How to get the input number in java
There are two ways to get input numbers in Java: use the Scanner class and call the nextInt() method to get the integer. Using the BufferedReader class, call the readLine() method to get a line of text and convert it to a number.
How to get the input number using Java
Use Scanner class
## The #Scanner class is a commonly used class in Java for getting input from the console. It provides a nextInt() method to get the integer entered by the user.import java.util.Scanner; public class InputNumber { public static void main(String[] args) { // 创建一个 Scanner 对象 Scanner scanner = new Scanner(System.in); // 获取用户输入的数字 System.out.print("请输入一个数字:"); int number = scanner.nextInt(); // 打印用户输入的数字 System.out.println("你输入的数字是:" + number); // 关闭 Scanner 对象 scanner.close(); } }
Using the BufferedReader class
The BufferedReader class can also be used to get input from the console. It provides a readLine() method to obtain a line of text entered by the user. We can use this text to parse numbers entered by the user.import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; public class InputNumber { public static void main(String[] args) throws IOException { // 创建一个 BufferedReader 对象 BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); // 获取用户输入的数字 System.out.print("请输入一个数字:"); String input = reader.readLine(); // 解析用户输入的数字 int number = Integer.parseInt(input); // 打印用户输入的数字 System.out.println("你输入的数字是:" + number); // 关闭 BufferedReader 对象 reader.close(); } }
The above is the detailed content of How to get the input number in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


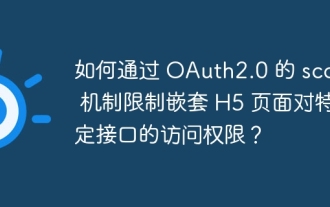
How to use OAuth2.0's access_token to achieve control of interface access permissions? In the application of OAuth2.0, how to ensure that the...
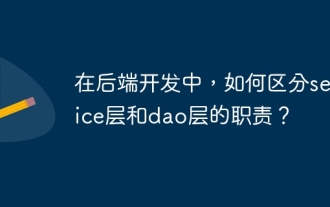
Discussing the hierarchical architecture in back-end development. In back-end development, hierarchical architecture is a common design pattern, usually including controller, service and dao three layers...
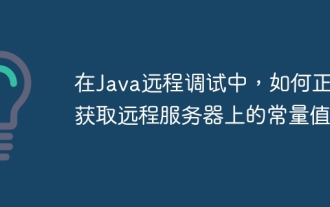
Questions and Answers about constant acquisition in Java Remote Debugging When using Java for remote debugging, many developers may encounter some difficult phenomena. It...
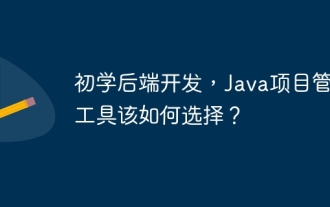
Confused with choosing Java project management tools for beginners. For those who are just beginning to learn backend development, choosing the right project management tools is crucial...
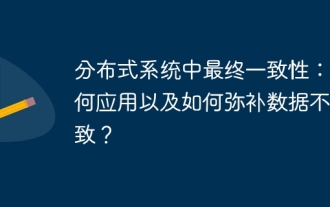
Exploring the application of ultimate consistency in distributed systems Distributed transaction processing has always been a problem in distributed system architecture. To solve the problem...
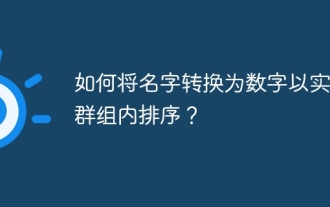
How to convert names to numbers to implement sorting within groups? When sorting users in groups, it is often necessary to convert the user's name into numbers so that it can be different...
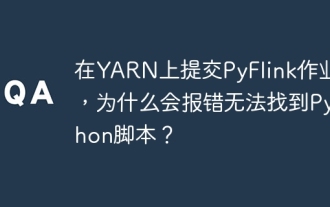
Analysis of the reason why Python script cannot be found when submitting a PyFlink job on YARN When you try to submit a PyFlink job through YARN, you may encounter...
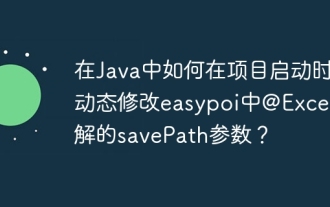
How to dynamically configure the parameters of entity class annotations in Java During the development process, we often encounter the need to dynamically configure the annotation parameters according to different environments...
