Golang function performance optimization and scalability
Apply the following techniques to optimize Go function performance and scalability: Function decomposition: Break large functions into smaller reusable functions. Parameter optimization: Use value passing when possible, and specify pointer receivers. Data structure selection: Choose a data structure suitable for the task, such as a map or a slice. Concurrency and parallelism: Take advantage of Go's concurrency features to use goroutines to execute tasks in parallel or use channels for data communication.
Go function performance optimization and scalability
Writing efficient and scalable functions in Go is critical for applications of any size of. You can improve function performance and make it more scalable by applying the following techniques:
Function Decomposition
Decompose large functions into smaller, reusable functions. This makes the code easier to maintain and test, and also reduces the number of nesting levels, thereby improving performance.
Example:
func LargeFunction() { // 大量代码 } // 将函数分解为较小的可重用函数 func SmallFunction1() { // 部分代码 } func SmallFunction2() { // 部分代码 } func LargeFunction() { SmallFunction1() SmallFunction2() }
Parameter optimization
- Use value passing when possible: Avoid using slices, maps or Large objects such as structures are passed using pointers as this creates unnecessary copies and reduces performance.
- Specify pointer receiver: If the function needs to modify the data passed to it, declare the receiver type as a pointer. This avoids the value being copied, saving memory and time.
Example:
// 值传递 func AppendToSlice(s []int) { s = append(s, 1) } // 指针接收器 func AppendToSlicePtr(s *[]int) { *s = append(*s, 1) }
Data structure selection
Choose a data structure suitable for the task. For example, if you need fast lookup, you can use a map; if you need fast traversal, you can use slices.
Example:
// 使用映射进行快速查找 countryCodes := map[string]string{ "US": "United States", "CA": "Canada", } // 使用切片进行快速遍历 countries := []string{ "United States", "Canada", }
Concurrency and Parallelism
Leverage Go’s powerful concurrency features to improve performance and increase application scalability. Use goroutines to execute tasks in parallel, or channels for data communication.
Example:
Goroutine executes tasks in parallel:
count := 0 for i := 0; i < 100000; i++ { go func() { // 并行执行代码 count++ }() }
Channel performs data communication:
// 生产者 goroutine producer := func(c chan int) { for i := 0; i < 10; i++ { c <- i } close(c) } // 消费者 goroutine consumer := func(c chan int) { for i := range c { // 处理收到的数据 fmt.Println(i) } } // 创建 channel c := make(chan int) // 启动 goroutine go producer(c) go consumer(c)
By applying these techniques, you can create high-performance, scalable Go functions that improve the overall performance and efficiency of your application.
The above is the detailed content of Golang function performance optimization and scalability. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


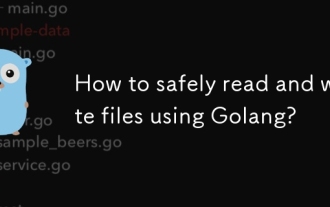
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
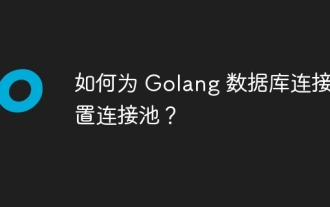
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
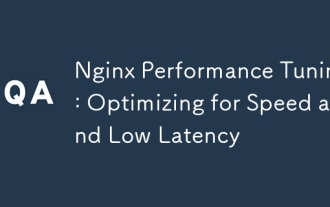
Nginx performance tuning can be achieved by adjusting the number of worker processes, connection pool size, enabling Gzip compression and HTTP/2 protocols, and using cache and load balancing. 1. Adjust the number of worker processes and connection pool size: worker_processesauto; events{worker_connections1024;}. 2. Enable Gzip compression and HTTP/2 protocol: http{gzipon;server{listen443sslhttp2;}}. 3. Use cache optimization: http{proxy_cache_path/path/to/cachelevels=1:2k
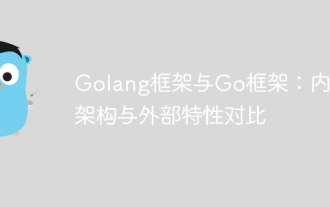
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
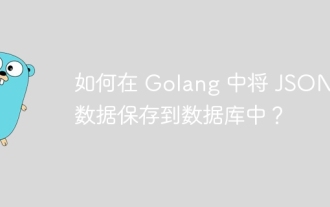
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
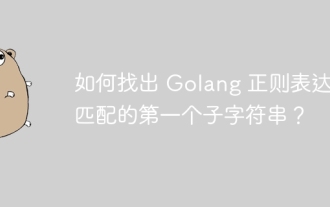
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
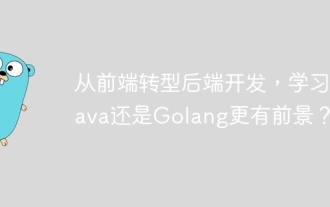
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
