


How does Java security mechanism handle security incidents and vulnerabilities?
Java security mechanisms include: security manager (checking sensitive operations); access control (restricting resource access); encryption (providing symmetric and asymmetric encryption); logging (used to record security events); in practical cases, Java SQL injection vulnerabilities can be handled using parameterized queries and input validation to ensure application and data security.
Java security mechanism: Handling security events and vulnerabilities
Java provides a comprehensive security mechanism to handle security events and vulnerabilities to ensure that applications and data are protected Protect. These mechanisms include:
Security Manager
The security manager is a component that inspects sensitive operations, such as file access or network connections, to ensure that they are performed by trusted code. Security Manager can be configured by modifying the policy file.
// 实例化安全管理器 SecurityManager securityManager = new SecurityManager(); // 启用安全管理器 System.setSecurityManager(securityManager); // 敏感代码(例如文件访问) try { File myFile = new File("myfile.txt"); myFile.createNewFile(); } catch (SecurityException e) { // 如果安全管理器阻止了敏感操作,则捕获SecurityException System.err.println("无法创建文件:" + e.getMessage()); }
Access Control
Java uses access control (permissions) to restrict access to sensitive resources such as the file system or network. Permissions can be set through code (using the Permissions
class) or in a policy file (using the PolicyManager
).
// 创建文件权限 Permission filePermission = new FilePermission("/myfile.txt", "read"); // 检查当前代码是否具有该权限 if (AccessController.checkPermission(filePermission)) { // 代码具有权限,可以访问文件 } else { // 代码不具有权限,无法访问文件 }
Encryption
Java provides a range of encryption functions, such as:
- Symmetric encryption: Use the same key for encryption and decryption (e.g., AES)
- Asymmetric encryption: Use different keys for encryption and decryption (e.g., RSA)
- Hashing: Use a one-way function to generate a unique value for the data (for example, SHA-256)
// 创建对称加密器 Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding"); // 使用密钥对数据加密 byte[] encryptedData = cipher.doFinal(data.getBytes());
Logging
Java provides extensive logging capabilities using the following packages:
java.util.logging
: Standard logging APIlog4j
: Popular and powerful third-party logging library
Logging can be used to record security events and exceptions in applications for analysis and forensics.
// 获取日志记录器 Logger logger = Logger.getLogger("myLogger"); // 记录一条信息日志消息 logger.info("信息:应用程序初始化成功");
Practical case: Dealing with SQL injection vulnerabilities
SQL injection vulnerabilities allow attackers to modify the database by constructing malicious queries. Java can handle this vulnerability using the following methods:
Use Parameterized queries: Use question marks (?) as placeholders for query parameters to prevent malicious code injection into the SQL statement.
// 使用参数化查询 String sql = "SELECT * FROM users WHERE username = ?"; PreparedStatement statement = connection.prepareStatement(sql); statement.setString(1, username);
Copy after loginUse Input Validation: Check user input before executing the query to ensure there are no malicious characters.
// 检查用户输入是否包含SQL注入字符 if (username.contains("'") || username.contains(";")) { throw new SQLException("非法字符"); }
Copy after loginBy using these mechanisms, Java can effectively handle security events and vulnerabilities, ensuring the security of applications and data.
The above is the detailed content of How does Java security mechanism handle security incidents and vulnerabilities?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


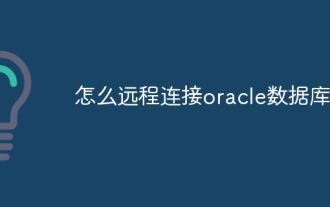
Remotely connecting to Oracle requires a listener, service name and network configuration. 1. The client request is forwarded to the database instance through the listener; 2. The instance verifies the identity and establishes a session; 3. The user name/password, host name, port number and service name must be specified to ensure that the client can access the server and the configuration is consistent. When the connection fails, check the network connection, firewall, listener and username and password. If the ORA-12154 error, check the listener and network configuration. Efficient connections require connection pooling, optimization of SQL statements and selection of appropriate network environments.

