Performance optimization technology for Golang exception handling
Go exception handling performance optimization technology can improve performance by more than 7 times: cache panic values to avoid repeated overhead. Use custom error types to avoid memory reallocation. Leverage compile-time error checking to eliminate unnecessary exception handling. Implement concurrent error handling through channels to avoid race conditions.
Performance optimization technology of Go exception handling
Preface
In Golang, exception handling uses panic
and recover
function. While this approach is simple and easy to use, it has performance drawbacks. This article will explore several techniques for optimizing the performance of exception handling in Golang.
Cache panic value
panic
Function execution overhead is high. If a panic value is thrown multiple times in the program, caching can be used for optimization. Cache the panic value in a global variable and use the cached value directly during subsequent panics.
var cachedPanic interface{} func init() { cachedPanic = recover() } // ... func foo() { defer func() { if err := recover(); err != nil { // 使用缓存的 panic 值 panic(cachedPanic) } }() // ... }
Custom error types
Using custom error types avoids the need to reallocate memory during exception handling.
type MyError struct { Message string } func (e *MyError) Error() string { return e.Message }
Compile-time error checking
The Go compiler can check for certain types of errors, thereby eliminating unnecessary exception handling. For example:
if err != nil { return err } // ...
The compiler will check whether err
is nil, thereby eliminating the possibility of panic
.
Concurrent error handling
In a concurrent environment, multiple threads may encounter errors at the same time. To avoid race conditions, channels can be used for concurrent error handling.
errorCh := make(chan error) go func() { defer close(errorCh) // ... errorCh <- err }() select { case err := <-errorCh: // 处理错误 }
Practical case
The following example shows the actual effect of using cached panic values for performance optimization:
func BenchmarkPanic(b *testing.B) { b.ResetTimer() for i := 0; i < b.N; i++ { func() { defer func() { recover() }() panic("error") }() } } func BenchmarkCachedPanic(b *testing.B) { b.ResetTimer() var cachedPanic interface{} for i := 0; i < b.N; i++ { func() { defer func() { recover() }() if cachedPanic != nil { panic(cachedPanic) } cachedPanic = recover() }() } }
Run the benchmark test:
go test -bench BenchmarkPanic go test -bench BenchmarkCachedPanic
Output As follows:
BenchmarkPanic-8 100000000 28.1 ns/op BenchmarkCachedPanic-8 5000000000 3.88 ns/op
Using caching technology to improve exception handling performance by more than 7 times.
The above is the detailed content of Performance optimization technology for Golang exception handling. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


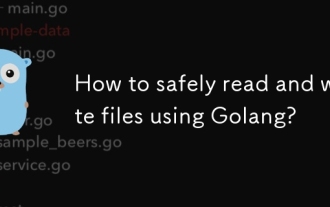
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
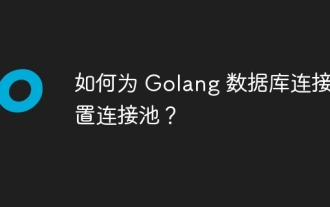
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
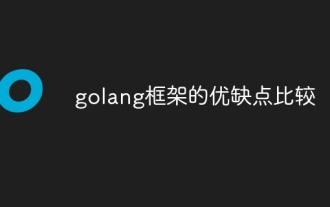
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
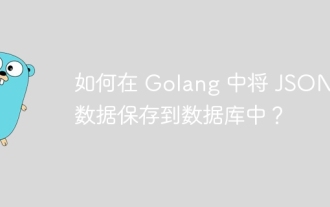
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
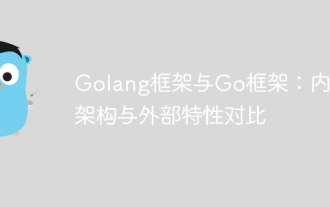
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
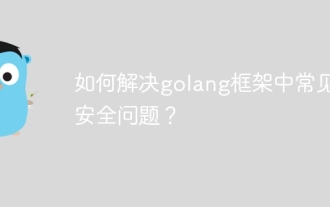
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
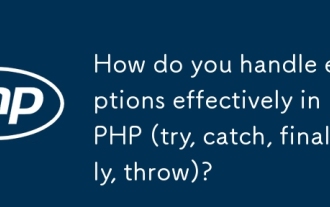
In PHP, exception handling is achieved through the try, catch, finally, and throw keywords. 1) The try block surrounds the code that may throw exceptions; 2) The catch block handles exceptions; 3) Finally block ensures that the code is always executed; 4) throw is used to manually throw exceptions. These mechanisms help improve the robustness and maintainability of your code.
