How are Golang functions used in command line tools?
Go functions can break down the complex functionality of command-line tools into manageable modules, thereby improving code maintainability and readability. The following steps illustrate the role of Go functions in command line tools: Define a function to perform a specific task. Call the function in the main function, passing in the appropriate parameters. Functions perform tasks and return results. The main function processes the results and performs subsequent operations.
#How do Go functions work in command line tools?
Go functions are powerful tools that allow you to write flexible and reusable code. They are not limited to large applications, they can also be used to create command line tools.
Understanding command line tools
Command line tools are a type of program that accept commands entered by users through the terminal. They are typically used to perform specific tasks such as file operations, text processing, or system configuration.
The role of functions in command line tools
Functions can help you break down the complex functionality of command line tools into smaller, manageable modules. By breaking each task into a separate function, you can enhance the maintainability and readability of your code.
Practical case
Let us create a simple command line tool for outputting the length of a string:
package main import "fmt" // 定义一个函数来计算字符串的长度 func strLen(s string) int { return len(s) } func main() { // 获取用户输入的字符串 var str string fmt.Print("请输入一个字符串:") fmt.Scanln(&str) // 调用函数计算长度 length := strLen(str) // 输出长度 fmt.Printf("字符串长度:%d\n", length) }
How to use Function
In this example, we define the strLen
function, which accepts a string and returns its length. In the main
function, we call the strLen
function to calculate the length of the user input string.
Conclusion
Functions are powerful tools when creating command line tools. By breaking tasks into smaller units, you can create tools that are robust, maintainable, and easily extensible.
The above is the detailed content of How are Golang functions used in command line tools?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


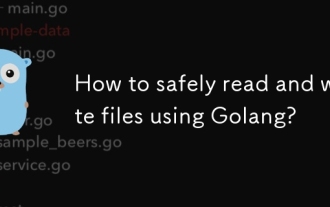
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
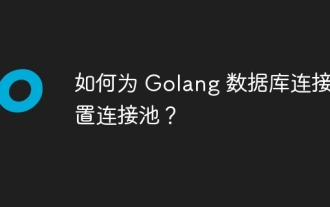
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
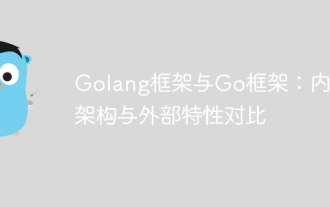
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
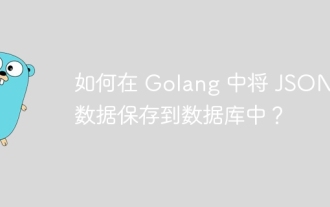
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
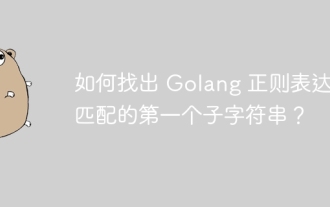
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
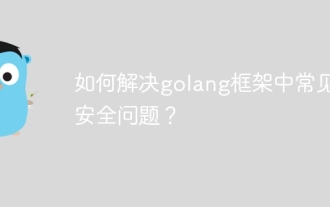
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
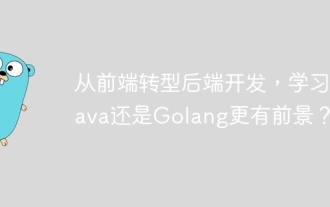
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
