Security considerations for Golang function libraries
When using Go function libraries, you need to consider the following security considerations: Update dependencies regularly to ensure there are no known vulnerabilities. Validate and sanitize user input to prevent injection attacks. Use proven encryption algorithms to handle sensitive data. Handle errors raised by the function library and take appropriate action. Follow best practices, such as providing a maximum number of splits when using the strings.Split function.
Security Precautions for Go Function Library
Go is a popular programming language that provides a rich set of functions Libraries that help developers build applications quickly and efficiently. However, as with any software component, there are security implications to consider when using Go libraries.
Security Practice
-
Audit dependencies: Use the
go mod tidy
command to regularly update and audit dependencies versions of the items to ensure they are up to date and have no known vulnerabilities. -
Check input: Always validate and sanitize user input. Use packages such as
regexp
andstrconv
to check formats, andhtml/template
to escape input to prevent injection attacks. -
Use encryption algorithms: When dealing with sensitive data (such as passwords), use a proven encryption algorithm such as
crypto/cipher
. Avoid using homemade encryption algorithms. -
Handling errors: Handle errors that may be caused by the function library. Use the
error
interface and theif err != nil
statement to check for errors and take appropriate action. -
Follow best practices: Follow best practices from the Go community, such as providing a maximum number of splits when using the
strings.Split
function.
Practical case
Consider the following code using the os/exec
function library:
package main import ( "fmt" "os/exec" ) func main() { cmd := exec.Command("/bin/bash", "-c", "echo hello world") output, err := cmd.CombinedOutput() if err != nil { fmt.Printf("Failed to execute command: %s", err) return } fmt.Println(string(output)) }
Although this paragraph The code attempts to execute a simple shell command to print "hello world", but it has several security risks:
- Injection attack: If the user input is not validated, the attack The attacker can inject malicious commands.
-
Elevated Privilege: Without appropriate restrictions,
os/exec
can allow an attacker to execute commands with elevated privileges.
Security Recommendations
To mitigate these risks, take the following steps:
-
Restrict user input: Use
strings.TrimSpace
andregexp
to limit the characters allowed to be entered. -
Restrict command permissions: Use
exec.CommandContext
and set the appropriate permissions to limit the permissions for command execution.
By following these security practices and best practices, you can minimize security risks when using Go function libraries and ensure the security and reliability of your applications.
The above is the detailed content of Security considerations for Golang function libraries. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


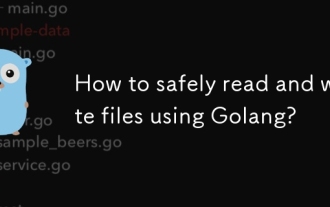
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
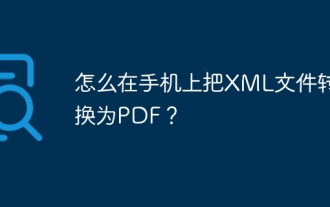
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
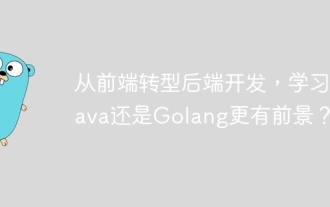
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
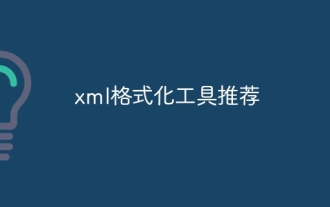
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
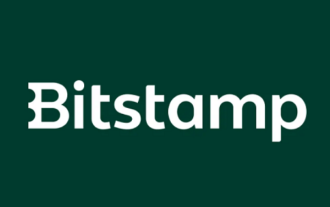
How to register BitstampPro? Visit the BitstampPro website. Fill in your personal information and email address. Create a password and accept the terms. Verify email address. Is BitstampPro safe? Authentication required. Enforce the use of two-factor authentication. Most assets are stored in cold storage. Use HTTPS to encrypt communication. Conduct regular security audits. Is BitstampPro legitimate? Registered in Luxembourg. Regulated by the Luxembourg Financial Supervisory Committee. Comply with anti-money laundering and know-your-customer regulations.
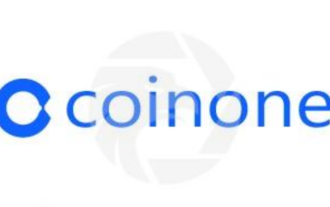
Coinone is a formal cryptocurrency trading platform founded in 2014 and is one of the leading trading platforms in South Korea. It is known for its transparency, security, reliability, and wide selection of digital assets. Coinone complies with Korean government regulations and provides transparent fees and clear transaction information. It uses industry-leading security measures, including 2FA, cold storage, and DDoS protection. Coinone has strong liquidity, ensures fast transactions, and provides over-the-counter trading and a user-friendly interface. But it is mainly targeted at the Korean market and transaction fees may be slightly higher.
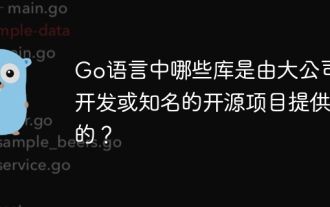
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
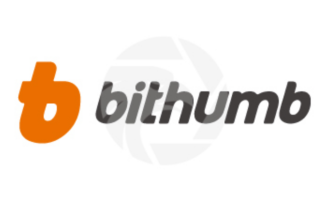
Bithumb is South Korea’s largest cryptocurrency exchange, offering: A wide selection of over 360 currencies. High liquidity, ensuring fast transactions and reasonable fees. Security measures include the use of cold wallet storage, two-factor authentication and anti-money laundering measures. However, Bithumb has faced money laundering accusations and its regulatory environment is uncertain. It's important to weigh the pros and cons and do your own research before using this exchange.
