Best practices and design patterns for Golang function libraries
Go function library design best practices include: modularization, clear interfaces, clear documentation, error handling, and careful use of type assertions. Commonly used design patterns include: singleton pattern (ensuring a single instance), factory pattern (creating object variants), and dependency injection (decoupling components). Following these principles can create a library that is modular, maintainable, extensible, and less error-prone.
Best practices and design patterns for Golang function libraries
In Go programming, a function library is a way to group related functions and provide a consistent interface Powerful tool. Use best practices and design patterns to create libraries that are modular, maintainable, and extensible.
Best Practices:
- Keep modular: Organize related functions into subpackages to improve code readability and manageability.
- Define clear interfaces: Use interface types to define the public API of the function library, allowing users to rely on the function library without knowing the implementation details.
-
Provide documentation: Use
//
comments or Go docstrings (///
) to provide documentation to help developers understand the functions Purpose, parameters and return values. - Handling errors: When possible, return an explicit error object with a detailed error message.
- Use type assertions: Use type assertions with caution as they may cause runtime errors.
Design pattern:
Singleton pattern:
- Ensure that there is only one instance of a specific object in the function library.
- Avoid resource waste and object conflicts.
-
Example:
package main import "sync" type Single struct { sync.Mutex instance *Single } func (s *Single) Instance() *Single { s.Lock() defer s.Unlock() if s.instance == nil { s.instance = &Single{} } return s.instance } func main() { instance1 := Single{}.Instance() instance2 := Single{}.Instance() fmt.Println(instance1 == instance2) // true }
Copy after login
Factory pattern:
- Create objects Multiple variants without exposing the creation logic to the client.
- Improve code flexibility, allowing new variants to be added without changing client code.
Example:
package main type Animal interface { GetName() string } type Cat struct { name string } func (c *Cat) GetName() string { return c.name } type Dog struct { name string } func (d *Dog) GetName() string { return d.name } type AnimalFactory struct {} func (f *AnimalFactory) CreateAnimal(animalType string, name string) Animal { switch animalType { case "cat": return &Cat{name} case "dog": return &Dog{name} default: return nil } } func main() { factory := &AnimalFactory{} cat := factory.CreateAnimal("cat", "Meow") dog := factory.CreateAnimal("dog", "Buddy") fmt.Println(cat.GetName()) // Meow fmt.Println(dog.GetName()) // Buddy }
Copy after login
Dependency Injection:
- Through functions Constructors or interfaces provide dependencies to objects.
- Decouple components to improve testability and maintainability.
Example:
package main import "fmt" type Logger interface { Log(msg string) } type Database interface { Get(id int) interface{} } type Service struct { Logger Database } func NewService(logger Logger, db Database) *Service { return &Service{logger, db} } func main() { logger := ConsoleLogger{} db := MockDatabase{} service := NewService(logger, db) service.Log("Hello world") fmt.Println(service.Get(1)) } type ConsoleLogger struct {} func (ConsoleLogger) Log(msg string) { fmt.Println(msg) } type MockDatabase struct {} func (MockDatabase) Get(id int) interface{} { return id }
Copy after login
By following these best practices and adopting design patterns, you can create powerful, scalable Golang function library makes code easier to maintain, more flexible, and reduces errors.
The above is the detailed content of Best practices and design patterns for Golang function libraries. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
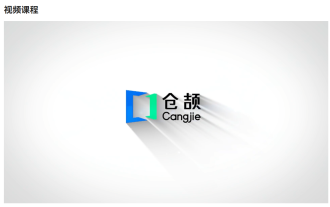
According to news from this site on June 24, at the keynote speech of the HDC2024 Huawei Developer Conference on June 21, Gong Ti, President of Huawei Terminal BG Software Department, officially announced Huawei’s self-developed Cangjie programming language. This language has been developed for 5 years and is now available for developer preview. Huawei's official developer website has now launched the official introductory tutorial video of Cangjie programming language to facilitate developers to get started and understand it. This tutorial will take users to experience Cangjie, learn Cangjie, and apply Cangjie, including using Cangjie language to estimate pi, calculate the stem and branch rules for each month of 2024, see N ways of expressing binary trees in Cangjie language, and use enumeration types to implement Algebraic calculations, signal system simulation using interfaces and extensions, and new syntax using Cangjie macros, etc. This site has tutorial access address: ht
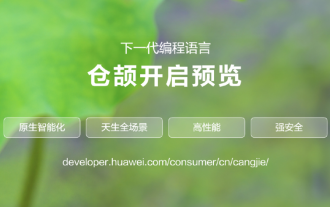
This site reported on June 21 that at the HDC2024 Huawei Developer Conference this afternoon, Gong Ti, President of Huawei Terminal BG Software Department, officially announced Huawei’s self-developed Cangjie programming language and released a developer preview version of HarmonyOSNEXT Cangjie language. This is the first time Huawei has publicly released the Cangjie programming language. Gong Ti said: "In 2019, the Cangjie programming language project was born at Huawei. After 5 years of R&D accumulation and heavy R&D investment, it finally meets global developers today. Cangjie programming language integrates modern language features, comprehensive compilation optimization and Runtime implementation and out-of-the-box IDE tool chain support create a friendly development experience and excellent program performance for developers. "According to reports, Cangjie programming language is an all-scenario intelligence tool.
