How to calculate several powers in C language
There are three ways to calculate powers: using the pow() function (fastest, but requires an external library), using a loop (simple, but inefficient), and using recursion (elegant, but may cause stack overflow).
How to use C language to calculate power
Use the pow() function directly
#include <math.h> int main() { double base = 2.0; int exponent = 3; double result = pow(base, exponent); printf("(%f) ^ %d = %f\n", base, exponent, result); return 0; }
Use loops
int main() { double base = 2.0; int exponent = 3; double result = 1.0; for (int i = 0; i < exponent; i++) { result *= base; } printf("(%f) ^ %d = %f\n", base, exponent, result); return 0; }
Use recursion
double power(double base, int exponent) { if (exponent == 0) { return 1.0; } else if (exponent < 0) { return 1.0 / power(base, -exponent); } else { return base * power(base, exponent - 1); } } int main() { double base = 2.0; int exponent = 3; double result = power(base, exponent); printf("(%f) ^ %d = %f\n", base, exponent, result); return 0; }
Which method you choose depends on performance and code readability .
- pow() function is the fastest, but it requires an external library.
- The loop method is simple, but it is inefficient for large exponent.
- The recursive approach is elegant, but it can cause stack overflow.
The above is the detailed content of How to calculate several powers in C language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


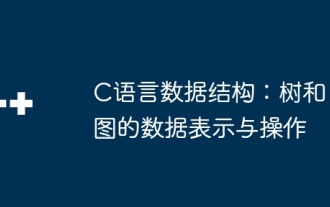
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
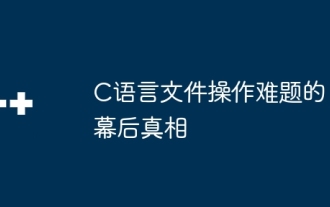
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
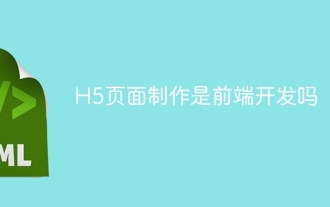
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
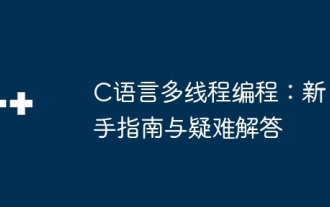
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
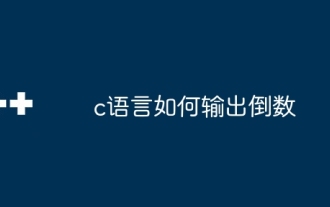
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
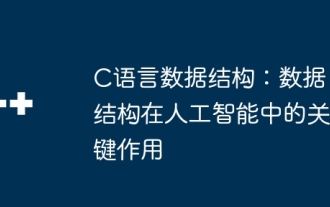
C Language Data Structure: Overview of the Key Role of Data Structure in Artificial Intelligence In the field of artificial intelligence, data structures are crucial to processing large amounts of data. Data structures provide an effective way to organize and manage data, optimize algorithms and improve program efficiency. Common data structures Commonly used data structures in C language include: arrays: a set of consecutively stored data items with the same type. Structure: A data type that organizes different types of data together and gives them a name. Linked List: A linear data structure in which data items are connected together by pointers. Stack: Data structure that follows the last-in first-out (LIFO) principle. Queue: Data structure that follows the first-in first-out (FIFO) principle. Practical case: Adjacent table in graph theory is artificial intelligence
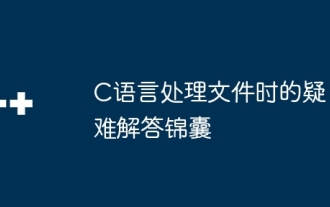
Troubleshooting Tips for C language processing files When processing files in C language, you may encounter various problems. The following are common problems and corresponding solutions: Problem 1: Cannot open the file code: FILE*fp=fopen("myfile.txt","r");if(fp==NULL){//File opening failed} Reason: File path error File does not exist without file read permission Solution: Check the file path to ensure that the file has check file permission problem 2: File reading failed code: charbuffer[100];size_tread_bytes=fread(buffer,1,siz
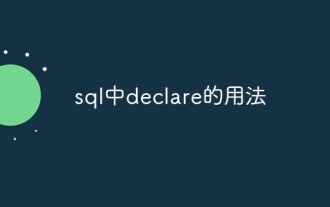
The DECLARE statement in SQL is used to declare variables, that is, placeholders that store variable values. The syntax is: DECLARE <Variable name> <Data type> [DEFAULT <Default value>]; where <Variable name> is the variable name, <Data type> is its data type (such as VARCHAR or INTEGER), and [DEFAULT <Default value>] is an optional initial value. DECLARE statements can be used to store intermediates
