


Recursive implementation of C++ functions: How to use recursion to solve mathematical problems?
Recursion is a programming technique in which a function calls itself, used to solve complex problems. In mathematical problems, recursion is widely used, for example: calculating factorial: factorial(n) = n * factorial(n-1) if n > 0, factorial(0) = 1 calculating Fibonacci sequence: fibonacci(n) = fibonacci(n-1) fibonacci(n-2) if n > 1, fibonacci(0) = 0, fibonacci(1) = 1
Recursive implementation of C function: using recursion to solve mathematical problems
What is recursion?
Recursion is a programming technique where a function calls itself. This allows us to solve complex problems in a simple and elegant way.
Example of recursively solving a mathematical problem
Calculating factorial
Factorial is a mathematical function that takes a given A positive integer n maps to the product of all its positive integer factors. It can be defined using the following recursive relationship:
factorial(n) = 1 if n == 0 factorial(n) = n * factorial(n-1) if n > 0
Sample Code
int factorial(int n) { if (n == 0) { return 1; } return n * factorial(n-1); }
Calculate Fibonacci Sequence
Fibo The Nachi sequence is a sequence of numbers in which each number is the sum of the previous two numbers. It can be defined with the following recursive relationship:
fibonacci(0) = 0 fibonacci(1) = 1 fibonacci(n) = fibonacci(n-1) + fibonacci(n-2) if n > 1
Sample code
int fibonacci(int n) { if (n <= 1) { return n; } return fibonacci(n-1) + fibonacci(n-2); }
Advantages
Recursion has the following advantages:
- Clean and elegant
- Easy to understand and implement
- Can be used to solve many complex mathematical problems
Limitations
Recursion also has some limitations:
- For large problems, stack overflow errors may occur
- The efficiency may not be as efficient as iterative implementation
Practical cases
The following are some practical cases of using recursion to solve mathematical problems:
- Calculating the square root of a number
- Solving one dollar Quadratic equation
- Find the maximum value in an array
- Sort an array
The above is the detailed content of Recursive implementation of C++ functions: How to use recursion to solve mathematical problems?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


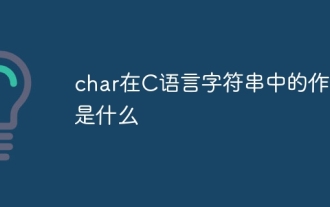
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
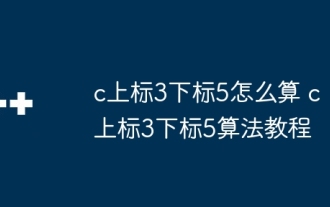
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
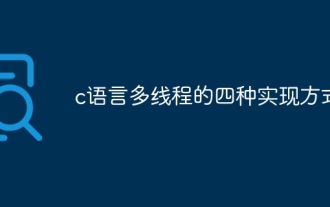
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
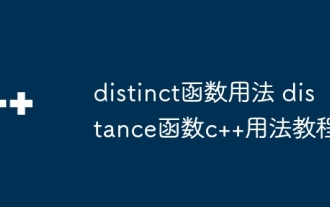
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
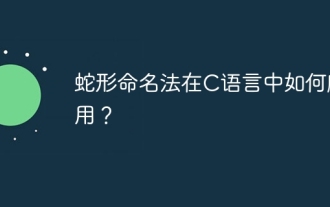
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
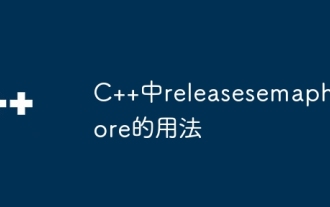
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
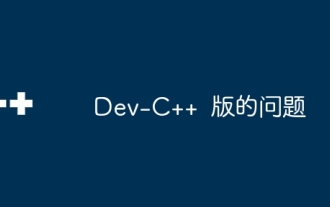
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
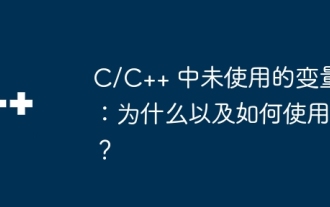
In C/C code review, there are often cases where variables are not used. This article will explore common reasons for unused variables and explain how to get the compiler to issue warnings and how to suppress specific warnings. Causes of unused variables There are many reasons for unused variables in the code: code flaws or errors: The most direct reason is that there are problems with the code itself, and the variables may not be needed at all, or they are needed but not used correctly. Code refactoring: During the software development process, the code will be continuously modified and refactored, and some once important variables may be left behind and unused. Reserved variables: Developers may predeclare some variables for future use, but they will not be used in the end. Conditional compilation: Some variables may only be under specific conditions (such as debug mode)
