C++ function overloading and function virtual functions
Answer: Function overloading and function virtual functions in C allow developers to create functions with the same name but different parameter lists or behaviors. Detailed description: Function overloading: Create functions with the same name but different parameter lists to use functions with similar functionality in different situations. Function virtual function: Override the function of the base class in the derived class, used for polymorphism, allowing the derived class to provide a different implementation than the base class.
Function overloading and function virtual functions in C
Function overloading
Function overloading allows you to create multiple functions with the same name but different parameter lists. This is useful if you need to use functions with similar functionality in different situations.
Syntax:
ret_type function_name(parameter_list_1); ret_type function_name(parameter_list_2); ...
Example:
void printInfo(int x) { cout << "int: " << x << endl; } void printInfo(double x) { cout << "double: " << x << endl; } int main() { int a = 10; double b = 3.14; printInfo(a); // calls printInfo(int) printInfo(b); // calls printInfo(double) return 0; }
Function virtual function
Function Virtual functions allow you to override functions of a base class in a derived class. This is useful for polymorphism and object-oriented programming because it allows you to provide a derived class with a different implementation than the base class.
Syntax:
Use the keyword virtual
when declaring a function in a base class.
virtual ret_type function_name(parameter_list) const = 0;
Use the keyword override
when overriding a function in a derived class.
override ret_type function_name(parameter_list) const { /* implementation */ }
##Example:
class Shape { public: virtual double area() const = 0; // pure virtual function }; class Circle : public Shape { public: double radius; Circle(double radius) : radius(radius) {} override double area() const { return 3.14 * radius * radius; } }; class Square : public Shape { public: double side; Square(double side) : side(side) {} override double area() const { return side * side; } }; int main() { Shape* s1 = new Circle(5); Shape* s2 = new Square(10); cout << "Area of circle: " << s1->area() << endl; cout << "Area of square: " << s2->area() << endl; return 0; }
Note:
- Function overloading only applies to different parameter lists. You can't just change the return type.
- Function virtual functions only apply to virtual functions, that is, functions declared as
- virtual
in the base class.
Pure virtual functions (declared as - virtual
and
= 0in the base class) must be overridden in the derived class, otherwise the derived class will become an abstract class.
The above is the detailed content of C++ function overloading and function virtual functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

C++ object layout and memory alignment optimize memory usage efficiency: Object layout: data members are stored in the order of declaration, optimizing space utilization. Memory alignment: Data is aligned in memory to improve access speed. The alignas keyword specifies custom alignment, such as a 64-byte aligned CacheLine structure, to improve cache line access efficiency.
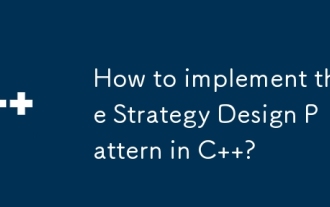
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
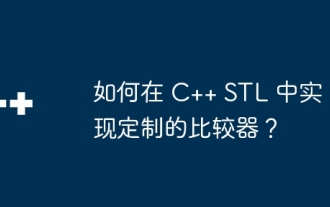
Implementing a custom comparator can be accomplished by creating a class that overloads operator(), which accepts two parameters and indicates the result of the comparison. For example, the StringLengthComparator class sorts strings by comparing their lengths: Create a class and overload operator(), returning a Boolean value indicating the comparison result. Using custom comparators for sorting in container algorithms. Custom comparators allow us to sort or compare data based on custom criteria, even if we need to use custom comparison criteria.
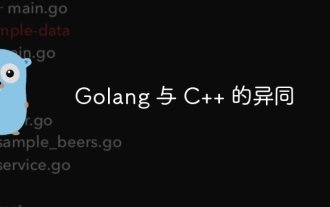
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
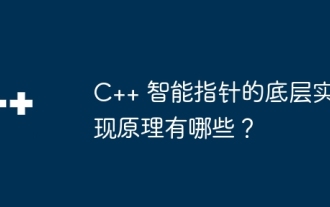
C++ smart pointers implement automatic memory management through pointer counting, destructors, and virtual function tables. The pointer count keeps track of the number of references, and when the number of references drops to 0, the destructor releases the original pointer. Virtual function tables enable polymorphism, allowing specific behaviors to be implemented for different types of smart pointers.
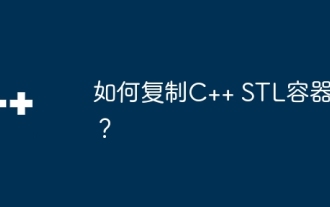
There are three ways to copy a C++ STL container: Use the copy constructor to copy the contents of the container to a new container. Use the assignment operator to copy the contents of the container to the target container. Use the std::copy algorithm to copy the elements in the container.
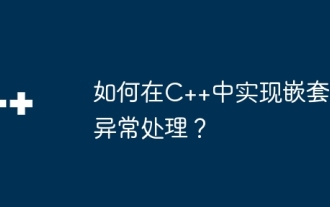
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
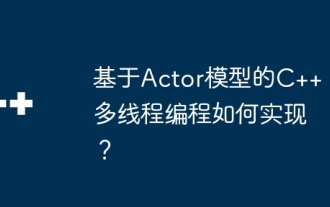
C++ multi-threaded programming implementation based on the Actor model: Create an Actor class that represents an independent entity. Set the message queue where messages are stored. Defines the method for an Actor to receive and process messages from the queue. Create Actor objects and start threads to run them. Send messages to Actors via the message queue. This approach provides high concurrency, scalability, and isolation, making it ideal for applications that need to handle large numbers of parallel tasks.
