How to optimize Golang functional programs using lazy evaluation?
Lazy evaluation can be implemented in the Go language by using lazy data structures: create a wrapper type that encapsulates the actual value and only evaluates it when needed. Optimize the calculation of Fibonacci sequences in functional programs, deferring the calculation of intermediate values until actually needed. This can eliminate unnecessary overhead and improve the performance of functional programs.
How to use lazy evaluation to optimize Golang functional programs
Introduction
Lazy evaluation is a programming paradigm that allows deferring the evaluation of an expression until its result is actually used. This is useful in functional programming because it can optimize the execution of the program. This article will introduce how to implement lazy evaluation using Go language, and provide a practical case to demonstrate its role in optimization programs.
Lazy evaluation in Go
The Go language does not directly support lazy evaluation, but we can use lazy data structures to simulate its behavior. A common approach is to create a wrapper type that encapsulates the actual value and only evaluates it when needed.
Code Example
type Lazy[T any] struct { value T computed bool } func (l *Lazy[T]) Get() T { if !l.computed { l.value = calculateValue() l.computed = true } return l.value }
In this example, Lazy
is a generic type that represents a lazily computed value. When the Get()
method is called, it checks whether the value has already been calculated. If not, it calculates the value, stores it, and returns that value.
Practical Case
Consider a functional program using the Fibonacci sequence. The purpose of this program is to calculate the Fibonacci sequence for a given integer n. Normally, we would use a recursive function to solve this problem, but this would create a lot of intermediate calls, which would reduce efficiency.
Optimized code
func fibonacciLazy(n int) Lazy[int] { return Lazy[int]{ value: 0, computed: false, } } func (l Lazy[int]) Fibonacci(n int) Lazy[int] { if n <= 1 { return Lazy[int]{ value: n, computed: true, } } fibMinusOne := l.Fibonacci(n - 1).Get() fibMinusTwo := l.Fibonacci(n - 2).Get() return Lazy[int]{ value: fibMinusOne + fibMinusTwo, computed: true, } }
With lazy evaluation, we postpone the calculation of the intermediate values of the Fibonacci sequence until they are actually needed Just calculate. This eliminates unnecessary intermediate calls, resulting in a more efficient program.
Conclusion
By using lazy data structures, we can simulate lazy evaluation in the Go language. This allows us to optimize functional programs, eliminating unnecessary overhead by deferring the calculation of intermediate values. On problems like the Fibonacci sequence, this optimization can significantly improve the performance of your program.
The above is the detailed content of How to optimize Golang functional programs using lazy evaluation?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


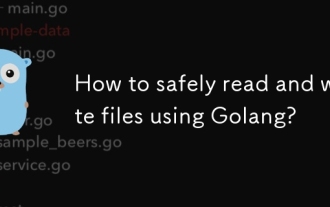
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
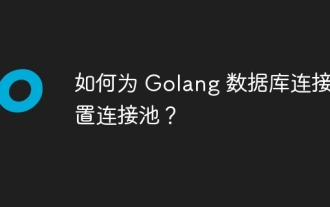
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
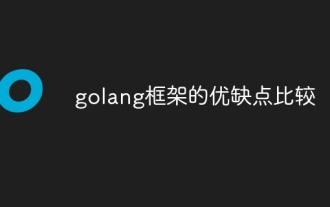
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
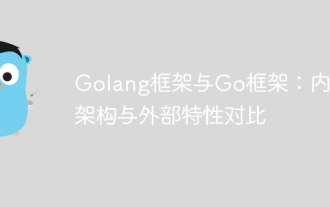
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
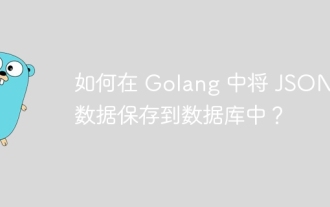
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
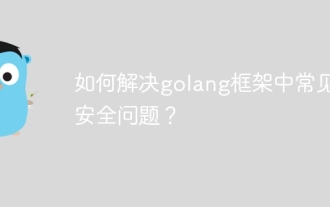
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
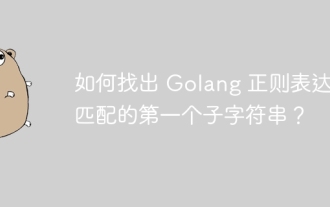
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
