


How do C++ functions handle timeouts and exceptions in network programming?
C In network programming, use the chrono library to set the timeout when processing timeouts, such as setting a 10-second timeout: std::chrono::seconds timeout = 10s;. Use try-catch statements to handle exceptions, such as: try { ... } catch (const std::exception& e) { ... }.
How C functions handle timeouts and exceptions in network programming
In network programming, timeouts and exceptions are common challenges . C provides powerful functions for handling these situations, and this article will explore how to use them effectively.
Handling timeouts
C provides the chrono
library to manage time. To set a timeout, you can use the following function:
#include <chrono> using namespace std::chrono_literals; std::chrono::seconds timeout = 10s; // 设置 10 秒的超时
Practical case: Use the select()
function to implement the timeout
select( )
Function waits for the readability of one or more file descriptors for a specific period of time. It can be used with timeouts:
#include <sys/select.h> int main() { // 设置文件描述符集合 fd_set fds; FD_ZERO(&fds); FD_SET(socket_fd, &fds); // 设置超时 struct timeval timeout; timeout.tv_sec = 10; timeout.tv_usec = 0; // 等待可读性或超时 int result = select(socket_fd + 1, &fds, NULL, NULL, &timeout); if (result == 0) { // 超时 std::cout << "Operation timed out." << std::endl; } else if (result > 0) { // 文件描述符可读 // ... } else { // 错误 std::cout << "An error occurred." << std::endl; } return 0; }
Handling Exceptions
C Use exceptions to handle exceptional situations. When an exception is thrown, it causes the immediate termination of the current function and transfers control to its caller. To catch exceptions, you can use try-catch
statements around the code block:
#include <stdexcept> try { // ... } catch (const std::exception& e) { // 异常处理 std::cout << "An exception occurred: " << e.what() << std::endl; }
Practical example: Handling std::runtime_error
exceptions in a network connection
std::runtime_error
is a commonly used exception used to represent runtime errors. It can throw when a network connection fails:
#include <iostream> using namespace std; int main() { try { // 建立网络连接 // ... } catch (const std::runtime_error& e) { // 连接失败 cout << "Connection failed: " << e.what() << endl; } return 0; }
Efficient handling of timeouts and exceptions is critical for robust and reliable network applications. C provides powerful functions that allow you to easily manage these situations and ensure that your code still works properly when unforeseen problems arise.
The above is the detailed content of How do C++ functions handle timeouts and exceptions in network programming?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


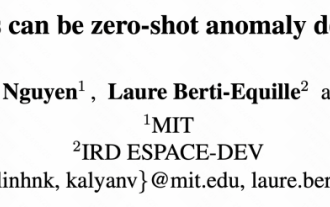
Today I would like to introduce to you an article published by MIT last week, using GPT-3.5-turbo to solve the problem of time series anomaly detection, and initially verifying the effectiveness of LLM in time series anomaly detection. There is no finetune in the whole process, and GPT-3.5-turbo is used directly for anomaly detection. The core of this article is how to convert time series into input that can be recognized by GPT-3.5-turbo, and how to design prompts or pipelines to let LLM solve the anomaly detection task. Let me introduce this work to you in detail. Image paper title: Largelanguagemodelscanbezero-shotanomalydete
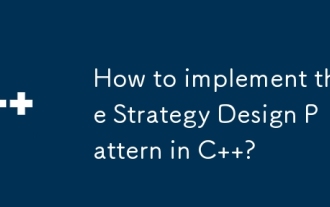
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
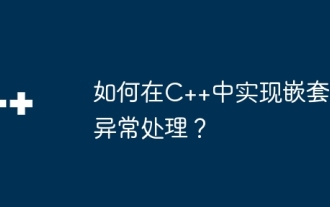
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
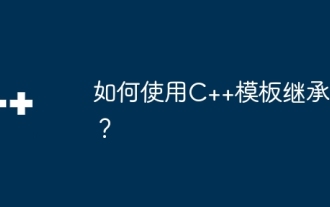
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
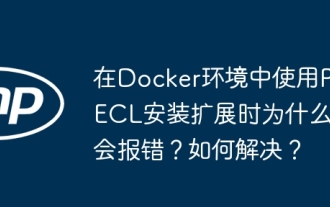
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
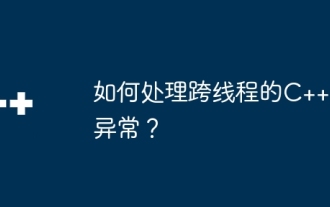
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
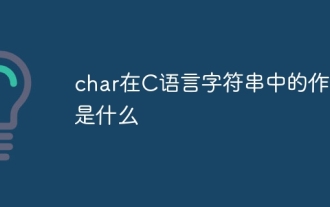
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
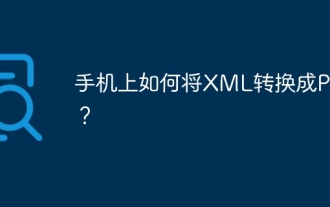
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.
