Nested use of Java function generics
Nested generics allow the use of other generic types within generic methods, creating highly reusable code. In practice, nested generics can be used to find two peak elements in an array. The sample code uses the generic Pair to store the results and finds the peak value by iteratively checking the elements and comparing them with adjacent elements.
Nested use of Java function generics
Generics are a powerful Java language feature that allows us to create Reusable code that can operate on a variety of data types. Function generics allow us to use generics within functions, thus increasing flexibility.
Nested generics
Nested generics refer to using other generic types inside generic methods. This allows us to create highly reusable code, especially when dealing with complex data structures.
The following is an example of a nested generic:
public class Pair<T, U> { private T first; private U second; public Pair(T first, U second) { this.first = first; this.second = second; } public T getFirst() { return first; } public U getSecond() { return second; } }
The Pair
class is a nested generic that stores two elements of different types.
Practical case
Let us consider a scenario where we need to return an array nums
of two peak elements. A peak element is an element that is larger than its neighboring elements.
The following is a Java code using nested generics:
public class FindPeakElements { public static <T extends Comparable<T>> Pair<T, T> findPeakElements(T[] nums) { if (nums == null || nums.length < 3) { throw new IllegalArgumentException("Invalid array size"); } T firstPeak = nums[0]; T secondPeak = nums[nums.length - 1]; for (int i = 1; i < nums.length - 1; i++) { T current = nums[i]; T prev = nums[i - 1]; T next = nums[i + 1]; if (current > prev && current > next) { if (current > firstPeak) { secondPeak = firstPeak; firstPeak = current; } else if (current > secondPeak) { secondPeak = current; } } } return new Pair<>(firstPeak, secondPeak); } public static void main(String[] args) { Integer[] nums = {1, 2, 3, 4, 5, 6, 7, 6, 5, 4, 3, 2, 1}; Pair<Integer, Integer> peaks = findPeakElements(nums); System.out.println("First peak: " + peaks.getFirst()); System.out.println("Second peak: " + peaks.getSecond()); } }
This code uses nested generics Pair
to store two peak elements. It finds the peak element by iterating through the array and checking if the element is larger than its neighbors.
The above is the detailed content of Nested use of Java function generics. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


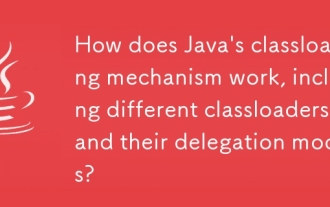
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
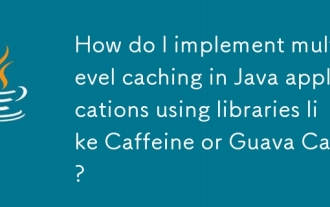
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
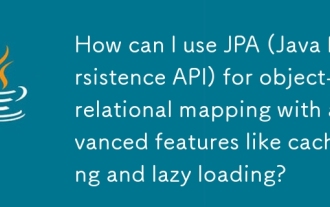
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
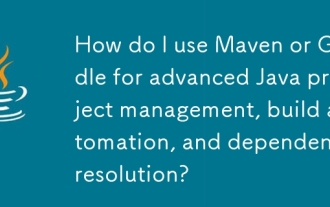
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
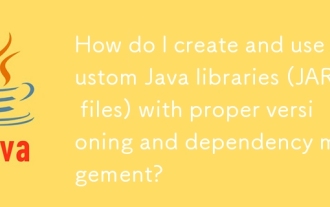
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
