


How does the parameter passing method of PHP functions affect function dependency injection?
PHP parameter passing method has the following effects on Function Dependency Injection (FDI): when passing by value, the function cannot modify the original variable, and FDI cannot be implemented; passing by reference allows the function to modify the original variable, and FDI is supported but should be used with caution; Pass-and-return by value allows a function to return a new variable containing the passed variable, supports FDI and is more secure.
The impact of PHP function parameter passing method on function dependency injection
Parameter passing method
PHP functions support three parameter passing methods:
- Pass by value (default): Pass a copy of the variable value to the function.
- Pass by reference: Directly pass the variable address to the function, and the function can modify the original variable value.
- Pass by value and return: The function will return a new variable containing the passed variable, which can be obtained from outside the function.
Functional Dependency Injection
Functional Dependency Injection (FDI) is a design pattern that allows a function to receive its dependencies from the outside instead of hard-coding them inside the function body. With FDI we can create code that is loosely coupled, easy to test and maintain.
Parameter passing methods and FDI
Different parameter passing methods have the following effects on FDI:
Passing by value
- When parameters are passed by value, the function cannot modify the original variable, defeating the purpose of FDI.
- In order to implement FDI, the function needs to be implemented by returning a new variable containing the passed variable.
Pass by reference
- Pass by reference allows a function to modify the original variable, thus supporting FDI.
- However, reference parameters need to be used with caution as it increases potential side effects and reduces code flexibility.
Pass by value and return
- Pass by value and return allows a function to return a new variable containing the passed variable, supporting FDI.
- This method is safer than passing by reference because it does not modify the original variable.
Practical case: database connection
Consider a function that connects to the database:
function get_connection() { $conn = new mysqli('localhost', 'user', 'password', 'database'); return $conn; }
If we want to change the database connection configuration through FDI , passing by value will not work. Instead, we can use pass by value and return:
function get_connection_config(array $config) { $conn = new mysqli($config['host'], $config['user'], $config['password'], $config['database']); return $conn; }
Now we can set the database connection configuration externally and pass it to the get_connection_config
function:
$config = ['host' => 'newhost', 'user' => 'newuser', ...]; $conn = get_connection_config($config);
This allows us to separate the database connection logic from the function itself, thus improving the flexibility, testability, and maintainability of the code.
The above is the detailed content of How does the parameter passing method of PHP functions affect function dependency injection?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
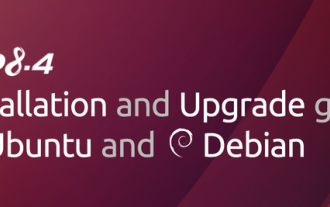
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
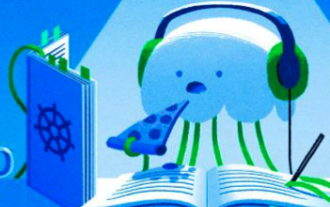
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c

One of the major changes introduced in MySQL 8.4 (the latest LTS release as of 2024) is that the "MySQL Native Password" plugin is no longer enabled by default. Further, MySQL 9.0 removes this plugin completely. This change affects PHP and other app
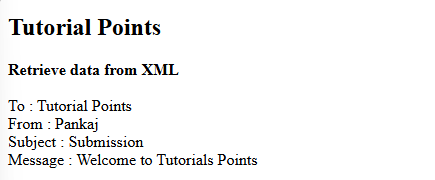
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
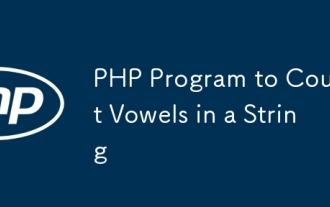
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
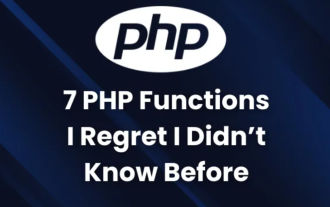
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
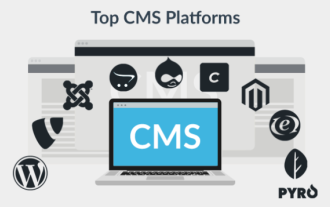
CMS stands for Content Management System. It is a software application or platform that enables users to create, manage, and modify digital content without requiring advanced technical knowledge. CMS allows users to easily create and organize content
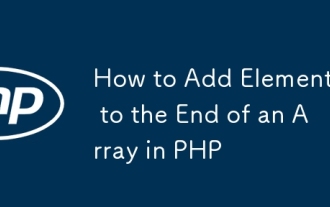
Arrays are linear data structures used to process data in programming. Sometimes when we are processing arrays we need to add new elements to the existing array. In this article, we will discuss several ways to add elements to the end of an array in PHP, with code examples, output, and time and space complexity analysis for each method. Here are the different ways to add elements to an array: Use square brackets [] In PHP, the way to add elements to the end of an array is to use square brackets []. This syntax only works in cases where we want to add only a single element. The following is the syntax: $array[] = value; Example
