Cooperation between Golang coroutine and channel
The cooperation of coroutines and Channels can realize concurrent programming and improve program performance and throughput. Through Channels, coroutines can communicate and exchange data safely and efficiently. The main steps are as follows: Create a Channel receiving task. Start multiple coroutines to receive and process tasks from Channel. Create a task on the main thread and send it to the Channel. Closing the Channel tells the coroutine that there are no more tasks. Use sync.WaitGroup to wait for all coroutines to complete.
The cooperation between Golang coroutine and Channel
Introduction
Coroutine is a user-mode lightweight thread, which is related to the process or Compared with threads, the creation and destruction of coroutines consumes fewer resources. Channel is a mechanism in the Go language for communication between goroutines. The combination of coroutines and channels enables concurrent programming, thereby improving program performance and throughput.
Practical Case
Let us use a practical case to demonstrate the cooperation between coroutine and channel. This case will show how to process a set of tasks in parallel.
// 任务定义 type Task struct { ID int Data []int } // 任务处理函数 func processTask(task *Task) { // 耗时处理 fmt.Printf("Processing task %d\n", task.ID) time.Sleep(time.Second * 2) } func main() { // 创建一个 channel 用于接收任务 tasks := make(chan *Task, 10) // 启动 4 个协程来处理任务 for i := 0; i < 4; i++ { go func() { for { // 从 channel 中接收任务 task := <-tasks // 处理任务 processTask(task) } }() } // 创建任务并将其发送到 channel for i := 0; i < 10; i++ { task := &Task{ ID: i, Data: []int{i, i + 1, i + 2}, } tasks <- task } // 关闭 channel 告知协程没有更多任务 close(tasks) // 等待所有协程完成 var wg sync.WaitGroup wg.Add(4) for i := 0; i < 4; i++ { go func() { // 协程退出时通知WaitGroup wg.Done() }() } wg.Wait() }
Code running process:
- Create a channel
tasks
for receiving tasks. - Start 4 coroutines, each coroutine receives tasks from the channel and processes them.
- Create 10 tasks in the main thread and send them to the channel.
- Close the channel to inform the coroutine that there are no more tasks.
- Use
sync.WaitGroup
to wait for all coroutines to complete.
Summary
The combination of coroutines and channels can achieve concurrent programming, thereby improving program performance and throughput. By using channels, coroutines can communicate and exchange data safely and efficiently. This is useful for handling large amounts of tasks or scenarios that require parallel processing.
The above is the detailed content of Cooperation between Golang coroutine and channel. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
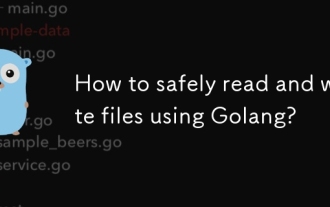
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
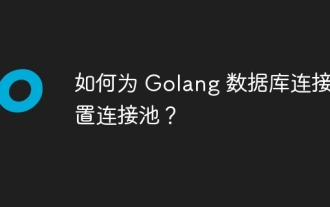
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
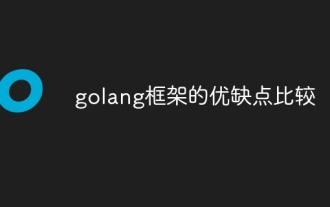
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
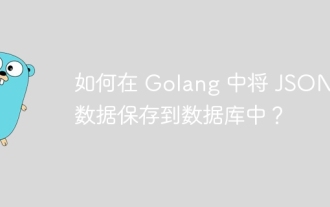
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
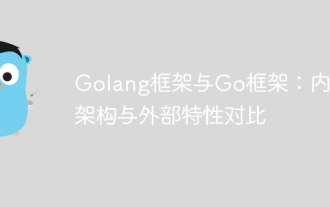
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
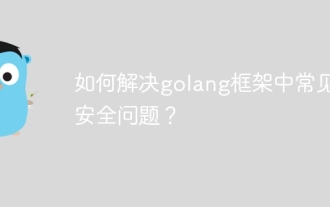
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
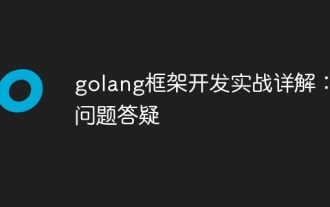
In Go framework development, common challenges and their solutions are: Error handling: Use the errors package for management, and use middleware to centrally handle errors. Authentication and authorization: Integrate third-party libraries and create custom middleware to check credentials. Concurrency processing: Use goroutines, mutexes, and channels to control resource access. Unit testing: Use gotest packages, mocks, and stubs for isolation, and code coverage tools to ensure sufficiency. Deployment and monitoring: Use Docker containers to package deployments, set up data backups, and track performance and errors with logging and monitoring tools.
