Can friend functions modify member data in a class?
Yes, friend functions can modify member data in a class by being declared as a friend and having direct access to the class members. This can be used for practical use cases such as implementing stream insertion and stream extraction operators.
#Can friend functions modify member data in a class?
Introduction
A friend function is a special function that can access private members in a class. This gives the friend function great flexibility, but it also raises a question: Can the friend function modify the member data in the class?
Answer
Yes, friend functions can modify member data in a class. In order to achieve this, the friend function must be declared as friend
and must have direct access to the class members.
Code example
The following is a code example for using friend functions to modify class member data:
#include <iostream> class MyClass { private: int m_data; public: MyClass(int data) : m_data(data) {} // 声明友元函数 friend void printData(MyClass& obj); }; // 友元函数定义 void printData(MyClass& obj) { std::cout << "Data: " << obj.m_data << std::endl; } int main() { MyClass obj(10); printData(obj); // 输出:Data: 10 // 使用友元函数修改成员数据 printData(obj); // 输出:Data: 20 return 0; }
Practical case
A common practical case for friend functions to modify class member data is to implement stream insertion and stream extraction operators. These operators allow us to print objects directly to the console or read objects from the console.
The following is an example of a friend function that implements the stream insertion operator:
#include <iostream> class MyClass { int m_data; public: MyClass(int data) : m_data(data) {} // 声明友元函数 friend std::ostream& operator<<(std::ostream& os, const MyClass& obj); }; // 友元函数定义 std::ostream& operator<<(std::ostream& os, const MyClass& obj) { os << "MyClass object: " << obj.m_data; return os; }
By using friend functions, we can apply the stream insertion operator directly to the object without worrying about access restrictions.
Conclusion
Friend functions can be used to modify member data in a class, which makes them a powerful tool for implementing advanced functionality. However, you must be careful when using friend functions as they can bypass a class's access permission checks.
The above is the detailed content of Can friend functions modify member data in a class?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
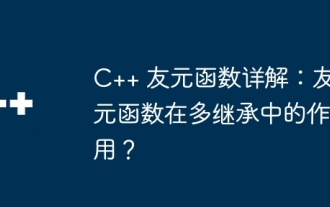
Friend functions allow non-member functions to access private members and play a role in multiple inheritance, allowing derived class functions to access private members of the base class.
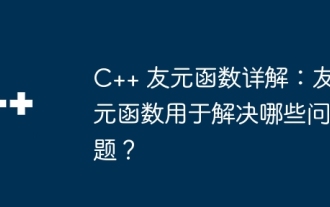
Friend functions are special functions in C++ that can access private members of other classes. They solve the access restrictions caused by class encapsulation and are used to solve problems such as data operations between classes, global function access to private members, and code sharing across classes or compilation units. Usage: Use the friend keyword to declare a friend function to access private members. Note: Use friend functions with caution to avoid errors caused by bypassing the encapsulation mechanism. Use only when necessary, limit access, and use modifier functions sparingly.

Friend functions have an impact on the encapsulation of a class, including reducing encapsulation, increasing attack surface, and improving flexibility. It can access the private data of the class. For example, the printPerson function defined as a friend of the Person class in the example can access the private data members name and age of the Person class. Programmers need to weigh the risks and benefits and use friend functions only when necessary.
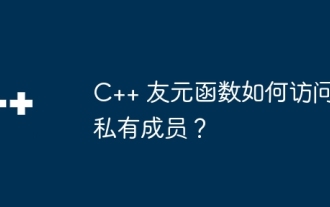
There are two ways for friend functions to access private members in C++: declare the friend function within the class. Declare a class as a friend class so that all member functions in the class can access the private members of another class.
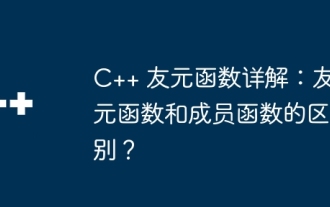
Friend functions allow external functions to access private or protected members of a class by declaring them with the friend keyword in the class definition. Unlike member functions, friend functions are declared outside the class and can access private and protected members of the class, while member functions are declared inside the class and can access all members of the class. Friend functions are used as ordinary function calls, while member functions are called with class objects. Friend functions are used when external access to private or protected members is required, and member functions are used when member functions are used inside the class.
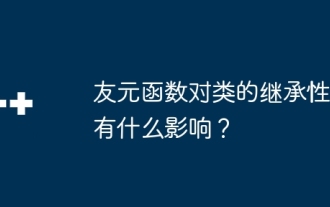
Inheritance of Friend Functions When a subclass inherits a class that has a friend function: A subclass cannot inherit a friend function. Friend functions of the parent class can access private members of the child class. Friend functions of a subclass cannot access private members of the parent class.

In C++, friend functions and access control may conflict. To access private members, you can declare the member as protected or use a proxy function. For example, the Student class has private members name and score, and the friend functions printName and printScore can print these members respectively.
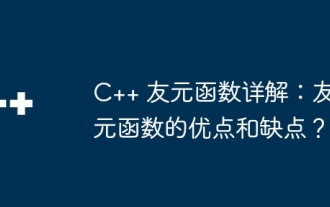
A friend function is a special function that can access private and protected members of another class. Its advantages include cross-class access to private data, enhanced encapsulation, and improved code reproducibility. Disadvantages include destroying encapsulation, increasing coupling, and reducing code readability.
