


What security considerations should you be aware of when creating custom PHP functions?
Security considerations for PHP custom functions include: validating user input to prevent injection and cross-site scripting attacks; limiting function usage to prevent type coercion attacks; using parameter whitelists to only allow expected input values; escaping output , prevent cross-site scripting attacks; restrict function access, hide implementation details and prevent unauthorized access.
Security Considerations in PHP Custom Functions
When creating custom functions in PHP, it is crucial to ensure their security to prevent Potential malicious use. Here are some key security considerations:
1. Validate user input
Use the filter_input()
or filter_var()
function to validate user input , to ensure it is well-formed and contains the expected data. By eliminating malicious characters, you can prevent code injection and cross-site scripting attacks.
<?php function sanitizeInput($input) { return filter_input(INPUT_POST, $input, FILTER_SANITIZE_STRING); } ?>
2. Restrict the use of functions
Use the declare(strict_types=1);
statement to enable strict typing and force the use of typed variables. This helps prevent type coercion attacks, where an attacker attempts to pass an unexpected value to a function.
<?php declare(strict_types=1); function sum(int $a, int $b): int { return $a + $b; } ?>
3. Use parameter whitelist
Create a parameter whitelist that only allows expected input values. Use the in_array()
or array_key_exists()
function to check if the input matches the whitelist.
<?php function checkRole($role) { $validRoles = ['user', 'admin', 'moderator']; return in_array($role, $validRoles); } ?>
4. Escape output
Use htmlspecialchars()
or htmlentities()
function to escape output to prevent cross-site scripting attacks, This attack allows an attacker to inject malicious code on your website.
<?php function displayMessage($message) { echo htmlspecialchars($message); } ?>
5. Restrict function access
Use visibility specifiers (public
, protected
, private
) to restrict functions Access. This helps hide implementation details and prevent unauthorized access.
<?php class User { private function getPassword() { // ... } } ?>
Practical Case
Consider a sample PHP function that allows the user to enter an email address for verification. By following these security considerations, we can ensure that our functions are safe and secure:
<?php function validateEmail($email) { // 验证输入 $email = filter_input(INPUT_POST, 'email', FILTER_SANITIZE_EMAIL); // 检查电子邮件格式 if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { throw new InvalidArgumentException('Invalid email address'); } // 检查长度 if (strlen($email) > 255) { throw new InvalidArgumentException('Email address too long'); } // 检查域名是否存在 $domain = explode('@', $email)[1]; if (!checkdnsrr($domain, 'MX')) { throw new InvalidArgumentException('Invalid domain'); } return true; } ?>
By following these security considerations, we can ensure that our custom PHP functions are safe from common attacks and protect users and applications.
The above is the detailed content of What security considerations should you be aware of when creating custom PHP functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


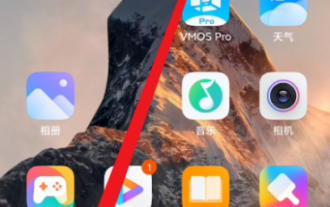
RedmiNote13RPro is a new model with very good performance and configuration. This phone has a very useful function of hiding photo albums. It can help users hide their photo albums so that others cannot view your photo albums. Next, the editor will tell you how to hide the photo album on Redmi Note13R Pro to help you protect your privacy. How to hide photo album on Redmi Note13RPro? 1. Enter the settings of your Xiaomi phone. 2. Then click Privacy and Protection. 3. Click Protect Privacy here again. 4. Click on the safe. 5. Finally, you can set up a private photo album here. Frequently Asked Questions Connecting to Bluetooth Changing Input Method Theme Changing Factory Settings Hide Applications Mirroring TV NFC Enable Dual SIM Installation Activation Time
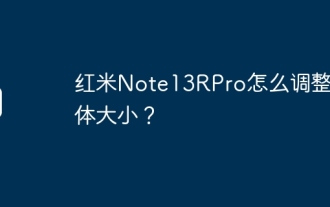
Do not change the meaning of the original content, fine-tune the content, rewrite the content, do not continue. Redmi Note13RPro not only has excellent performance, but also has an even better user experience. In order to give everyone a more comfortable experience, Redmi Note13RPro is equipped with a font adjustment function to allow users to Ability to adjust fonts for your phone. If you want to know how to adjust the font size of Redmi Note13R Pro, then take a look. How to adjust the font size on Redmi Note13RPro? 1. Open the Settings app. 2. Scroll to the bottom and select "Display." 3. Click "Text Size". 4. On this screen, you can adjust the font size by dragging the slider or select a preset font size. 5. After the adjustment is completed, press "OK" to save
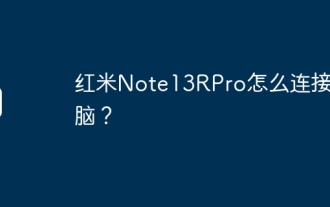
