PHP function performance optimization tips
PHP function performance optimization tips include: avoiding unnecessary object creation; reducing the number of function calls; using function caching; optimizing database queries; using third-party tools to analyze and optimize performance.
PHP function performance optimization tips
Performance optimization of PHP functions is crucial because it can greatly improve the response of the application speed. This article will introduce several practical optimization techniques to help you improve the performance of PHP functions.
1. Avoid creating unnecessary objects
Creating objects is a time-consuming operation, and unnecessary object creation should be avoided as much as possible. Consider using static methods or caching the object in a class.
Example:
// 不使用对象 function get_current_date() { return date('Y-m-d'); } // 使用静态方法 class DateHelper { public static function get_current_date() { return date('Y-m-d'); } }
2. Reduce the number of function calls
Each function call will increase the overhead, by reducing the number of function calls The number of calls can improve performance. Consider using temporary variables or caching results to reduce the number of calls.
Example:
// 减少函数调用次数 function calculate_average($data) { $sum = 0; $count = 0; foreach ($data as $value) { $sum += $value; $count++; } return $sum / $count; }
3. Using function cache
Frequently called functions can be accelerated by using cache. PHP has a built-in accelerator
extension that can be used to cache results for functions.
Example:
// 使用函数缓存 function get_cached_data() { $cache = new Cache(); $data = $cache->get('my_data'); if (!$data) { $data = load_data_from_database(); $cache->set('my_data', $data); } return $data; }
4. Optimize database queries
Database queries are a common performance bottleneck in PHP applications. Database queries can be optimized using indexes, proper connection pooling, and query caching.
Example:
// 使用索引优化查询 $sql = 'SELECT * FROM users WHERE username LIKE :username'; $stmt = $db->prepare($sql); $stmt->execute([':username' => '%john%']);
5. Use third-party tools
There are many third-party tools that can help analyze and optimize PHP Function performance. For example, Xdebug can be used to analyze execution time and memory consumption.
By applying these tips, you can significantly improve the performance of your PHP functions, thereby improving your application's responsiveness and user experience.
The above is the detailed content of PHP function performance optimization tips. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


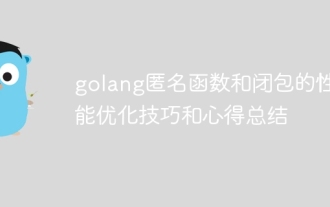
Although anonymous functions and closures are anonymous in Go, improper use will affect performance. In order to optimize closures, you can avoid unnecessary copies, reduce the number of captured variables, use the peephole optimizer and inlining, and finally determine the effectiveness through benchmark testing.
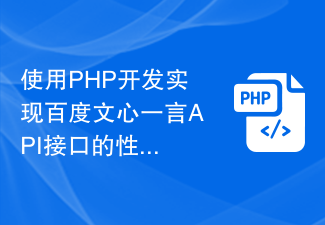
Performance optimization techniques for using PHP to develop and implement Baidu Wenxin Yiyan API interface. With the popularity of the Internet, more and more developers use third-party API interfaces to obtain data to enrich their application content. Baidu Wenxin Yiyan API interface is a popular data interface. It can return a random inspirational, philosophical or warm sentence, which can be used to beautify the program interface, increase user experience, etc. However, when using the Baidu Wenxinyiyan API interface, we also face some performance considerations. API call speed
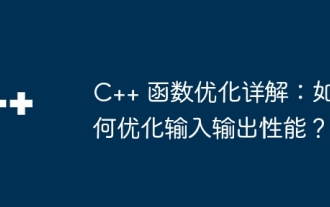
The input and output performance in C++ can be improved through the following optimization techniques: 1. Using file pointers; 2. Using streams; 3. Using cache; 4. Optimizing I/O operations (batch I/O, asynchronous I/O, memory mapped I/O) /O).
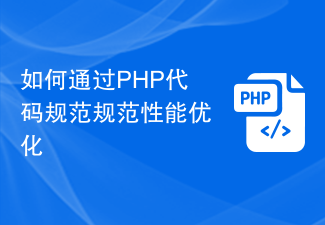
How to standardize performance optimization through PHP code specifications Introduction: With the rapid development of the Internet, more and more websites and applications are developed based on the PHP language. In the PHP development process, performance optimization is a crucial aspect. A high-performance PHP code can significantly improve the website's response speed and user experience. This article will explore how to standardize performance optimization through PHP code specifications and provide some practical code examples for reference. 1. Reduce database queries. Frequent database queries are a common feature during the development process.
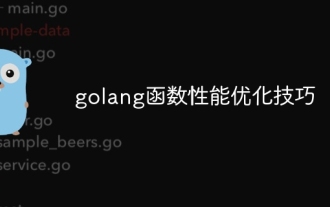
Go function performance can be optimized with the following tips: Use caching to avoid double calculations. Use goroutines to concurrentize calculations to improve efficiency. Use assembly code for critical calculations to improve performance. Choose appropriate data structures, such as slices, maps, and channels, to optimize data storage and retrieval. Avoid unnecessary memory allocations to reduce performance overhead. Inline frequently called functions to reduce calling overhead.
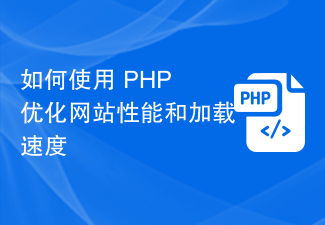
How to use PHP to optimize website performance and loading speed With the rapid development of the Internet, website performance and loading speed have attracted more and more attention. As a widely used server-side scripting language, PHP plays an important role in optimizing website performance and loading speed. This article will introduce some tips and methods for using PHP to improve the performance and loading speed of your website. Using a caching mechanism Caching is an effective way to improve website performance. PHP provides a variety of caching mechanisms, such as file caching, memory caching and data caching.
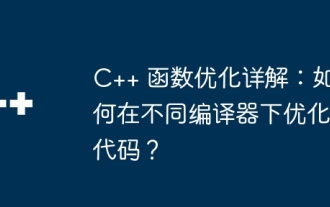
Functions can be optimized in C++ to improve code performance and save resources through measures such as preprocessing optimizations (such as macro definitions), compiler flag optimizations (such as -O2), and inlining and loop optimizations. Specific optimization steps include: 1. Use preprocessing instructions for macro definition and preprocessing; 2. Use compiler flags to specify optimization settings, such as -O2; 3. Mark functions through the inline keyword so that they can be inlined at compile time; 4. Apply Loop optimization techniques such as loop unrolling and loop vectorization. Through these optimizations, we can significantly improve program performance.
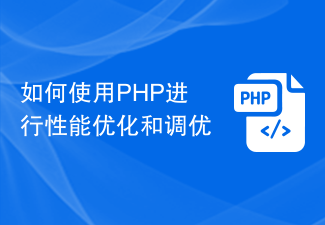
How to use PHP for performance optimization and tuning In the process of developing web applications, performance optimization and tuning are important tasks that cannot be ignored. As a popular server-side scripting language, PHP also has some techniques and tools that can improve performance. This article will introduce some common PHP performance optimization and tuning methods, and provide sample code to help readers better understand. Using cache caching is one of the important means to improve the performance of web applications. You can reduce access to the database and reduce IO operations to improve performance by using cache. make
