Java Maven Build Tool: Extend and customize your build process
Maven is an extensible build tool that enables customization and expansion of the build process by creating plug-ins, extending the life cycle, using configuration files, and filtering resources. Specifically include: 1. Create a custom plug-in; 2. Extend the life cycle; 3. Use configuration files to override default behavior; 4. Perform resource filtering to modify the resource files used in the build; 5. Actual case: use custom plug-ins before compilation Perform code reviews.
Java Maven Build Tool: Extend and Customize Your Build Process
Maven is a popular Java build tool that provides a flexible and configurable Extensible way to manage your project builds. This article will guide you on how to extend and customize the Maven build process to meet your specific needs.
Extending Maven
Creating a plugin
To extend Maven, you can create your own plugin. A plugin is an XML file that contains build logic. To create a plugin:
- Create an XML file such as
my-plugin.xml
. - Add the following content in the XML file:
<plugin> <groupId>com.my-company</groupId> <artifactId>my-plugin</artifactId> <version>1.0</version> <extensions>true</extensions> <executions> <execution> <id>my-execution</id> <phase>compile</phase> <goals> <goal>my-goal</goal> </goals> </execution> </executions> </plugin>
Extend Maven's life cycle
Maven's life cycle is a predefined sequence of build phases. You can extend it by adding your own lifecycle stages. Add the following to your plugin:
<pluginManagement> <plugins> <plugin> <artifactId>maven-lifecycle-plugin</artifactId> <configuration> <lifecycleMappingMetadata> <lifecycle> <id>my-lifecycle</id> <phase>my-phase</phase> </lifecycle> </lifecycleMappingMetadata> </configuration> </plugin> </plugins> </pluginManagement>
Customizing Maven
Using configuration files
Configuration files allow you to override Maven's default behavior. To create a configuration file:
- Create an XML file such as
my-config.xml
. - Add the following content in the XML file:
<configuration> <my-setting>my-value</my-setting> </configuration>
Using resource filtering
Resource filtering allows you to modify the resource files used during the build process. To use resource filtering:
- Configure the resource filter in the
pom.xml
file:
<build> <resources> <resource> <directory>src/main/resources</directory> <filtering>true</filtering> </resource> </resources> </build>
- Use properties in the resource file :
${my-property}
Practical case
Case: Perform code review before compilation
Using a custom plug-in, you can execute code before compilation review.
- Create the plugin and add the following:
<goal>my-goal</goal> <configuration> <checkstyle-config>my-checkstyle-config.xml</checkstyle-config> </configuration>
- Create
my-checkstyle-config.xml
and add your Checkstyle configuration. - Configure the plugin in
pom.xml
:
<plugins> <plugin> <groupId>com.my-company</groupId> <artifactId>my-plugin</artifactId> <version>1.0</version> </plugin> </plugins>
Now, every time you run mvn compile
, it will execute the code first review.
The above is the detailed content of Java Maven Build Tool: Extend and customize your build process. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
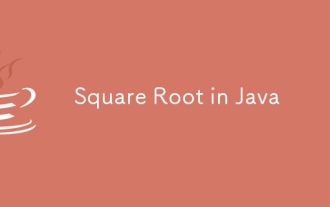
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
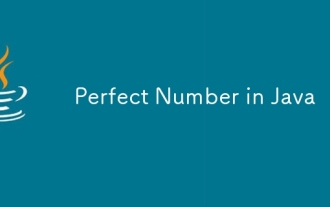
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
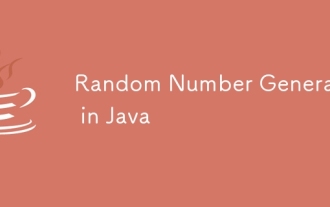
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
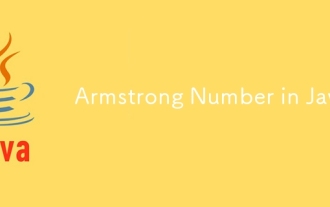
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
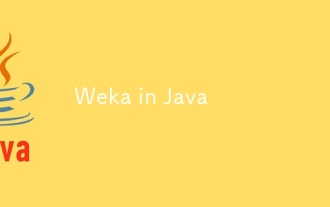
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
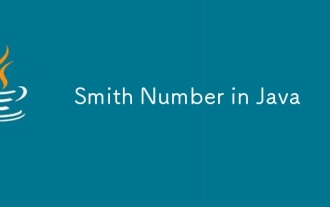
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
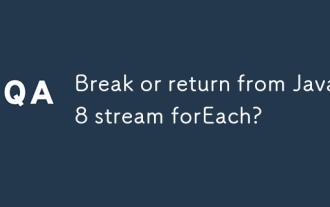
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
