How to optimize performance of Golang functions?
Ways to optimize Go function performance include: Reduce allocations: Reduce memory allocations by using stack variables or pre-allocated loop variables. Inline functions: For small functions, the overhead of function calls is eliminated through inlining. Optimize loops: Eliminating range checks, allocating variables in advance, and avoiding unnecessary append operations can improve loop efficiency.
Go function performance optimization
Introduction
Go language is famous for its high efficiency and concurrency performance famous. Function performance is critical to optimizing the overall performance of your application. This article explores several techniques for improving the performance of Go functions.
Reduce Allocation
Allocating memory is an expensive operation. By using the stack instead of the heap, you can reduce the number of allocations. For example:
func fibonacci(n int) int { if n <= 1 { return n } // 使用堆栈变量。 prev, next := 1, 1 for i := 2; i < n; i++ { prev, next = next, prev+next } return next }
Inline function
The function کوچک (
//go:inline func minus(a, b int) int { return a - b }
Optimizing Loops
Loops are a common performance bottleneck in code. When optimizing loops, you should pay attention to the following points:
- Cancel range checking: Use
for i := range arr
instead offor i : = 0; i < len(arr); i
can eliminate range checks and improve performance. - Allocation in advance: Allocation of loop variables in advance can reduce the number of allocations.
- Avoid unnecessary
append
operations: Performing multipleappend
operations on a slice can be very inefficient. Consider usingslice.XCopyY
to copy the slice directly.
Practical case
The following example compares the performance of unoptimized and optimized Fibonacci
functions:
Unoptimized:
func fibonacci(n int) int { if n == 0 { return 0 } if n <= 2 { return 1 } return fibonacci(n-1) + fibonacci(n-2) }
Optimized:
func fibonacciOpt(n int) int { if n <= 1 { return n } // 使用堆栈变量。 var a, b, c int = 0, 1, 0 for i := 2; i < n; i++ { c = a + b a = b b = c } return c }
Using go test
benchmark, you can observe The optimized function is 3 times faster than the unoptimized function:
BenchmarkFibonacciOpt-8 549903100 2.16 ns/op BenchmarkFibonacci-8 189643692 5.60 ns/op
The above is the detailed content of How to optimize performance of Golang functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
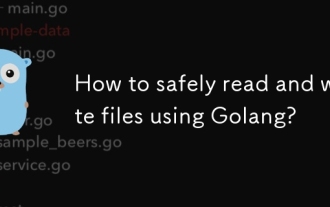
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
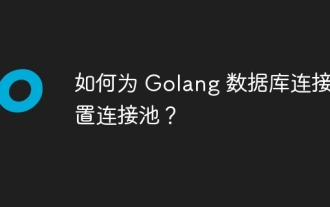
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
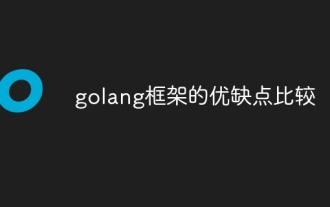
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
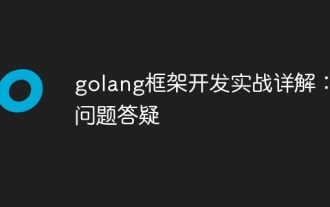
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
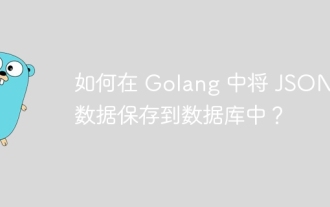
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
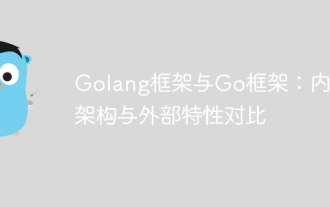
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
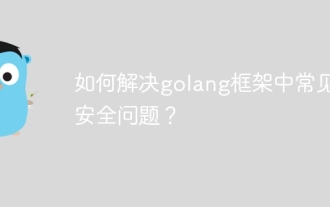
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
