What is the role of C++ functions in cloud-based GUI applications?
C functions play a key role in cloud GUI applications, including: creating and operating GUI components, such as buttons, windows, etc.; managing and processing data, such as validating input and updating cloud data; processing network communications, such as sending HTTP Request and receive server responses; use multi-threading and concurrency to perform tasks in the background to improve application response speed.
The role of C functions in cloud-based GUI applications
In cloud-based graphical user interface (GUI) applications In the program, C functions play a vital role. These functions provide modularity and reusability of application logic and functionality. The following are some key roles of C functions in cloud-based GUI applications:
Creation and manipulation of GUI components:
- Create and manage windows, buttons , input fields, menus and toolbars and other GUI components.
- Set the component's properties such as size, position, color, and text.
- Handle component events such as clicks, keyboard input, and focus changes.
// 创建一个按钮 QPushButton* button = new QPushButton("点击我!"); // 设置按钮属性 button->setGeometry(QRect(100, 100, 100, 50)); // 设置位置和大小 button->setStyleSheet("background-color: red"); // 设置背景颜色 // 处理按钮单击事件 QObject::connect(button, SIGNAL(clicked()), this, SLOT(onButtonClicked()));
Data management and processing:
- Load and process data from the cloud.
- Validate user input and perform necessary calculations.
- Update and store application data.
// 从云端加载用户数据 QJsonArray userData = loadUser() // 验证用户输入的用户名 if (username.isEmpty() || username.length() < 6) { // 显示错误消息 } // 计算购物车总价 double totalPrice = 0.0; for (const QJsonObject& item : items) { totalPrice += item["price"].toDouble(); }
Network communication:
- HTTP request and response processing with cloud services.
- Send and receive JSON, XML or other data formats.
- Handling errors and timeouts.
// 向云端发送登录请求 QNetworkAccessManager* networkManager = new QNetworkAccessManager(); QNetworkRequest request("https://example.com/login"); request.setHeader(QNetworkRequest::ContentTypeHeader, "application/json"); // 准备 JSON 请求体 QJsonObject json; json["username"] = username; json["password"] = password; QByteArray postData = QJsonDocument(json).toJson(); // 发送请求 QNetworkReply* reply = networkManager.post(request, postData);
Multi-Threading and Concurrency:
- Create and manage multiple threads to perform background tasks such as data loading and processing.
- Use the signal and slot mechanism to implement communication between threads.
// 创建一个后台线程 QThread* thread = new QThread(); // 创建一个任务 QObject* task = new Task(); task->moveToThread(thread); // 将信号槽连接到主线程 QObject::connect(task, SIGNAL(progressUpdated(int)), this, SLOT(onProgressUpdated(int))); // 启动线程 thread->start();
By using C functions, developers can break down the complex logic of cloud-based GUI applications into manageable components. This improves code reusability, maintainability, and scalability, resulting in more powerful and reliable applications.
The above is the detailed content of What is the role of C++ functions in cloud-based GUI applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


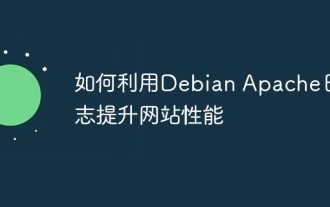
This article will explain how to improve website performance by analyzing Apache logs under the Debian system. 1. Log Analysis Basics Apache log records the detailed information of all HTTP requests, including IP address, timestamp, request URL, HTTP method and response code. In Debian systems, these logs are usually located in the /var/log/apache2/access.log and /var/log/apache2/error.log directories. Understanding the log structure is the first step in effective analysis. 2. Log analysis tool You can use a variety of tools to analyze Apache logs: Command line tools: grep, awk, sed and other command line tools.

This article describes how to customize Apache's log format on Debian systems. The following steps will guide you through the configuration process: Step 1: Access the Apache configuration file The main Apache configuration file of the Debian system is usually located in /etc/apache2/apache2.conf or /etc/apache2/httpd.conf. Open the configuration file with root permissions using the following command: sudonano/etc/apache2/apache2.conf or sudonano/etc/apache2/httpd.conf Step 2: Define custom log formats to find or
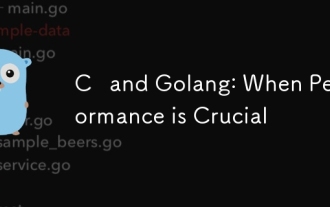
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
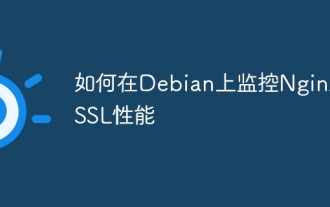
This article describes how to effectively monitor the SSL performance of Nginx servers on Debian systems. We will use NginxExporter to export Nginx status data to Prometheus and then visually display it through Grafana. Step 1: Configuring Nginx First, we need to enable the stub_status module in the Nginx configuration file to obtain the status information of Nginx. Add the following snippet in your Nginx configuration file (usually located in /etc/nginx/nginx.conf or its include file): location/nginx_status{stub_status
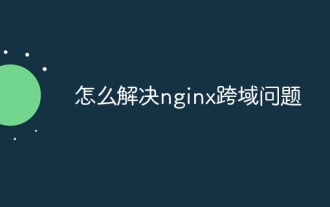
There are two ways to solve the Nginx cross-domain problem: modify the cross-domain response header: add directives to allow cross-domain requests, specify allowed methods and headers, and set cache time. Use CORS modules: Enable modules and configure CORS rules that allow cross-domain requests, methods, headers, and cache times.
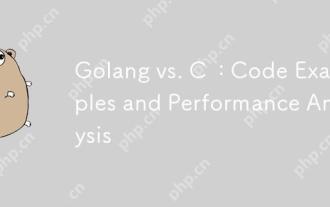
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.

The methods to view the running status of Nginx are: use the ps command to view the process status; view the Nginx configuration file /etc/nginx/nginx.conf; use the Nginx status module to enable the status endpoint; use monitoring tools such as Prometheus, Zabbix, or Nagios.
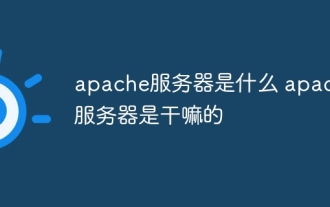
Apache server is a powerful web server software that acts as a bridge between browsers and website servers. 1. It handles HTTP requests and returns web page content based on requests; 2. Modular design allows extended functions, such as support for SSL encryption and dynamic web pages; 3. Configuration files (such as virtual host configurations) need to be carefully set to avoid security vulnerabilities, and optimize performance parameters, such as thread count and timeout time, in order to build high-performance and secure web applications.
