


Detailed explanation of C++ function parameters: the essence and precautions of the outgoing mechanism
C There are two ways to pass function parameters: call by value (does not affect actual parameters) and call by reference (affects actual parameters). Passing out parameters is achieved by passing a reference or pointer, and the function can pass the value to the caller by modifying the variable pointed to by the parameter reference or pointer. Please note when using: The outgoing parameters must be clearly declared, can only correspond to one actual parameter, and cannot point to local variables within the function. When calling by passing a pointer, be careful to avoid wild pointers.
Detailed explanation of function parameters in C: The essence and precautions of the outgoing mechanism
The essence of the outgoing mechanism
The function parameters in C There are two main transfer methods: Call by value and Call by reference.
- Call by value: Pass a copy of the actual parameters to the function parameters. Modifications to the parameters within the function will not affect the actual parameters.
- Call by reference: Pass a reference of the actual parameter to the function parameter. Modifications to the parameter within the function will directly affect the actual parameter.
Implementation of outgoing parameters
Outgoing parameters refers to the function passing the value to the caller through parameters. In a pass-by-reference call, the function can be passed out by modifying the variable pointed to by the parameter reference.
In C, you can use the reference (&) symbol or the pointer (*) symbol to implement outgoing parameters:
// 传引用调用 void Swap(int &a, int &b) { int temp = a; a = b; b = temp; } // 传指针调用 void Increment(int *ptr) { (*ptr)++; }
Actual case
Calling by reference
int main() { int x = 1, y = 2; Swap(x, y); // 传引用调用 // x 和 y 的值已经交换 cout << "x: " << x << ", y: " << y << endl; return 0; }
Calling by pointer
int main() { int n = 5; // 获取 n 的地址 int *ptr = &n; // 通过指针修改 n 的值 Increment(ptr); // n 的值已增加 1 cout << "n: " << n << endl; return 0; }
Notes
- Outgoing parameters must be explicitly declared. In function declarations and definitions, outgoing parameters need to be declared using reference or pointer notation.
- The outgoing parameter can only correspond to one actual parameter. If a function has multiple outgoing parameters, each parameter must correspond to a separate actual parameter.
- Outgoing parameters cannot point to local variables within the function. The life cycle of local variables is the same as that of the function. Once the function ends, the local variable will be destroyed and the reference or pointer pointing to it will become invalid.
- Use pointer-passing calls with caution. Pointers are prone to pointing to wild pointers, so you need to ensure that the pointer always points to a valid address.
The above is the detailed content of Detailed explanation of C++ function parameters: the essence and precautions of the outgoing mechanism. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


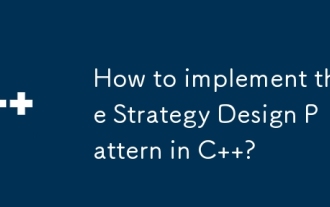
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
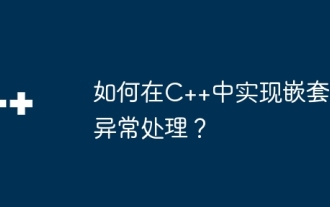
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
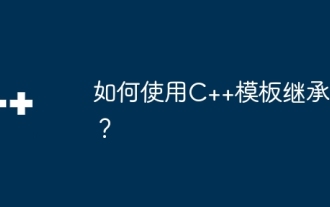
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
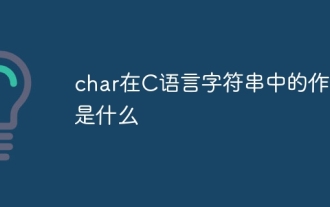
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
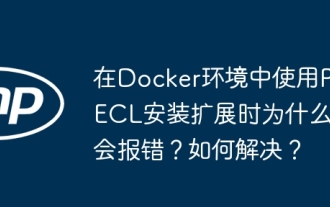
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
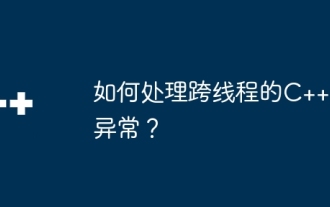
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
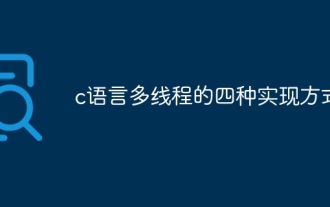
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
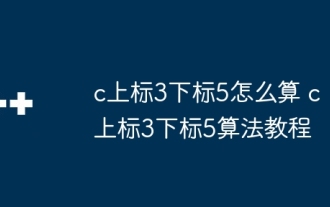
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
