How to avoid code duplication within Golang functions?
To avoid code duplication in Go functions, you can use the following methods: Use inline functions: embed the function body into the function call and reduce the number of lines of code. Use anonymous functions: Define functions without a name that can be executed immediately or passed to other functions without naming and calling. Create an extension library: encapsulate common logic and import the same code block into multiple functions to achieve code reuse.
How to avoid code duplication within Go functions
Introduction
When writing When writing Go code, you may encounter code duplication, which can lead to code that is difficult to maintain and error-prone. To avoid code duplication, you can use several features provided by Go.
Using inline functions
Inline functions allow you to embed the body of a function within a call to another function. This eliminates the need to create separate functions, thereby reducing lines of code and potential errors.
func main() { fmt.Println(sum(1, 2)) } func sum(a, b int) int { return a + b }
Using Anonymous Functions
Anonymous functions allow you to define a function without a name that can be executed immediately or passed to another function. This eliminates the need to name the function and call it.
func main() { fmt.Println(func(a, b int) int { return a + b }(1, 2)) }
Create your own extension library
If you often use the same block of code for multiple functions, you may consider creating your own extension library and importing it in your program. This helps encapsulate common logic and simplify code.
// utils.go package utils import "fmt" // Sum returns the sum of two integers. func Sum(a, b int) int { return a + b } // main.go package main import "github.com/username/customlib/utils" func main() { fmt.Println(utils.Sum(1, 2)) }
Practical Case
Suppose you have a function that needs to perform multiple operations on the input string, such as trimming, uppercase and lowercase conversion. You don't want to split these operations into separate functions, but you want to avoid code duplication.
You can use an anonymous function to avoid this situation:
func formatString(s string) string { f := func(op string) string { switch op { case "trim": return strings.TrimSpace(s) case "upper": return strings.ToUpper(s) case "lower": return strings.ToLower(s) default: return s } } return f("trim") + f("upper") + f("lower") }
This function can be called as follows:
s := " Hello, World! " fmt.Println(formatString(s)) // 输出: // HELLO, WORLD!hello, world!
The above is the detailed content of How to avoid code duplication within Golang functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


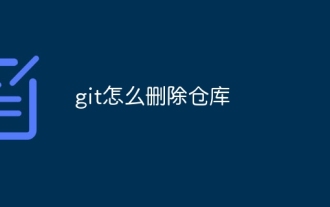
To delete a Git repository, follow these steps: Confirm the repository you want to delete. Local deletion of repository: Use the rm -rf command to delete its folder. Remotely delete a warehouse: Navigate to the warehouse settings, find the "Delete Warehouse" option, and confirm the operation.

Connecting a Git server to the public network includes five steps: 1. Set up the public IP address; 2. Open the firewall port (22, 9418, 80/443); 3. Configure SSH access (generate key pairs, create users); 4. Configure HTTP/HTTPS access (install servers, configure permissions); 5. Test the connection (using SSH client or Git commands).
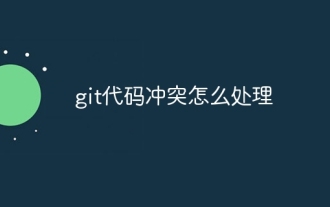
Code conflict refers to a conflict that occurs when multiple developers modify the same piece of code and cause Git to merge without automatically selecting changes. The resolution steps include: Open the conflicting file and find out the conflicting code. Merge the code manually and copy the changes you want to keep into the conflict marker. Delete the conflict mark. Save and submit changes.
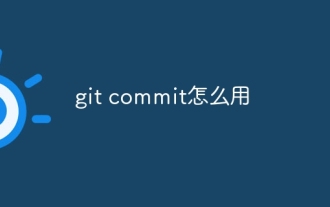
Git Commit is a command that records file changes to a Git repository to save a snapshot of the current state of the project. How to use it is as follows: Add changes to the temporary storage area Write a concise and informative submission message to save and exit the submission message to complete the submission optionally: Add a signature for the submission Use git log to view the submission content
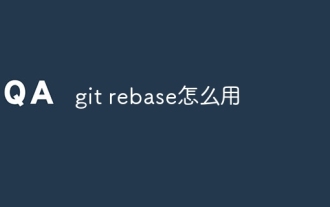
git rebase is used to reapply commits to a new baseline to clean up history or relocate branches. How to use: Create a target branch Select the commit to be reapplied and execute the git rebase command, specify the target branch and commit scope to resolve conflicts, continue to reapply the remaining commit verification changes.

To submit an empty folder in Git, just follow the following steps: 1. Create an empty folder; 2. Add the folder to the staging area; 3. Submit changes and enter a commit message; 4. (Optional) Push the changes to the remote repository. Note: The name of an empty folder cannot start with . If the folder already exists, you need to use git add --force to add.
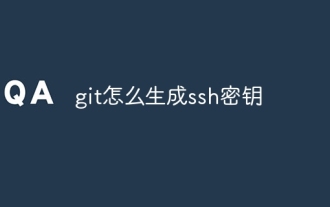
In order to securely connect to a remote Git server, an SSH key containing both public and private keys needs to be generated. The steps to generate an SSH key are as follows: Open the terminal and enter the command ssh-keygen -t rsa -b 4096. Select the key saving location. Enter a password phrase to protect the private key. Copy the public key to the remote server. Save the private key properly because it is the credentials for accessing the account.
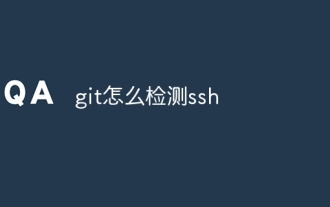
To detect SSH through Git, you need to perform the following steps: Generate an SSH key pair. Add the public key to the Git server. Configure Git to use SSH. Test the SSH connection. Solve possible problems according to actual conditions.