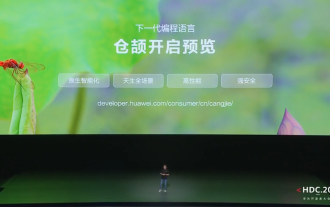
According to news from this site on June 21, Huawei’s self-developed Cangjie programming language was officially unveiled today, and the official announced the launch of HarmonyOSNEXT Cangjie language developer preview version Beta recruitment. This upgrade is an early adopter upgrade to the developer preview version, which provides Cangjie language SDK, developer guides and related DevEcoStudio plug-ins for developers to use Cangjie language to develop, debug and run HarmonyOSNext applications. Registration period: June 21, 2024 - October 21, 2024 Application requirements: This HarmonyOSNEXT Cangjie Language Developer Preview Beta recruitment event is only open to the following developers: 1) Real names have been completed in the Huawei Developer Alliance Certification; 2) Complete H
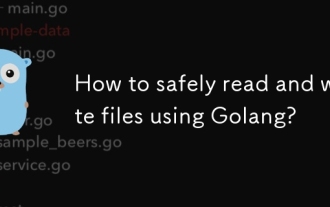
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
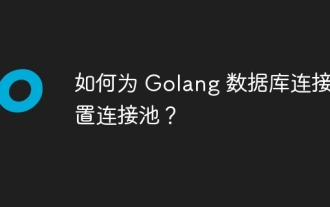
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
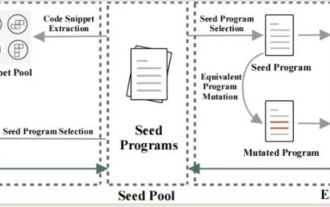
According to news from this site on June 22, Huawei yesterday introduced Huawei’s self-developed programming language-Cangjie to developers around the world. This is the first public appearance of Cangjie programming language. According to inquiries on this site, Tianjin University and Beijing University of Aeronautics and Astronautics were deeply involved in the research and development of Huawei’s “Cangjie”. Tianjin University: Cangjie Programming Language Compiler The software engineering team of the Department of Intelligence and Computing of Tianjin University joined hands with the Huawei Cangjie team to deeply participate in the quality assurance research of the Cangjie programming language compiler. According to reports, the Cangjie compiler is the basic software that is symbiotic with the Cangjie programming language. In the preparatory stage of the Cangjie programming language, a high-quality compiler that matches it became one of the core goals. As the Cangjie programming language evolves, the Cangjie compiler is constantly being upgraded and improved. In the past five years, Tianjin University
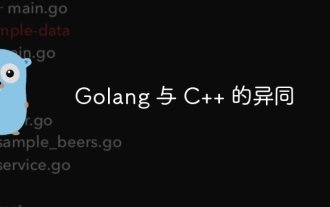
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
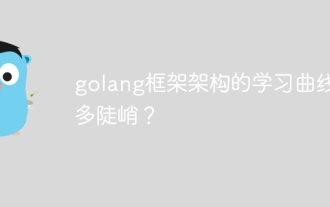
The learning curve of the Go framework architecture depends on familiarity with the Go language and back-end development and the complexity of the chosen framework: a good understanding of the basics of the Go language. It helps to have backend development experience. Frameworks that differ in complexity lead to differences in learning curves.
