How to implement multi-threading in nodejs
Although Node.js is single-threaded, it can simulate multi-threading in many ways: 1. Worker thread: independently create threads to perform tasks; 2. Cluster module: create multiple worker processes for parallel processing; 3. Event loop : Arrange tasks into the event loop for non-blocking execution.
How to implement multi-threading in Node.js?
Node.js is a single-threaded running environment, which means it can only handle one task at a time. However, multi-threading can be simulated by:
1. Worker threads
Node.js 10.5 and later introduces Worker threads, which allow the creation of independent thread to perform time-consuming tasks, thereby releasing the main thread. Worker threads have the following advantages:
- Isolated from the main thread, so they do not block the main thread.
- Can communicate with the main thread through channels.
- Using shared memory by default can improve performance.
2. Cluster module
The Cluster module allows the creation of multiple worker processes, each with its own event loop. The main process is responsible for assigning tasks to worker processes, and the worker processes are responsible for processing tasks. The Cluster module has the following advantages:
- Execute tasks in parallel across processes.
- Improve scalability and throughput.
- Can automatically restart failed worker processes.
3. Event loop
The operation of Node.js is based on the event loop, which is a continuously running loop that constantly checks whether there are any pending tasks. Tasks can be I/O operations, timers, or user code. Non-blocking execution is achieved by scheduling time-consuming tasks into the event loop, freeing up the main thread.
Specific implementation
Use Worker thread:
// 创建一个 worker 线程 const worker = new Worker('./worker.js'); // 监听 worker 线程的消息 worker.addEventListener('message', (event) => { console.log('Received message from worker:', event.data); }); // 向 worker 线程发送消息 worker.postMessage({ message: 'Hello from main thread!' });
Use Cluster module:
// 创建一个 cluster 监听器 const cluster = require('cluster'); // 如果是主进程,则创建 worker 进程 if (cluster.isMaster) { for (let i = 0; i < numCPUs; i++) { cluster.fork(); } } else { // 如果是 worker 进程,则执行任务 console.log(`Worker ${cluster.worker.id} started`); performTask(); }
Use event loop:
// 安排一个耗时任务到事件循环 setTimeout(() => { console.log('耗时任务完成'); }, 1000);
The above is the detailed content of How to implement multi-threading in nodejs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


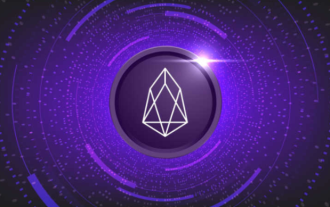
Yuzi Coin is a cryptocurrency based on blockchain technology with the following characteristics: Consensus mechanism: PoS Proof of Stake High scalability: Processing 10,000 transactions per second Low transaction fees: A few cents Support for smart contracts
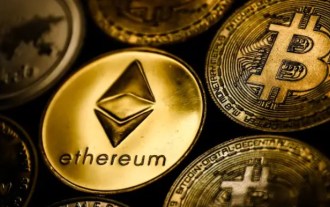
The upgrade of Ethereum has had a profound impact on the Layer 2 ecosystem, which is mainly reflected in four aspects: First, the upgrade improves the scalability and performance of Layer 2, meets the growing transaction needs, and promotes innovation in technologies such as zk-Rollup; Second, the upgrade enhances the security of Layer 2, and reduces risks by sharing the security mechanism of the Ethereum main network and promoting the integration of security technologies; Third, the upgrade improves the interoperability of Layer 2, optimizes cross-layer communication, and promotes collaboration between different Layer 2 solutions; Finally, the upgrade reduces the development cost and difficulty of Layer 2, provides a more friendly development environment, and promotes open source and sharing. In short, Ethereum upgrade
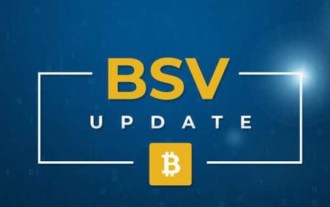
The future prospects of BSV coins are uncertain, affected by factors such as community support, legal proceedings and technological advantages, but there are also negative factors such as controversy, competition and regulatory uncertainty. Analysts are divided on predicting its price trend in 2024, with some predicting a sharp increase and others predicting it will remain stable or decline. Investors should carefully consider these factors before making investment decisions.
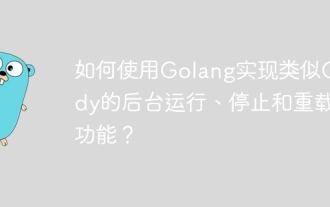
How to implement background running, stopping and reloading functions in Golang? During the programming process, we often need to implement background operation and stop...

Solanacoin is a blockchain-based cryptocurrency focused on delivering high performance and scalability. Its advantages include: high scalability, low transaction costs, fast confirmation times, a strong developer ecosystem and compatibility with the Ethereum Virtual Machine. But it also suffers from network congestion, relative newness and fierce competition. Whether or not to hold Solana depends on your personal risk tolerance and investment goals.
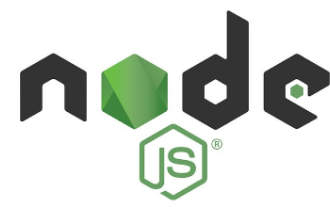
Node is an environment in which you can run JavaScript code "Outside the web browser". Node be like – "Hey y'all, you give your JS code to me and I'll run it ". It uses Google's V8 Engine to convert the JavaScript code to Machine Code. Since Node runs JavaScript code outside the web browser, this means that it doesn't have access to certain features that are only available in the browser, like the DOM or the window object or even the localStorage.
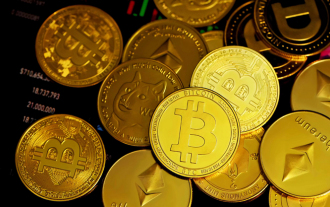
Ethereum’s price has gone through a roller coaster ride since its launch in 2015. It started at $0.31 and soared to $413 in the 2017 bubble. It then plummeted to $89 in 2018, but rebounded to $750 due to the rise of DeFi in 2020. It peaked at $4,891 in 2021, but then reversed in 2022, falling to $922. Rebounded to over $1,600 in 2023, and is affected in the future by potential growth factors such as Ethereum 2.0 upgrade, DeFi growth and enterprise adoption.
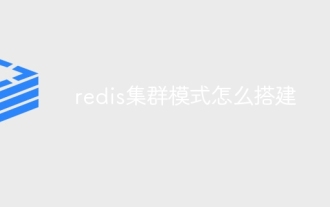
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
