The advantages of Go functions include: first-class citizens, which can be passed, returned, and stored; declarative syntax, concise and easy to read; parallelism, supporting concurrent programming; closures, realizing state preservation. Disadvantages include: forced typing, which can lead to redundant code; naming convention restrictions; no generics, unable to create generic functions; limited function overloading support.
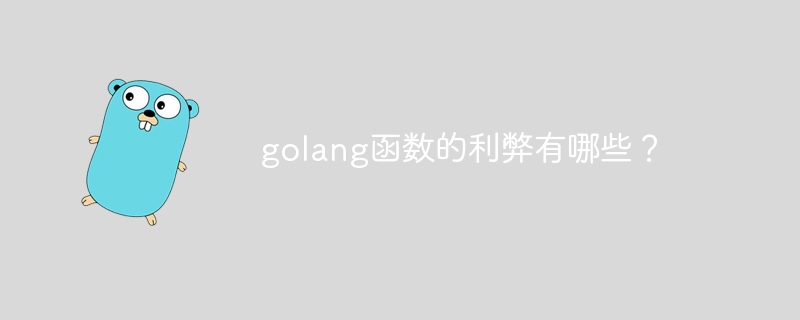
Pros and cons of Go functions
In the Go language, functions are the basic unit of code organization and reuse. They allow you to break down complex tasks into smaller manageable parts, making your code more readable and maintainable. Go functions have unique advantages and disadvantages compared to other languages.
Advantages:
-
First-class citizens: Go functions are first-class citizens, which means they can act like other types of values Same as passing, returning and storing in data structures. This provides great flexibility, allowing you to create high-level abstractions and dynamic code.
-
Declarative syntax: Function syntax in Go is concise and clear. A function signature clearly specifies the function's input parameters and return value types. This simplicity makes the code easy to read and understand.
-
Parallelism: The Go language supports concurrent programming with lightweight goroutine and channel mechanisms. Functions can run concurrently in different goroutines, taking full advantage of multi-core processors.
-
Closure: Go language supports closure, that is, nested functions can access variables of their outer functions. This allows you to create state-saving functions, simplifying the implementation of complex code.
Disadvantages:
-
Forced typing: Go is a strongly typed language, which means that the input of functions Parameter and return value types must be specified explicitly. While this provides type safety, it can also lead to redundant code, especially when dealing with complex data types.
-
Naming convention: The Go language has strict conventions on the naming of functions. Function names must begin with a lowercase letter and subsequent words with an uppercase letter, which may be inconsistent with conventions in other languages.
-
No Generics: Go does not currently support generics, which means you cannot create generic functions that work with different types of arguments. However, generics can be simulated by using interface types.
-
Limited function overloading: The Go language allows overloading functions with different parameter types, but it does not support different number or order of parameters for the same name function.
Practical case:
The following is a practical case of a Go function, demonstrating the flexibility of the function:
package main
import "fmt"
func main() {
// 定义一个接受任意数量整数参数并计算总和的函数
sum := func(args ...int) int {
total := 0
for _, num := range args {
total += num
}
return total
}
// 调用函数传递不同的参数列表
fmt.Println(sum(1, 2, 3)) // 输出:6
fmt.Println(sum(10, 20, 30)) // 输出:60
}
Copy after login
This function uses Variable length argument lists (...int) and the ability to return integers to create flexible and reusable code. It can perform various summing operations by passing different parameter lists.
The above is the detailed content of What are the pros and cons of golang functions?. For more information, please follow other related articles on the PHP Chinese website!