Function return value errors
Forgetting to return the specified value of a function or returning an unexpected value are common errors.
Debugging Tips:
- Use the debugger to set breakpoints and examine the returned value.
- Add a print statement in the function to track the value of the variable.
2. Function parameter errors
Passing incorrect parameters or forgetting to pass necessary parameters can lead to unexpected results.
Debugging Tips:
- Check the function declaration to make sure the call matches the expected arguments.
- Use the debugger to monitor the parameters and make sure the correct values are passed.
3. Function scope and life cycle errors
Practical example:
The following C function attempts to pass Reference returns a dynamically allocated array:
int* allocateArray(int size) { int* arr = new int[size]; return arr; } int main() { int* ptr = allocateArray(10); // 分配数组 delete[] ptr; // 释放数组 // 访问释放后的数组,导致未定义行为 ptr[0] = 10; return 0; }
Error:
Accessing a freed ptr
array causes undefined behavior.
Debugging tips:
- Use memory debugging tools such as Valgrind to detect memory access errors.
- Allocate arrays on the stack instead of using dynamic memory allocation to avoid lifetime issues.
4. Function pointer error
Practical example:
The following code attempts to create and call a function pointer:
void myFunction(int a, int b) { // ... } int main() { void (*functionPtr)(int, int) = &myFunction; // 创建函数指针 functionPtr(1, 2); // 调用函数指针 }
Error:
Forgot to take the address operator &
when calling functionPtr
, causing the function pointer to point to the wrong address.
Debugging Tips:
- Use a debugger such as GDB to inspect and verify the value of the function pointer.
- Ensure that the function pointer is set correctly, including the correct prototype and address operator.
5. Function overloading error
Practical example:
The following code is overloaded calculate
function, but there is an error:
int calculate(int a, int b) { return a + b; } double calculate(double a, double b) { return a + b; } int main() { // 尝试混合数据类型,导致编译器错误 int result = calculate(1, 2.5); }
Error:
Attempting to mix integer and floating point arguments results in a compiler error.
Debugging tips:
- Check the signatures of function overload declarations to ensure they do not overlap.
- Explicit casts, such as
static_cast
, to allow mixed data types.
The above is the detailed content of Common errors and debugging techniques in C++ functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
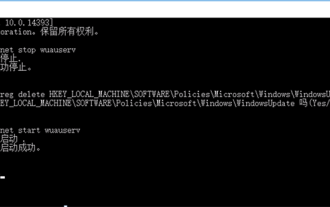
Table of Contents Solution 1 Solution 21. Delete the temporary files of Windows update 2. Repair damaged system files 3. View and modify registry entries 4. Turn off the network card IPv6 5. Run the WindowsUpdateTroubleshooter tool to repair 6. Turn off the firewall and other related anti-virus software. 7. Close the WidowsUpdate service. Solution 3 Solution 4 "0x8024401c" error occurs during Windows update on Huawei computers Symptom Problem Cause Solution Still not solved? Recently, the web server needs to be updated due to system vulnerabilities. After logging in to the server, the update prompts error code 0x8024401c. Solution 1

C++ object layout and memory alignment optimize memory usage efficiency: Object layout: data members are stored in the order of declaration, optimizing space utilization. Memory alignment: Data is aligned in memory to improve access speed. The alignas keyword specifies custom alignment, such as a 64-byte aligned CacheLine structure, to improve cache line access efficiency.
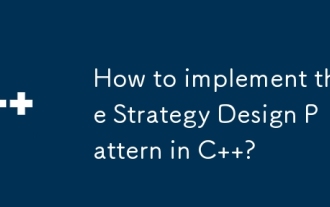
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
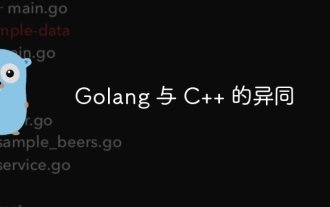
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
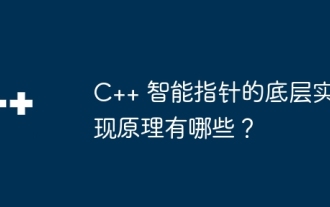
C++ smart pointers implement automatic memory management through pointer counting, destructors, and virtual function tables. The pointer count keeps track of the number of references, and when the number of references drops to 0, the destructor releases the original pointer. Virtual function tables enable polymorphism, allowing specific behaviors to be implemented for different types of smart pointers.
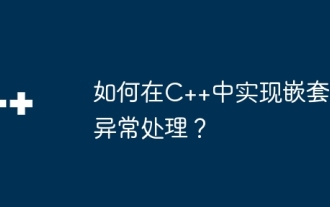
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
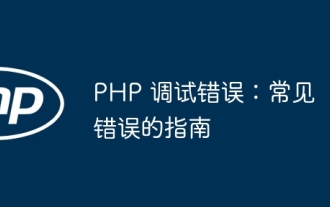
Common PHP debugging errors include: Syntax errors: Check the code syntax to make sure there are no errors. Undefined variable: Before using a variable, make sure it is initialized and assigned a value. Missing semicolons: Add semicolons to all code blocks. Function is undefined: Check that the function name is spelled correctly and make sure the correct file or PHP extension is loaded.
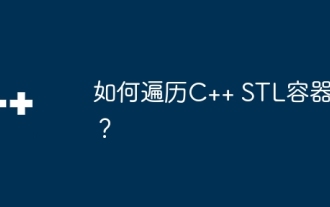
To iterate over an STL container, you can use the container's begin() and end() functions to get the iterator range: Vector: Use a for loop to iterate over the iterator range. Linked list: Use the next() member function to traverse the elements of the linked list. Mapping: Get the key-value iterator and use a for loop to traverse it.
