Java Concurrent Programming Best Practices
Java concurrent programming best practices include: 1. Use the synchronization mechanism correctly and choose the appropriate mechanism for synchronization; 2. Avoid deadlocks and be careful about the order of acquiring and releasing locks; 3. Use thread pools to manage threads to reduce overhead and Improve performance; 4. Pay attention to visibility issues and use volatile keywords or memory barriers to ensure thread visibility; 5. Use atomic operations to ensure that operations are uninterruptible when executed concurrently; 6. Handle exceptions correctly and use thread-safe processing technology.
Java Concurrent Programming Best Practices
Concurrent programming is crucial in modern software development, enabling applications to fully Take advantage of multi-core processors. Java provides rich support for concurrent programming, but implementing efficient and error-free concurrent code remains a challenge. This article will introduce the best practices of Java concurrent programming and illustrate it through practical cases.
1. Correct use of synchronization mechanism
The synchronization mechanism is the key to coordinating concurrent access to shared resources. Java provides a variety of synchronization mechanisms, including locks, synchronizers, and atomic variables. Choosing the right mechanism depends on the specific scenario and concurrency model. For example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
|
2. Avoid deadlock
Deadlock is a deadlock caused by two or more threads waiting for each other's resources. To avoid deadlocks, care should be taken in the order in which locks are acquired and released. Deadlock detection or prevention algorithms can be used to further reduce the possibility of deadlock.
3. Use thread pool to manage threads
Creating and destroying threads is an expensive operation. Use thread pools to manage thread resources, reduce overhead, and improve performance. The thread pool can allocate and recycle threads as needed.
1 2 3 4 5 6 7 |
|
4. Pay attention to visibility issues
In a multi-threaded environment, there may be problems with the visibility of shared variables. To ensure that threads see the latest changes made to shared variables, you can use the volatile keyword or other memory fence techniques.
5. Use atomic operations
Atomic operations ensure that an operation is uninterruptible when multiple threads execute concurrently. Classes like AtomicInteger and LongAdder in Java provide atomic operations.
1 2 3 4 5 6 |
|
6. Handle exceptions correctly
Exception handling in concurrent code is crucial because exceptions may cause thread interruption or data corruption. Thread-safe exception handling techniques should be used, such as Thread.UncaughtExceptionHandler
or ListeningExecutorService
from the Guava
library.
Practical Case
Consider a Web service that needs to process a large number of tasks concurrently. Using Java concurrent programming best practices, you can build an efficient and error-free solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
|
By applying these best practices, the web service can efficiently handle parallel tasks while maintaining thread safety and avoiding death Lock.
The above is the detailed content of Java Concurrent Programming Best Practices. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
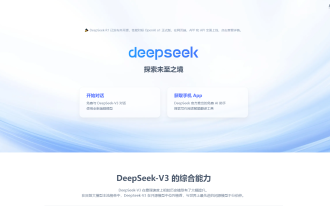
DeepSeek: How to deal with the popular AI that is congested with servers? As a hot AI in 2025, DeepSeek is free and open source and has a performance comparable to the official version of OpenAIo1, which shows its popularity. However, high concurrency also brings the problem of server busyness. This article will analyze the reasons and provide coping strategies. DeepSeek web version entrance: https://www.deepseek.com/DeepSeek server busy reason: High concurrent access: DeepSeek's free and powerful features attract a large number of users to use at the same time, resulting in excessive server load. Cyber Attack: It is reported that DeepSeek has an impact on the US financial industry.
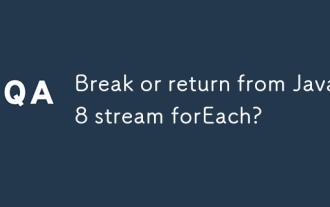
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
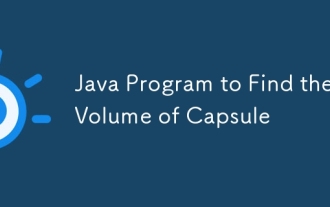
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
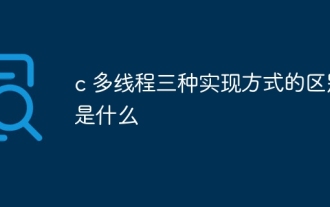
Multithreading is an important technology in computer programming and is used to improve program execution efficiency. In the C language, there are many ways to implement multithreading, including thread libraries, POSIX threads, and Windows API.
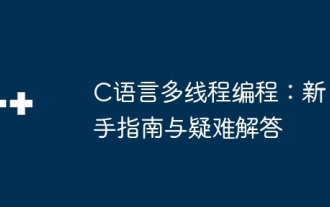
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
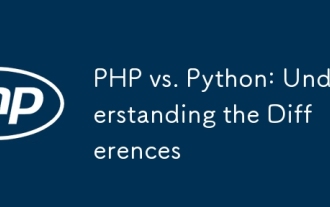
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
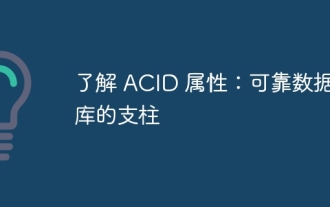
Detailed explanation of database ACID attributes ACID attributes are a set of rules to ensure the reliability and consistency of database transactions. They define how database systems handle transactions, and ensure data integrity and accuracy even in case of system crashes, power interruptions, or multiple users concurrent access. ACID Attribute Overview Atomicity: A transaction is regarded as an indivisible unit. Any part fails, the entire transaction is rolled back, and the database does not retain any changes. For example, if a bank transfer is deducted from one account but not increased to another, the entire operation is revoked. begintransaction; updateaccountssetbalance=balance-100wh
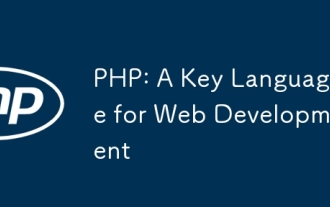
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
