How nodejs interacts with the front end
Node.js interacts with the frontend via HTTP request/response, WebSocket, and Socket.IO: Set up a Node.js server and define routes. The front end sends HTTP requests or establishes connections using WebSocket or Socket.IO. The Node.js server handles the request and returns a response or sends data over a live connection.
Node.js Interaction with the front-end
Node.js is a method for building server-side applications JavaScript runtime environment. It can interact with front-end technologies such as HTML, CSS, and JavaScript to provide dynamic and interactive web applications.
Interaction methods
Interaction between Node.js and the front-end can be carried out in the following ways:
- HTTP request/response: Node.js servers can handle HTTP requests from browsers or other clients and return HTML, JSON, or other types of responses.
- WebSocket: WebSocket is a two-way real-time communication protocol that allows a Node.js server to establish a persistent connection with a front-end for real-time transfer of data.
- Socket.IO: Socket.IO is a WebSocket library that simplifies real-time communication between Node.js and frontends. It also provides advanced features such as event handling and message namespaces.
Implementation steps
1. Create server:
const express = require('express'); const app = express(); const server = app.listen(3000);
2. Define route:
app.get('/', (req, res) => { res.send('Hello from Node.js!'); });
3. Handle front-end requests:
app.post('/submit-form', (req, res) => { const data = req.body; // 处理表单数据... });
4. Use WebSocket:
const WebSocket = require('ws'); const wss = new WebSocket.Server({ server }); wss.on('connection', (ws) => { // 与客户端建立 WebSocket 连接... });
5 . Using Socket.IO:
const socketIO = require('socket.io'); const io = socketIO(server); io.on('connection', (socket) => { // 与客户端建立 Socket.IO 连接... });
Front-end code example:
// 发送 HTTP 请求 fetch('/submit-form', { method: 'POST', body: JSON.stringify({ name: 'John' }), }) .then((res) => res.json()) .then((data) => console.log(data)); // 建立 WebSocket 连接 const socket = new WebSocket('ws://localhost:3000'); socket.onopen = () => console.log('Connected to WebSocket'); // 使用 Socket.IO const socket = io(); socket.on('connect', () => console.log('Connected to Socket.IO'));
The above is the detailed content of How nodejs interacts with the front end. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
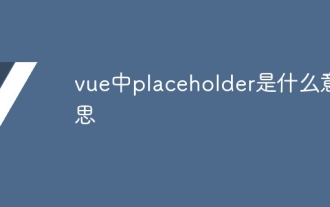
In Vue.js, the placeholder attribute specifies the placeholder text of the input element, which is displayed when the user has not entered content, provides input tips or examples, and improves form accessibility. Its usage is to set the placeholder attribute on the input element and customize the appearance using CSS. Best practices include being relevant to the input, being short and clear, avoiding default text, and considering accessibility.
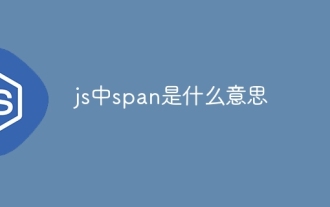
The span tag can add styles, attributes, or behaviors to text. It is used to: add styles, such as color and font size. Set attributes such as id, class, etc. Associated behaviors such as clicks, hovers, etc. Mark text for further processing or citation.
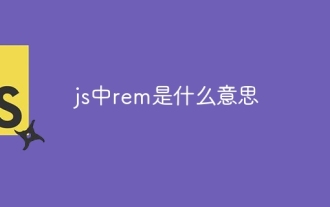
REM in CSS is a relative unit relative to the font size of the root element (html). It has the following characteristics: relative to the root element font size, not affected by the parent element. When the root element's font size changes, elements using REM will adjust accordingly. Can be used with any CSS property. Advantages of using REM include: Responsiveness: Keep text readable on different devices and screen sizes. Consistency: Make sure font sizes are consistent throughout your website. Scalability: Easily change the global font size by adjusting the root element font size.
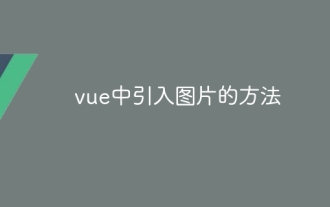
There are five ways to introduce images in Vue: through URL, require function, static file, v-bind directive and CSS background image. Dynamic images can be handled in Vue's computed properties or listeners, and bundled tools can be used to optimize image loading. Make sure the path is correct otherwise a loading error will appear.
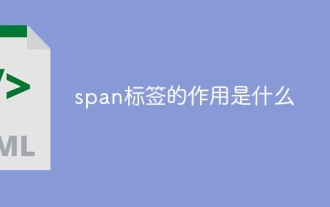
The SPAN tag is an inline HTML tag that is used to highlight text by applying attributes such as style, color, and font size. This includes emphasizing text, grouping text, adding hover effects, and dynamically updating content. It is used by placing <span> and </span> tags around the text you want to emphasize, and is manipulated via CSS styling or JavaScript. The benefits of SPAN tags include semantic clarity, styling flexibility, and ease of maintenance.

When using the prompt() method in JavaScript, you can achieve line breaks through the following three methods: 1. Insert the "\n" character at the position where you want to break the line; 2. Use the line break character in the prompt text; 3. Use CSS's "white" -space: pre" style forces line breaks.
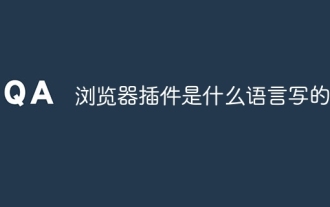
Browser plug-ins are usually written in the following languages: Front-end languages: JavaScript, HTML, CSS Back-end languages: C++, Rust, WebAssembly Other languages: Python, Java
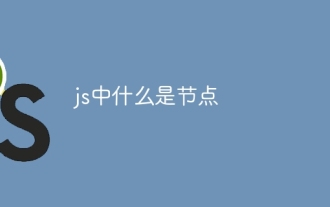
Nodes are entities in the JavaScript DOM that represent HTML elements. They represent a specific element in the page and can be used to access and manipulate that element. Common node types include element nodes, text nodes, comment nodes, and document nodes. Through DOM methods such as getElementById(), you can access nodes and operate on them, including modifying properties, adding/removing child nodes, inserting/replacing nodes, and cloning nodes. Node traversal helps navigate within the DOM structure. Nodes are useful for dynamically creating page content, event handling, animation, and data binding.
