What is the difference between Java functions and Go language functions?
The main difference between Java and Go language functions is: Creating functions: Java requires explicitly specifying types, while Go has type inference. Number of parameters: Fixed in Java, variable in Go (variable length parameters). Number of return values: Java can only return one, Go can return multiple (tuples). Pass by value and pass by reference: Java passes by value, Go passes by reference by default.
The difference between Java functions and Go language functions
Overview
Java and Go There are differences in the handling of functions between the two programming languages. This article will introduce the main differences between Java functions and Go language functions.
Creating functions
In Java, function declarations use the public static
keyword as follows:
public static int sum(int a, int b) { return a + b; }
In In the Go language, function declarations begin with the func
keyword, as shown below:
func sum(a, b int) int { return a + b }
Type inference
Java requires explicit The formula specifies the parameter type and return value type. The Go language has a type inference function that can automatically infer types based on parameter values.
func sum(a, b int) int { return a + b }
Number of parameters
The number of parameters of a Java function must be fixed. The number of parameters of Go language functions can be variable, which is called "variable length parameters".
func sum(numbers ...int) int { total := 0 for _, num := range numbers { total += num } return total }
Number of return values
Java functions can only return one value. Go language functions can return multiple values, called "tuples".
func minMax(numbers ...int) (int, int) { min := numbers[0] max := numbers[0] for _, num := range numbers { if num < min { min = num } if num > max { max = num } } return min, max }
Value passing and reference passing
Java functions use value passing, that is, a copy of the parameter value is passed. The Go language function uses reference passing by default, that is, the reference of the parameter is passed.
// Java public static void changeValue(int value) { value = 100; }
// Go func changeValue(value *int) { *value = 100 }
Practical case
The following is a function that calculates the sum of two numbers, showing the difference between Java and Go language functions:
Java
public static int sum(int a, int b) { return a + b; } public static void main(String[] args) { int result = sum(10, 20); System.out.println(result); // 输出:30 }
Go Language
func sum(a, b int) int { return a + b } func main() { result := sum(10, 20) println(result) // 输出:30 }
As can be seen from this example, the parameter and return value types of Java functions must be explicitly specified. Go language functions support type inference and variable-length parameters.
The above is the detailed content of What is the difference between Java functions and Go language functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
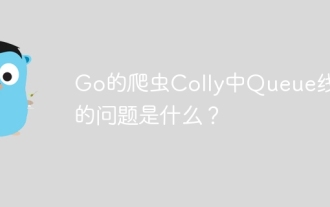
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
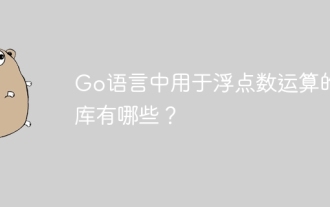
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
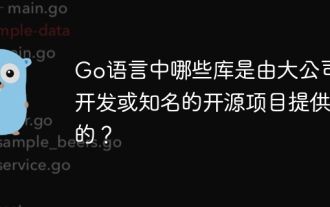
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
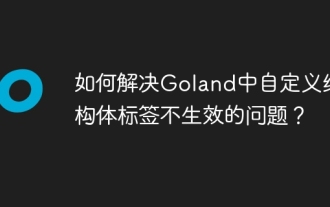
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
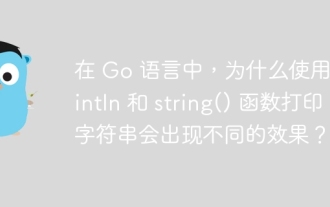
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
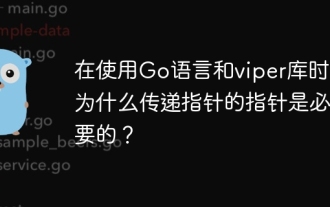
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
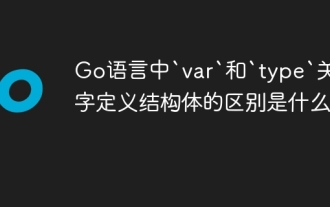
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
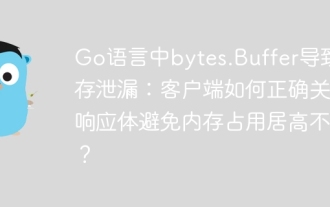
Analysis of memory leaks caused by bytes.makeSlice in Go language In Go language development, if the bytes.Buffer is used to splice strings, if the processing is not done properly...
