


Pitfalls and preventive measures for common mistakes in PHP functions
Common error traps in PHP functions include: lack of parameter type checking, resulting in type errors. Default parameter values are immutable and may cause unexpected results if modified. Misusing return values and not handling potential errors or exceptions properly. Namespace conflict, resulting in function call error. Recursive call stack overflow, lack of clear exit conditions.
Traps and Precautions for Common Mistakes with PHP Functions
PHP functions are the basic building blocks in programming, but if used carelessly, they can cause Common mistakes. This article highlights common error traps often encountered in PHP functions and provides steps on how to avoid or prevent them.
1. Lack of parameter type checking
Trap: Not specifying the type of a function parameter can lead to type errors, especially when handling user input.
Precautions: Use PHP type hints, or use functions such as filter_input()
to enforce data types.
Practical case:
function addNumbers(int $a, int $b) { return $a + $b; } echo addNumbers("10", 20); // TypeError: Argument 1 passed to addNumbers() must be of the type integer, string given
2. The default parameter value is immutable
Trap: Although functions can have default parameter values , but these values are immutable inside the function. Attempting to modify them can lead to unexpected results.
Precautions: Avoid modifying default parameter values. If a dynamic value is required, pass it as a parameter.
Practical case:
function greet($name = "John") { $name = "Alice"; echo "Hello, $name!"; } greet(); // 输出:Hello, John!
3. Misuse of return value
Trap: The function returns a value, but if not Handled or used incorrectly, it may cause errors.
Precautions: Always check return values and handle any potential errors or exceptions.
Practical case:
function readFile($filename) { if (!file_exists($filename)) { return false; // 返回布尔值表示文件不存在 } $content = file_get_contents($filename); return $content; // 返回文件内容 } $contents = readFile("non-existent-file.txt"); if ($contents === false) { // 检查返回值 echo "File not found"; } else { echo $contents; }
4. Namespace conflict
Trap: When multiple namespaces use the same function name Namespace conflicts may occur.
Precautions: Always fully qualify function names in a namespace, or use aliases to avoid conflicts.
Practical case:
namespace App; function greet() { echo "Hello from App namespace"; } namespace Vendor; function greet() { echo "Hello from Vendor namespace"; } greet(); // 输出:Hello from Vendor namespace (由于命名空间冲突)
5. Recursive call stack overflow
Trap: When a function calls itself recursively Without appropriate boundary conditions, a recursive call stack overflow error can occur.
Precautions: Set explicit exit conditions in recursive functions to terminate the call chain.
Practical case:
function factorial($n) { if ($n <= 1) { return 1; } return $n * factorial($n-1); // 递归调用 } factorial(10000); // 导致调用栈溢出
The above is the detailed content of Pitfalls and preventive measures for common mistakes in PHP functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


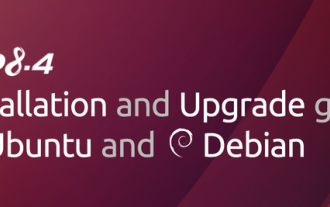
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
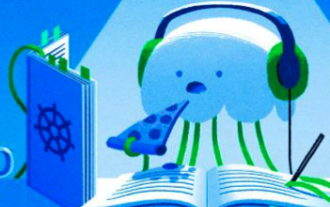
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
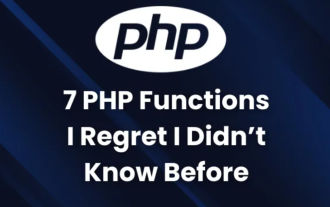
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
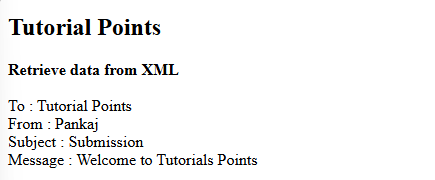
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
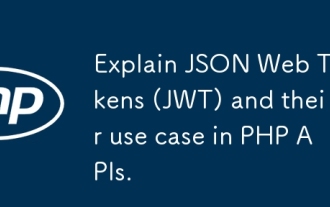
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
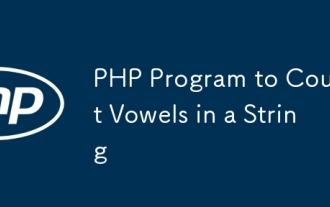
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
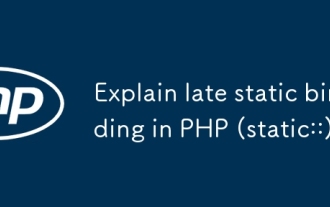
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
