Exception handling technology in C++ function performance optimization
Exception handling technology in C function performance optimization: reducing exception throwing: input validation, resource management, error handling. Fine-grained catching and handling: use try-catch blocks and specific exception classes. Use exception handling libraries: The C standard library or third-party libraries provide more robust and efficient error handling.
Exception handling technology in C function performance optimization
Exception handling is a mechanism in C to handle runtime errors. But it may also have an impact on function performance. This article describes how to use exception handling techniques to optimize C function performance.
Minimize exception throwing
Exception throwing is an expensive operation because it requires saving stack information and performing unwind operations. Therefore, exceptions should be thrown as little as possible. Consider using the following techniques:
- Input validation: On function input, check whether parameters are valid and, if not, return an appropriate error code or value.
- Resource management: Use RAII (resource acquisition and initialization) technology to manage resources and clean up when resources are destroyed to avoid exceptions.
- Error handling: Use error handling mechanisms such as try-catch blocks instead of throwing exceptions to handle errors.
Catching and Handling Exceptions
If it is unavoidable to throw an exception, you should use a try-catch
block to catch and Handle exceptions. Use specific exception classes for fine-grained catching to avoid catching all exceptions:
try { // 业务逻辑 } catch (const std::invalid_argument& e) { // 处理无效参数异常 } catch (const std::out_of_range& e) { // 处理超出范围异常 }
Use exception handling library
For complex or frequent exception handling, you can use the C standard library or a third-party exception handling library. These libraries provide more robust and efficient error handling. For example, the Boost.Exception library provides custom exception types, convenient error handling, and other advanced features.
Practical Case
Consider the following function, which parses a string and converts it to an integer:
int parse_int(const std::string& str) { try { return std::stoi(str); } catch (const std::invalid_argument& e) { throw std::invalid_argument("Invalid integer string."); } }
By using input validation and fine-graining Exception catching, this function can handle invalid input efficiently without throwing a general exception. This improves function performance and provides better error handling.
Conclusion
By using exception handling techniques, programmers can optimize the performance of C functions while still maintaining reliability. Performance can be significantly improved by reducing exception throwing, using try-catch
blocks, and leveraging exception handling libraries.
The above is the detailed content of Exception handling technology in C++ function performance optimization. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

C++ object layout and memory alignment optimize memory usage efficiency: Object layout: data members are stored in the order of declaration, optimizing space utilization. Memory alignment: Data is aligned in memory to improve access speed. The alignas keyword specifies custom alignment, such as a 64-byte aligned CacheLine structure, to improve cache line access efficiency.
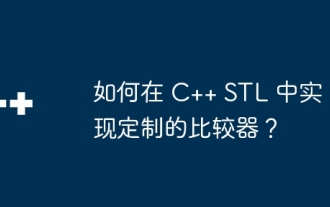
Implementing a custom comparator can be accomplished by creating a class that overloads operator(), which accepts two parameters and indicates the result of the comparison. For example, the StringLengthComparator class sorts strings by comparing their lengths: Create a class and overload operator(), returning a Boolean value indicating the comparison result. Using custom comparators for sorting in container algorithms. Custom comparators allow us to sort or compare data based on custom criteria, even if we need to use custom comparison criteria.
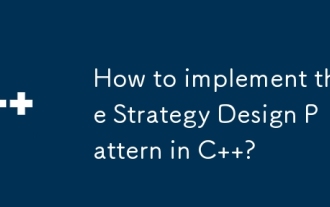
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
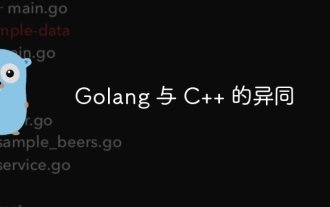
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
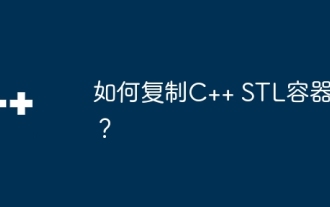
There are three ways to copy a C++ STL container: Use the copy constructor to copy the contents of the container to a new container. Use the assignment operator to copy the contents of the container to the target container. Use the std::copy algorithm to copy the elements in the container.
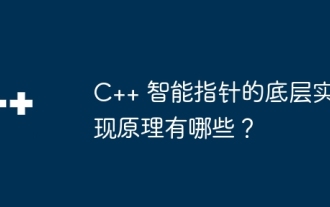
C++ smart pointers implement automatic memory management through pointer counting, destructors, and virtual function tables. The pointer count keeps track of the number of references, and when the number of references drops to 0, the destructor releases the original pointer. Virtual function tables enable polymorphism, allowing specific behaviors to be implemented for different types of smart pointers.
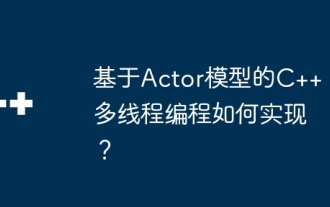
C++ multi-threaded programming implementation based on the Actor model: Create an Actor class that represents an independent entity. Set the message queue where messages are stored. Defines the method for an Actor to receive and process messages from the queue. Create Actor objects and start threads to run them. Send messages to Actors via the message queue. This approach provides high concurrency, scalability, and isolation, making it ideal for applications that need to handle large numbers of parallel tasks.
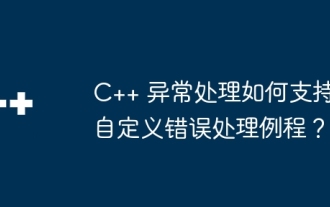
C++ exception handling allows the creation of custom error handling routines to handle runtime errors by throwing exceptions and catching them using try-catch blocks. 1. Create a custom exception class derived from the exception class and override the what() method; 2. Use the throw keyword to throw an exception; 3. Use the try-catch block to catch exceptions and specify the exception types that can be handled.
