


Detailed explanation of C++ function parameters: best practices in function signature design
Detailed explanation of C function parameters: Best practices in function signature design
In C, function signature defines the name, return type and parameter list. Well-designed function signatures are critical to writing readable, maintainable, and reusable code.
Parameter passing mode
C supports four parameter passing modes:
- Call by value:Copy the value of the parameter into the function.
- Call by reference: Pass the reference of the parameter to the function, and the modified value will be reflected in the caller.
- Call by pointer: Pass the parameter pointer to the function, and the modified value will be reflected in the caller.
- Call by rvalue reference: Allows functions to move or bind to rvalue (rvalue) parameters.
Best Practice
- Prefer using value-passing: For small objects or basic types that do not need to be modified, this It is an efficient and safe delivery method.
- For large or complex objects, use pass-by-reference or pointer: To avoid the overhead of copying, especially for objects that may need to be modified.
- Use const Quote For input parameters: Indicates that the function will not modify the value of the parameter, enhancing code security.
- For output parameters, use a pointer or pass an rvalue reference: Allows the function to modify external variables or move rvalue parameters.
- Limit the number of parameters: Excessive parameters will make the function signature difficult to understand and maintain.
- Use meaningful parameter names: They should clearly indicate the purpose of the parameter.
Practical case
Let us consider a function that calculates the sum of two numbers:
int sum(int a, int b); // 传值
If we want the function to modify the first number, you need to use pass-by-reference:
void add(int &a, int b); // 传引用
If we know that the first parameter will not be modified inside the function, we can use const reference to improve security:
int sum(const int &a, int b); // 传 const 引用
Note :
- Passing pointers and passing references are very similar in syntax, but different in semantics.
- Passing rvalue references only works in C 11 and later.
- The correct use of parameter passing mode can greatly improve code efficiency and security.
The above is the detailed content of Detailed explanation of C++ function parameters: best practices in function signature design. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
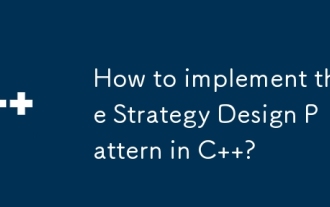
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
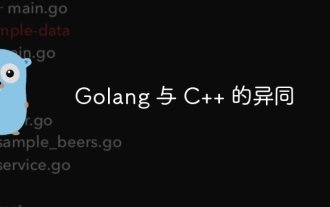
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
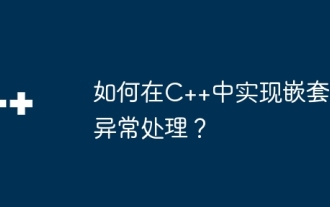
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
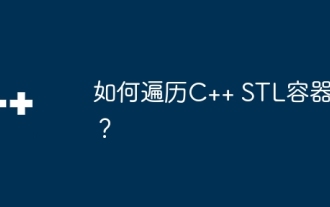
To iterate over an STL container, you can use the container's begin() and end() functions to get the iterator range: Vector: Use a for loop to iterate over the iterator range. Linked list: Use the next() member function to traverse the elements of the linked list. Mapping: Get the key-value iterator and use a for loop to traverse it.
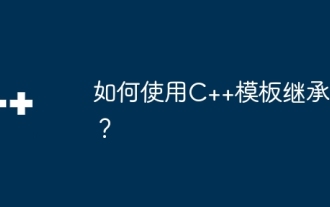
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
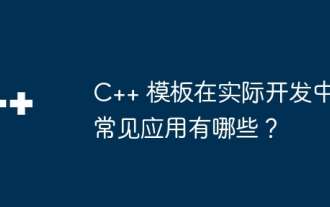
C++ templates are widely used in actual development, including container class templates, algorithm templates, generic function templates and metaprogramming templates. For example, a generic sorting algorithm can sort arrays of different types of data.
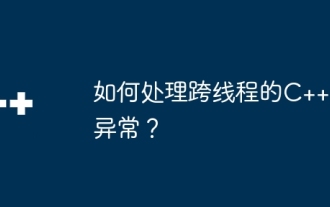
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
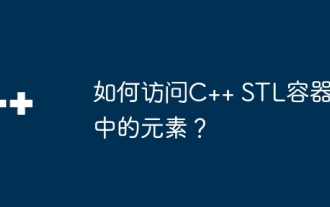
How to access elements in C++ STL container? There are several ways to do this: Traverse a container: Use an iterator Range-based for loop to access specific elements: Use an index (subscript operator []) Use a key (std::map or std::unordered_map)