The phone Redmi Note13RPro has been very popular recently. Many consumers have purchased this phone. However, many users are using this phone for the first time, so they don’t know how to connect the Redmi Note13RPro to the computer. In this regard, the editor is here to explain to you Detailed tutorial introduction is provided. How to connect Redmi Note13RPro to the computer? 1. Use a USB data cable to connect the Redmi phone to the USB interface of the computer. 2. Open the phone settings, click Options, and turn on USB debugging. 3. Open the device manager on your computer and find the mobile device option. 4. Right-click the mobile device, select Update Driver, and then select Automatically search for updated drivers. 5. If the computer does not automatically search for the driver,
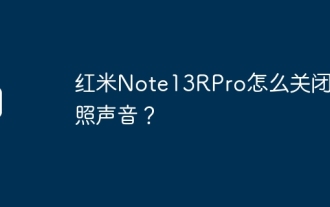
The camera function of Redmi Note13RPro is very easy to use, especially when taking pictures, it will simulate the sound of the camera shutter. However, this function may affect others in quiet situations such as libraries, so many users want to know how to turn off the camera sound of Redmi Note13RPro. , let the editor tell you below. How to turn off the camera sound on Redmi Note13RPro? 1. For the first method, you first need to open the settings of your phone. 2. Then find system applications under the settings menu. 3. Then we find the camera option under the system application interface. 4. Finally, we can set whether to turn off the camera sound in the camera interface. We only need to turn off the switch on the right side of the camera sound. 5. The second method, the first step, open
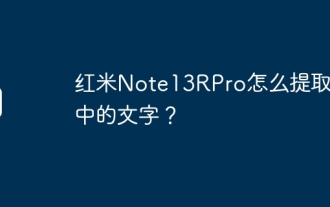
The Redmi Note13RPro mobile phone integrates a number of smart tools in terms of software functions. Among them, quickly and accurately extracting text content from pictures is one of them. The following editor will introduce to you how Redmi Note13RPro extracts text from pictures. How to extract text from pictures on Redmi Note13RPro? Use the Xiaomi QR code scanning function, open the QR code scanning application on your phone, click the picture icon, select a picture, and then click the "Recognize text" option on the right to successfully extract the text in the picture. Operate through mobile phone album. Find the picture for which text needs to be extracted in the mobile phone album, click "More" below the picture, and select "Extract Text". After successful recognition, you can copy or save the text as needed. Use WeChat mini programs. Open micro
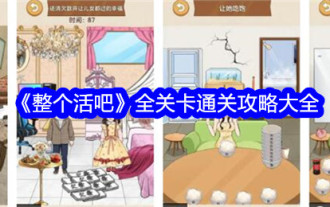
Entire Huoba is a very fun casual puzzle game that everyone can experience on multiple platforms! The game provides countless exciting levels waiting to be unlocked. Each level is full of novelty and challenges, allowing you to experience an interesting adventure of answering questions and unlock new game content. So how to clear the entire level of Huoba? Today I bring you a complete guide to all levels of "Whole Life". If you encounter any problems in passing the level, you can refer to it! "The Whole Life" complete guide to all levels of "The Whole Life" The complete guide to all levels of "The Whole Life" Help her to watch the New Year's Eve, help the little sister to identify the scumbag wolf, and the grandmother helps the little girl escape from danger for outdoor adventure, pretending not to see Ah Piao and transforming back The humanoid helped the orangutan to become a humanoid. Someone in the back seat stayed calm and dealt with the female ghost. The night-shift taxi kept calm and dealt with the female ghost.
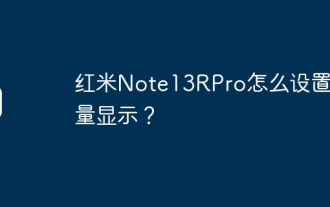
RedmiNote13RPro is a mobile phone that is loved by many users. This phone provides a special method to display traffic on the phone. If you still don’t know how to set up RedmiNote13RPro to display traffic, then follow the editor to find out. How to set traffic display on Redmi Note13RPro? 1. Enter "Settings" and click "Dual SIM Mobile Network". 2. Scroll down to select the "Data Package Settings" option. 3. Turn on the function switches on the right side of "Display traffic information in the notification bar" and "Display the current network speed in the status bar". 4. After the setting is successful, pull down the status bar to see the display of real-time network speed and traffic information. FAQ Connect Bluetooth Change input method theme Change factory settings Hide application casting
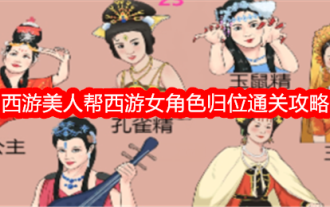
Entire Huoba is a very popular fun level-breaking game on Douyin. There are many levels in the game waiting for everyone to challenge! In the game level "Journey to the West Beauty", everyone needs to help the female character of "Journey to the West" return to her position. How to pass the level? The following is a guide for you to clear the level of "Journey to the West" brought to you by the beauty of Journey to the West. Friends who don't know how to pass the level, let's take a look. I hope it can help you. "Whole Life" Journey to the West beauty helps the female character of Journey to the West to return to her place. Strategy for clearing the stage. The beauty of Journey to the West helps the female character of Journey to the West to return to her place. 1. Click on the scroll to appear the female character. 2. The white-bone spirit and the peacock spirit need to click to switch actions. 3. The answer is as shown in the figure below: "The whole life" Let's Live" has a complete guide to all levels, helping her to be a watcher of the year, helping the little sister to identify the scumbag and the wolf, and the grandmother to help the little girl escape from danger and have outdoor adventures.
