golang function variable parameter passing
Go language function variable parameter passing allows a function to accept any number of parameters, marked by an ellipsis..., and passed to the function as a slice type. In practical applications, variable parameters are often used to process an indefinite number of inputs, such as calculating the average of a numerical sequence. When using it, please note that the variable parameter must be the last parameter of the function, avoid overuse, and consider explicit type assertion.
Go language function variable parameter passing detailed explanation
Introduction
In the Go language, function variable parameter passing allows the function to accept a number Indefinite parameters, which are useful when you need to handle an indefinite number of inputs.
Syntax
Variable parameter passing is marked using ...
(ellipsis) in the function declaration, as follows:
1 |
|
Here, myFunc
The function receives the first parameter as a string arg1
, and subsequent parameters as variable parameters args
, and as a type of []int The slice of
is passed to the function.
Practical Case
Consider a scenario where you need to write a function to calculate the average of a given sequence of numbers:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
In this case:
-
average
Function declaration has variadic parametersnumbers
. - Main function
main
Use ellipsis...
to expand thenumbers
slice and pass it as a variable parameter toaverage
function. - The function uses a loop internally to find the sum of the numbers and divides it by the length of the number sequence to calculate the average.
- Finally, print the calculated average.
Notes
When using variable parameter transfer, you need to pay attention to the following:
- The variable parameter must be the last parameter of the function.
- If you need to handle values of different types, you need to make explicit type assertions.
- Avoid excessive use of variadic parameters as it can make the code difficult to understand and maintain.
The above is the detailed content of golang function variable parameter passing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


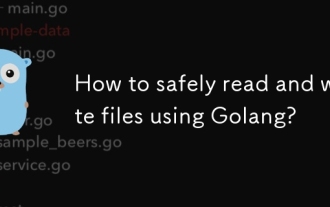
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
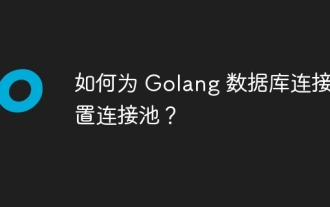
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
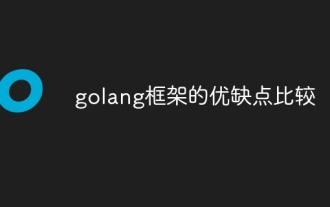
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
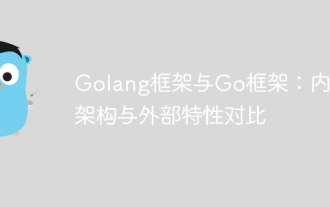
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
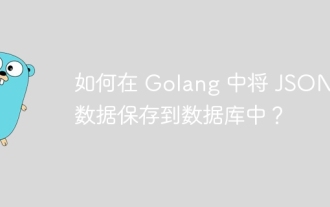
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
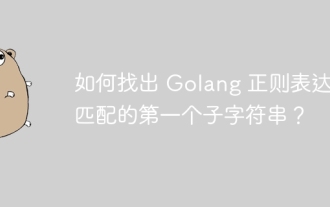
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
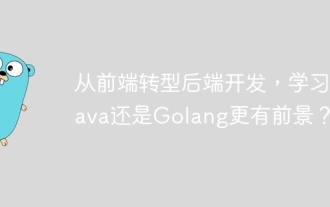
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
