


What advantages do C++ functions have when handling user input and events?
C functions handle user input and events with the following benefits: Modular and reusable code: Break down tasks, simplify testing and improve code quality. Input validation and exception handling: Ensure user input is valid and provide consistent error handling. Event handling: Use event handlers to create interactive applications in response to user interaction or system state changes.
Advantages of C functions in handling user input and events
When developing C applications, functions are used in handling user input and events play a key role. Functions provide the advantages of modular and reusable code, allowing developers to build robust applications more efficiently.
Modular and reusable code
Functions break complex tasks into smaller, manageable parts. This allows developers to easily isolate and test each operation, reducing errors and improving code quality. Additionally, functions can be reused, saving programming time and promoting code consistency.
Input validation and exception handling
C function can implement the input validation mechanism to ensure that user input is valid. For example, invalid input can be prevented by passing user input as arguments to functions and bounds checking the values. Functions also handle errors and exceptions efficiently, providing consistent error messages and a more user-friendly interface.
Event handling
C functions are particularly powerful in handling events, which are blocks of code triggered by user actions or external stimuli. Functions that respond to events are called event handlers, and they allow developers to create interactive applications that react to user interaction or changes in system state.
Practical case
The following C code demonstrates how to use functions to handle user input and respond to events:
// 处理用户输入的函数 int getUserInput() { int input; cout << "Enter a number: "; cin >> input; return input; } // 事件处理程序函数 void onButtonClicked() { cout << "Button clicked!" << endl; } int main() { // 获取用户输入 int num = getUserInput(); // 根据用户输入执行操作 if (num % 2 == 0) { cout << "The number is even." << endl; } else { cout << "The number is odd." << endl; } // 处理按钮点击事件 onButtonClicked(); return 0; }
In this example, The getUserInput()
function is responsible for obtaining user input, and the onButtonClicked()
function serves as the handler for the button click event. The modular and reusable nature of functions makes code easier to maintain and ensures a consistent user experience.
The above is the detailed content of What advantages do C++ functions have when handling user input and events?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


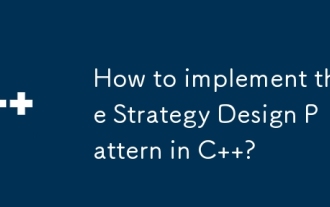
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
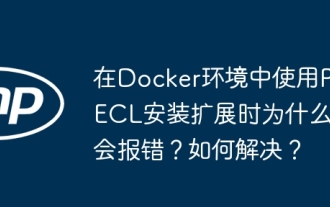
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
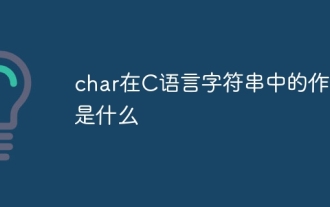
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
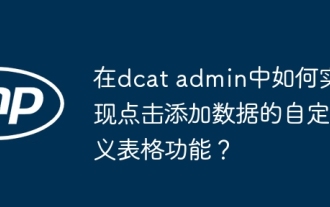
How to implement the table function of custom click to add data in dcatadmin (laravel-admin) When using dcat...
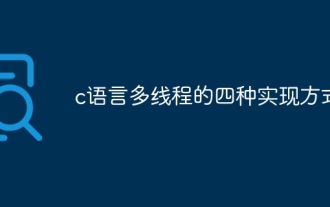
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
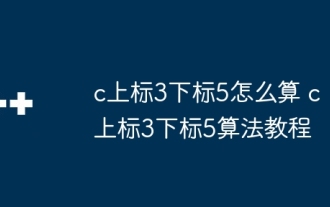
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
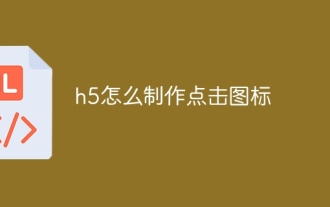
The steps to create an H5 click icon include: preparing a square source image in the image editing software. Add interactivity in the H5 editor and set the click event. Create a hotspot that covers the entire icon. Set the action of click events, such as jumping to the page or triggering animation. Export H5 documents as HTML, CSS, and JavaScript files. Deploy the exported files to a website or other platform.
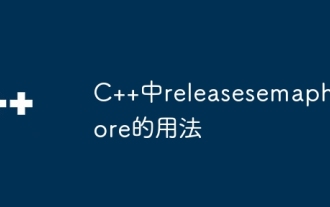
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
