


Data preprocessing techniques for Golang function performance optimization
In Golang function performance optimization, data preprocessing skills are crucial, including: caching commonly used data and avoiding I/O operations and calculations. Precompute derived values to save repeated calculations. Use slices to extend the length and avoid multiple allocations and copies.
Golang function performance optimization data preprocessing skills
To optimize function performance in Golang, data preprocessing skills are crucial . By preprocessing data, unnecessary overhead during function execution can be reduced, thereby improving execution efficiency.
1. Cache commonly used data
For frequently accessed data (such as configuration values, constants), caching it in memory can avoid frequent I/O operations and calculations. For example:
var cachedConfig *Config func GetConfig() *Config { if cachedConfig == nil { cachedConfig, err := LoadConfigFromFile("config.json") if err != nil { // 处理错误 } } return cachedConfig }
2. Precompute derived values
You can save repeated calculations in functions by precomputing derived values (such as hashes, converted values). For example:
var hashedPassword string func CheckPassword(password string, hashedPassword string) bool { if hashedPassword == "" { hashedPassword = Hash(password) } return hashedPassword == Hash(password) }
3. Use slices to extend the length
When it is predicted that the slice will continue to expand, use append(slice, ...) = nil
Extending the length of a slice avoids multiple allocations and copies. For example:
func AppendToSlice(slice []int, values ...int) { slice = append(slice, values...) // 扩展切片长度 _ = slice[:cap(slice)] // 清除未分配的元素 }
Practical case
The following is an actual optimization example of a function call:
// 不优化 func ProcessData(data [][]int) { for _, row := range data { for _, col := range row { // 对 col 进行计算 } } } // 优化 func ProcessData(data [][]int) { // 将 data 转换为 map,以列为键 cols := make(map[int][]int) for _, row := range data { for i, col := range row { cols[i] = append(cols[i], col) } } // 遍历列并进行计算 for col, values := range cols { // 对 values 进行计算 } }
After optimization, function By pre-fetching columns into a map, performance is improved by reducing the number of iterations over the original data.
The above is the detailed content of Data preprocessing techniques for Golang function performance optimization. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


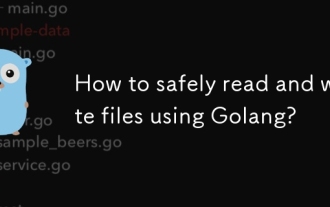
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
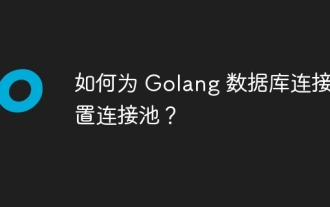
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
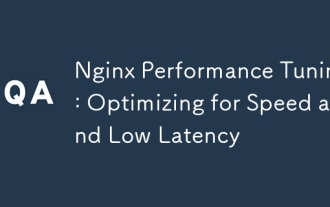
Nginx performance tuning can be achieved by adjusting the number of worker processes, connection pool size, enabling Gzip compression and HTTP/2 protocols, and using cache and load balancing. 1. Adjust the number of worker processes and connection pool size: worker_processesauto; events{worker_connections1024;}. 2. Enable Gzip compression and HTTP/2 protocol: http{gzipon;server{listen443sslhttp2;}}. 3. Use cache optimization: http{proxy_cache_path/path/to/cachelevels=1:2k
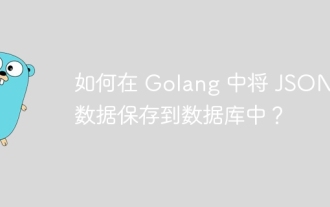
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
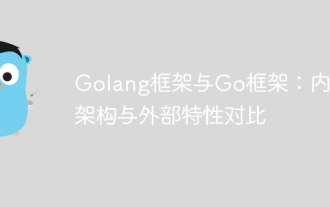
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
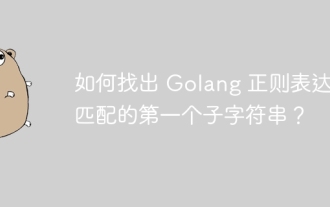
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
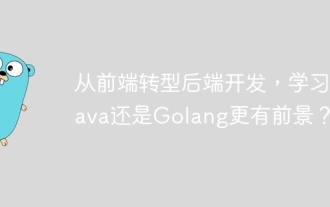
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
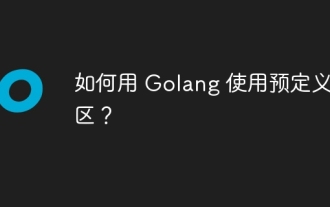
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