Creating an Oracle database is not easy, you need to understand the underlying mechanism. 1. You need to understand the concepts of database and Oracle DBMS; 2. Master the core concepts such as SID, CDB (container database), PDB (pluggable database); 3. Use SQL*Plus to create CDB, and then create PDB, you need to specify parameters such as size, number of data files, and paths; 4. Advanced applications need to adjust the character set, memory and other parameters, and perform performance tuning; 5. Pay attention to disk space, permissions and parameter settings, and continuously monitor and optimize database performance. Only by mastering it skillfully requires continuous practice can you truly understand the creation and management of Oracle databases.
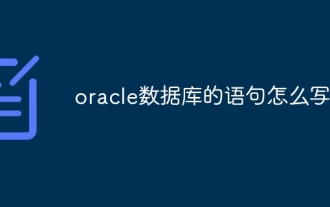
The core of Oracle SQL statements is SELECT, INSERT, UPDATE and DELETE, as well as the flexible application of various clauses. It is crucial to understand the execution mechanism behind the statement, such as index optimization. Advanced usages include subqueries, connection queries, analysis functions, and PL/SQL. Common errors include syntax errors, performance issues, and data consistency issues. Performance optimization best practices involve using appropriate indexes, avoiding SELECT *, optimizing WHERE clauses, and using bound variables. Mastering Oracle SQL requires practice, including code writing, debugging, thinking and understanding the underlying mechanisms.
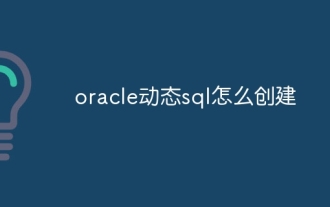
SQL statements can be created and executed based on runtime input by using Oracle's dynamic SQL. The steps include: preparing an empty string variable to store dynamically generated SQL statements. Use the EXECUTE IMMEDIATE or PREPARE statement to compile and execute dynamic SQL statements. Use bind variable to pass user input or other dynamic values to dynamic SQL. Use EXECUTE IMMEDIATE or EXECUTE to execute dynamic SQL statements.
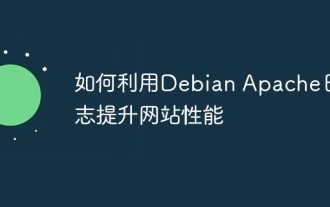
This article will explain how to improve website performance by analyzing Apache logs under the Debian system. 1. Log Analysis Basics Apache log records the detailed information of all HTTP requests, including IP address, timestamp, request URL, HTTP method and response code. In Debian systems, these logs are usually located in the /var/log/apache2/access.log and /var/log/apache2/error.log directories. Understanding the log structure is the first step in effective analysis. 2. Log analysis tool You can use a variety of tools to analyze Apache logs: Command line tools: grep, awk, sed and other command line tools.
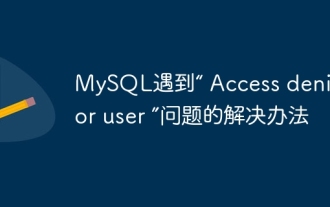
How to solve the MySQL "Access denied for user" error: 1. Check the user's permission to connect to the database; 2. Reset the password; 3. Allow remote connections; 4. Refresh permissions; 5. Check the database server configuration (bind-address, skip-grant-tables); 6. Check the firewall rules; 7. Restart the MySQL service. Tip: Make changes after backing up the database.
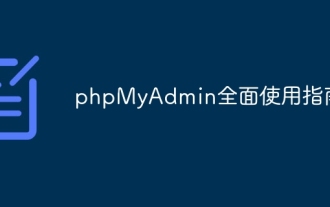
phpMyAdmin is not just a database management tool, it can give you a deep understanding of MySQL and improve programming skills. Core functions include CRUD and SQL query execution, and it is crucial to understand the principles of SQL statements. Advanced tips include exporting/importing data and permission management, requiring a deep security understanding. Potential issues include SQL injection, and the solution is parameterized queries and backups. Performance optimization involves SQL statement optimization and index usage. Best practices emphasize code specifications, security practices, and regular backups.
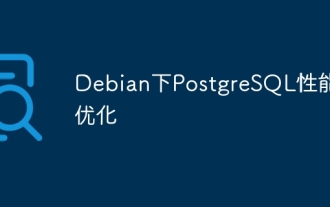
To improve the performance of PostgreSQL database in Debian systems, it is necessary to comprehensively consider hardware, configuration, indexing, query and other aspects. The following strategies can effectively optimize database performance: 1. Hardware resource optimization memory expansion: Adequate memory is crucial to cache data and indexes. High-speed storage: Using SSD SSD drives can significantly improve I/O performance. Multi-core processor: Make full use of multi-core processors to implement parallel query processing. 2. Database parameter tuning shared_buffers: According to the system memory size setting, it is recommended to set it to 25%-40% of system memory. work_mem: Controls the memory of sorting and hashing operations, usually set to 64MB to 256M
