How safe are C++ static functions in a multi-threaded environment?
Static functions may have thread safety issues in a multi-threaded environment. The reasons include concurrent access and damage to data integrity. The solution is to use mutex locks for synchronization protection, or use atomic operations or read-only data.
The safety of C static functions in a multi-threaded environment
Preface
In a multi-threaded environment, it is critical to understand how to use static functions safely. Static functions are functions that need to be instantiated only once, which means that only one copy of them exists within the scope of the program.
Thread safety issues
If a static function accesses or modifies shared data, it may be unsafe in a multi-threaded environment. The reasons are as follows:
- Concurrent access: Multiple threads can access static functions at the same time, resulting in data inconsistency.
- Violation of Data Integrity: One thread can modify static data while other threads may be using that data, thus compromising data integrity.
Solution
To ensure the safety of static functions in a multi-threaded environment, the following techniques can be used:
- Mutex lock: Mutex lock is used to prevent multiple threads from accessing shared resources at the same time. We can acquire the mutex before calling the static function and release it when done.
- Atomic operations: We can use atomic operations to update shared data to ensure that the operation is atomic, that is, completed once.
- Read-only data: If static data is read-only, it is safe in a multi-threaded environment.
Practical Case
The following is a practical case showing how to safely use static functions in a multi-threaded environment:
#include <mutex> using namespace std; class MyClass { public: static mutex m; static int shared_data; static void increment() { m.lock(); shared_data++; m.unlock(); } }; mutex MyClass::m; int MyClass::shared_data = 0; void thread_function() { for (int i = 0; i < 10000; i++) { MyClass::increment(); } } int main() { thread t1(thread_function); thread t2(thread_function); t1.join(); t2.join(); cout << "Shared data: " << MyClass::shared_data << endl; return 0; }
In this example:
-
increment
The function is static and it accesses shared datashared_data
. - We use a mutex lock (
m
) to prevent simultaneous access toshared_data
, thus ensuring thread safety. The value of -
shared_data
is eventually correctly updated to20000
(incremented 10000 times by both threads).
The above is the detailed content of How safe are C++ static functions in a multi-threaded environment?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
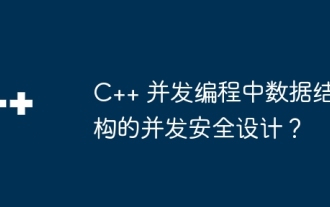
In C++ concurrent programming, the concurrency-safe design of data structures is crucial: Critical section: Use a mutex lock to create a code block that allows only one thread to execute at the same time. Read-write lock: allows multiple threads to read at the same time, but only one thread to write at the same time. Lock-free data structures: Use atomic operations to achieve concurrency safety without locks. Practical case: Thread-safe queue: Use critical sections to protect queue operations and achieve thread safety.

C++ object layout and memory alignment optimize memory usage efficiency: Object layout: data members are stored in the order of declaration, optimizing space utilization. Memory alignment: Data is aligned in memory to improve access speed. The alignas keyword specifies custom alignment, such as a 64-byte aligned CacheLine structure, to improve cache line access efficiency.
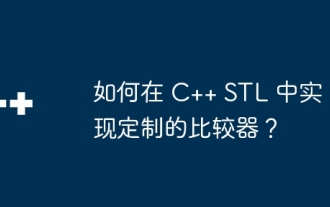
Implementing a custom comparator can be accomplished by creating a class that overloads operator(), which accepts two parameters and indicates the result of the comparison. For example, the StringLengthComparator class sorts strings by comparing their lengths: Create a class and overload operator(), returning a Boolean value indicating the comparison result. Using custom comparators for sorting in container algorithms. Custom comparators allow us to sort or compare data based on custom criteria, even if we need to use custom comparison criteria.
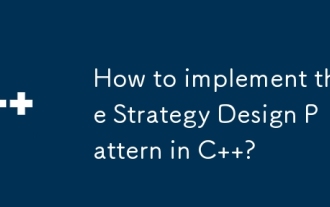
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
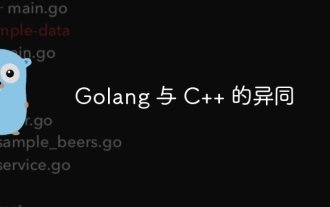
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
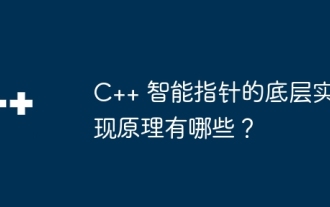
C++ smart pointers implement automatic memory management through pointer counting, destructors, and virtual function tables. The pointer count keeps track of the number of references, and when the number of references drops to 0, the destructor releases the original pointer. Virtual function tables enable polymorphism, allowing specific behaviors to be implemented for different types of smart pointers.
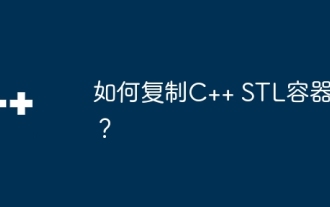
There are three ways to copy a C++ STL container: Use the copy constructor to copy the contents of the container to a new container. Use the assignment operator to copy the contents of the container to the target container. Use the std::copy algorithm to copy the elements in the container.
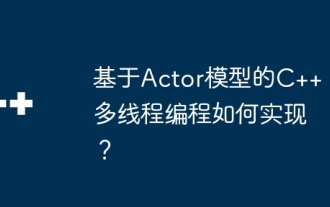
C++ multi-threaded programming implementation based on the Actor model: Create an Actor class that represents an independent entity. Set the message queue where messages are stored. Defines the method for an Actor to receive and process messages from the queue. Create Actor objects and start threads to run them. Send messages to Actors via the message queue. This approach provides high concurrency, scalability, and isolation, making it ideal for applications that need to handle large numbers of parallel tasks.
