


What are the best practices for improving execution efficiency of C++ functions?
In order to improve the execution efficiency of C functions, best practices include: reducing unnecessary function calls; inlining small functions; optimizing loops (using range loops, register keywords, and avoiding function calls in loops); avoiding dynamic allocation ( Use memory pools and preallocated containers); use constant expressions.
Best practices for improving C function execution efficiency
In large and complex projects, the execution efficiency of functions is crucial. The following best practices can significantly improve the performance of C functions:
1. Reduce the number of function calls
- Try to avoid unnecessary function calls because they There will be overhead.
- Consider inlining small functions or moving function calls outside the loop.
2. Inline functions
- Use the
inline
keyword to inline small functions, which can eliminate the need for function calls overhead. - Only inline simple functions that are called frequently, as inlining increases code size.
3. Optimize loops
- Use range loops (
for (auto& element : container)
) instead of iterator. - Use the
register
keyword to store local variables in a register for faster access. - Avoid making function calls in loops.
4. Avoid dynamic allocation
- Dynamic allocation (using
new
anddelete
) will Incur overhead. - Consider using memory pools and pre-allocated containers to reduce dynamic allocation.
5. Use constant expressions
- to mark the expression as
constexpr
to enable the compiler to calculate value, thereby eliminating runtime overhead. - Use constant variables instead of calculating the value each time.
Practical case: Optimizing Fibonacci sequence function
Consider the following unoptimized Fibonacci sequence function:
1 2 3 4 5 6 7 |
|
By applying the above best practices, we can greatly improve its efficiency:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
In the optimized function, we:
- utilize the constant cache to store the calculated value.
- Use register variables to optimize loop performance.
- Reduced unnecessary recursive calls.
Through these optimizations, the execution efficiency of the function is significantly improved, especially when entering large n
values.
The above is the detailed content of What are the best practices for improving execution efficiency of C++ functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
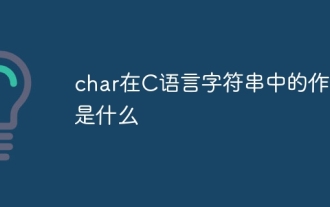
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
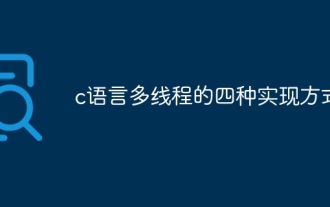
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
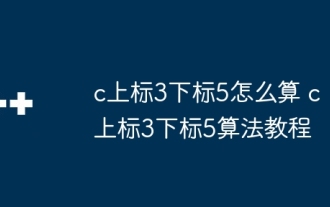
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
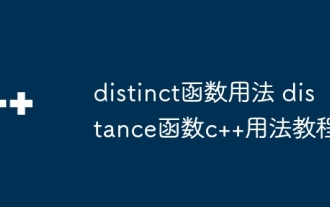
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
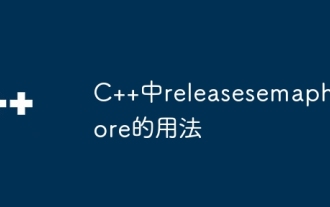
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
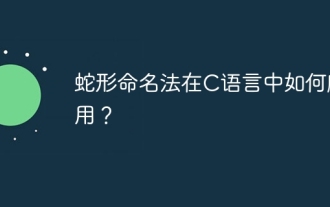
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
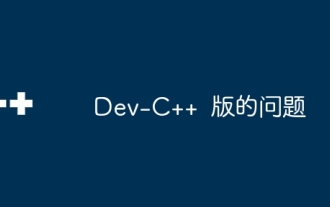
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
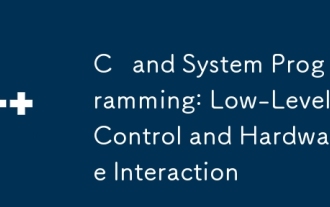
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
